How can I get the full/absolute URL (with domain) in Django?
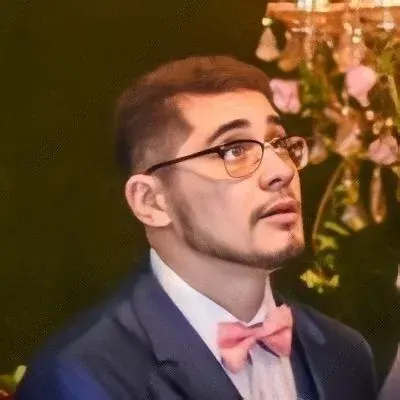
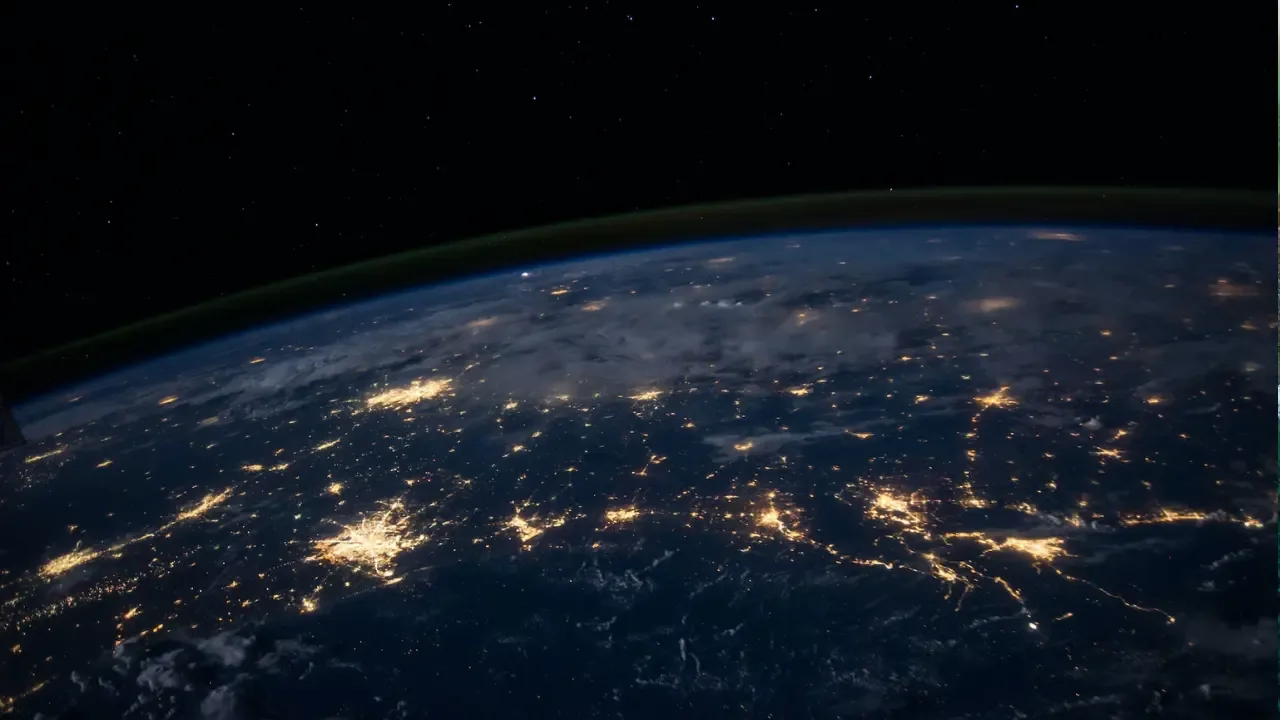
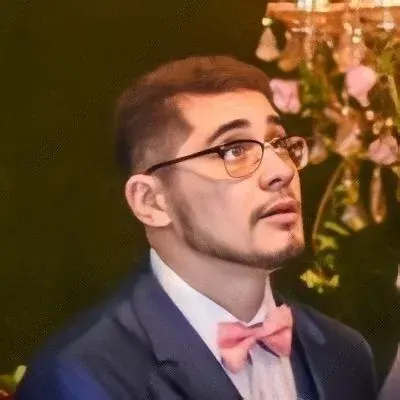
Getting the Absolute URL in Django: No Sites Module Required! 😎💻
So, you're building an awesome Django web app and you want to get the full/absolute URL to use it with the reverse()
function. You've stumbled upon a question on Stack Overflow where someone suggests using the Sites module, but you're thinking, "That's just silly! 🙄 I shouldn't need to query my DB to snag the URL!"
Fear not! In this blog post, I'll show you a simple solution to obtain the full/absolute URL without the Sites module. And the best part? No database queries required! Let's dive in! 🏊♂️
🚀 The Problem
Before we jump into the solution, let's make sure we understand the problem. You want to get the full/absolute URL, including the domain, for a specific path in your Django app. This URL will be used with the reverse()
function, which is great for generating URLs based on view names or URL patterns.
💡 The Solution
To get the full/absolute URL, we'll take advantage of Django's built-in request
object. This object contains information about the incoming HTTP request, including the domain.
Here's how you can achieve this:
from django.urls import reverse
from django.http import HttpRequest
def get_absolute_url(request: HttpRequest, path: str) -> str:
return request.build_absolute_uri(reverse(path))
In the code snippet above, we define a function called get_absolute_url
that takes the request
object and the path
you want to generate the URL for. We use request.build_absolute_uri()
to build the full/absolute URL and then combine it with the relative path using reverse()
.
🎉 Example Usage
Let's say you have a view called my_view
that maps to the URL pattern my-url/
. Here's how you can use our get_absolute_url
function:
def my_view(request):
absolute_url = get_absolute_url(request, 'my_view')
# do something awesome with the absolute_url
In the example above, absolute_url
will contain the full/absolute URL for the my_view
view.
📣 Call-to-Action: Share Your Thoughts and Experiences!
And there you have it! You now know how to get the full/absolute URL in Django without the Sites module. Pretty neat, right? 😄
I hope this blog post has been helpful to you on your Django journey. If you've faced similar challenges or have any tips to share, please leave a comment below. Let's learn from each other and build amazing Django apps together! 🎉🚀