Getting Django admin url for an object
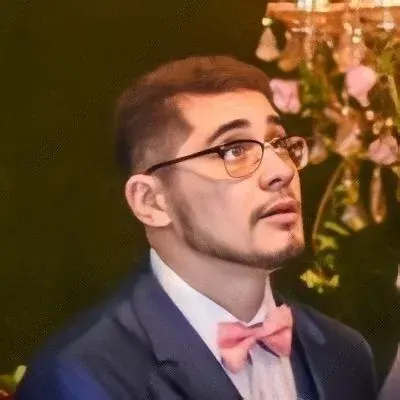
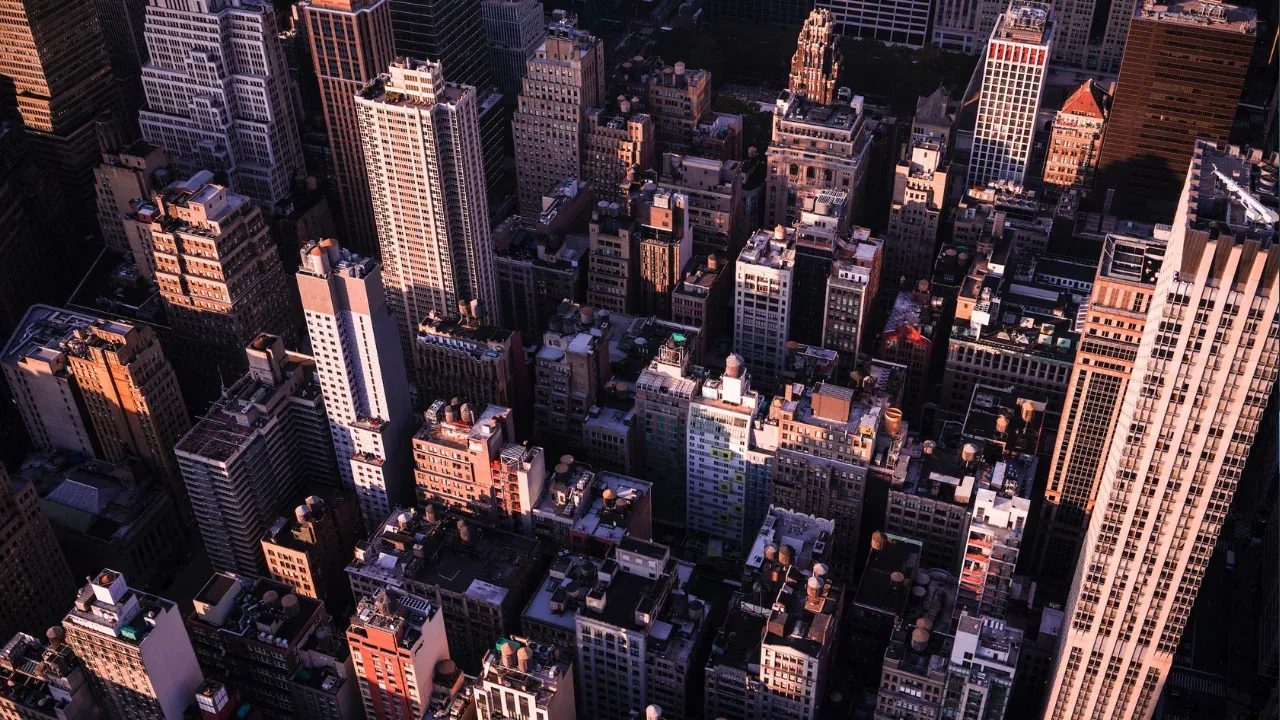
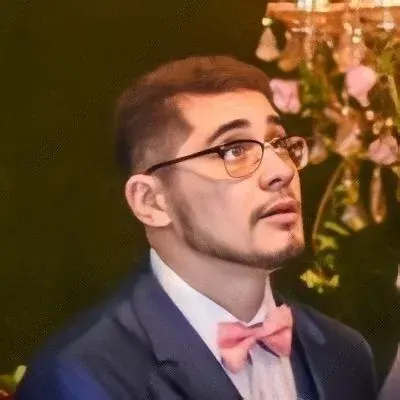
Getting the Django Admin URL for an Object 🌐
If you're a Django developer like me, you might have encountered a common issue when trying to get the admin URL for an object. In the past, it was a piece of cake, but with the latest versions of Django, a simple filter won't do the trick anymore. 😕
But fear not! I'm here to guide you through this and provide you with easy solutions. Let's dive in and find out how you can get that elusive admin URL!
The Old Approach 🕒
Before Django 1.0, you could easily obtain the admin URL of an object using a neat filter. Here's an example:
<a href="{{ object|admin_url }}">Click me!</a>
Behind the scenes, this filter utilized the url reverse
function with the view name django.contrib.admin.views.main.change_stage
. It even allowed you to pass additional arguments like app_label
, model_name
, and object_id
. But alas, this method is no longer effective in the latest Django versions. 😞
The Latest Approach ✨
To adapt to the changes in Django 1.0 and above, you'll need to take a different approach. Let's see how we can do this!
First, we'll import the necessary module:
from django.urls import reverse
Next, we can use the reverse
function and provide the appropriate view name, along with any required arguments. In this case, we'll be using the change
view:
admin_url = reverse('admin:APP_LABEL_MODEL_NAME_change', args=(object_id,))
Make sure to replace APP_LABEL
and MODEL_NAME
with the actual app label and model name of your object, and object_id
with the ID of the object you want to get the admin URL for.
A Handy Example 🌟
To make things clearer, let's look at a practical example. Suppose we have a Book
model within our Library
app. We want to retrieve the admin URL for a particular book with an ID of 42.
Here's how our code would look like:
from django.urls import reverse
app_label = 'library'
model_name = 'book'
object_id = 42
admin_url = reverse('admin:library_book_change', args=(object_id,))
With this, the admin_url
variable will hold the URL you need. How cool is that? 😎
Engage with the Community! 💬
Now that you have a solid grip on how to retrieve the admin URL for an object, why not engage with fellow Django enthusiasts? Share your thoughts, ask questions, or provide valuable insights! Let's all grow together as a community. 🌱
Feel free to leave a comment below and let me know if this guide was helpful to you. Have you encountered any other challenges while working with Django? I'd love to hear about it!
Happy coding! 💻🐍