Django REST Framework: adding additional field to ModelSerializer
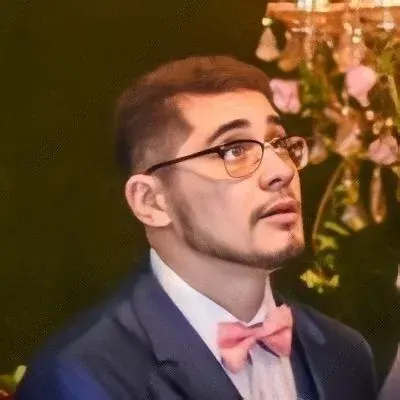
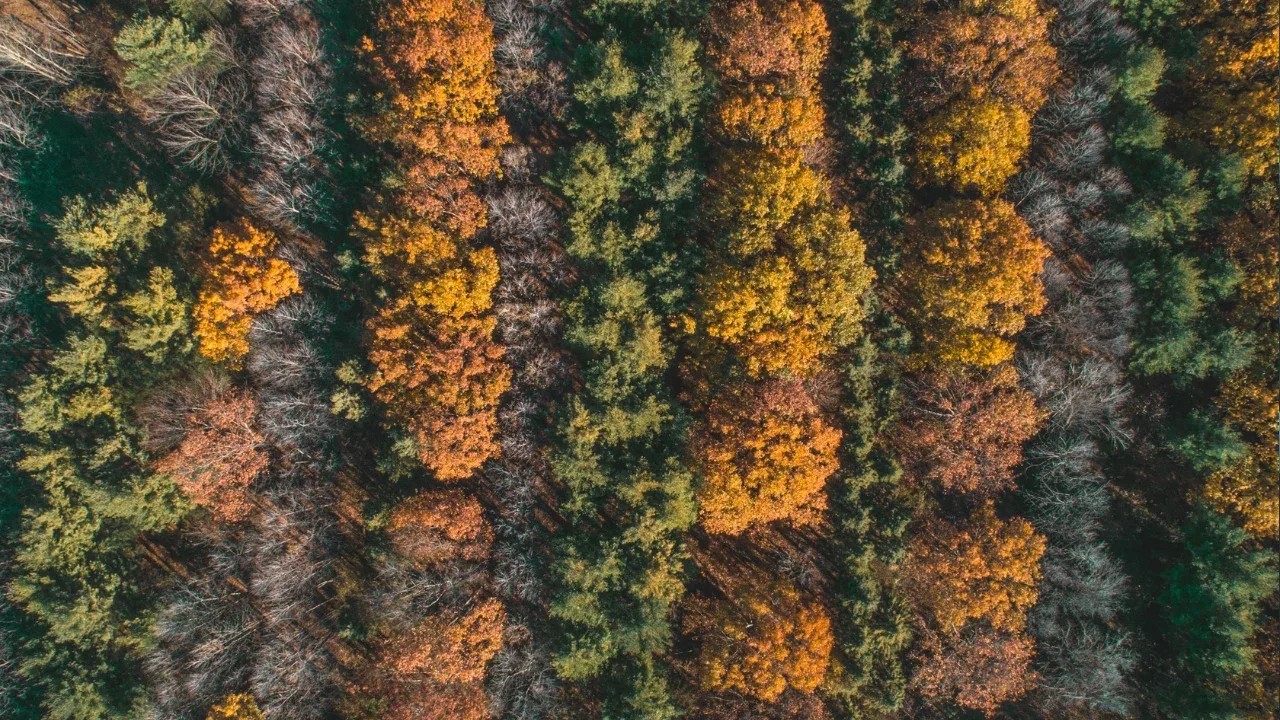
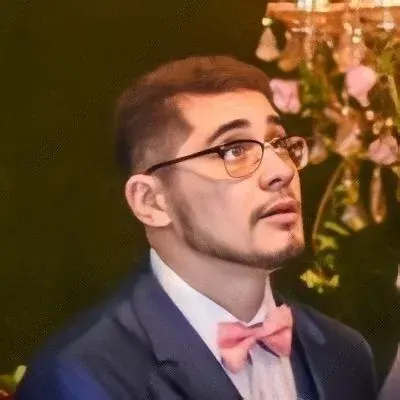
Django REST Framework: Adding Additional Field to ModelSerializer
So, you're working with Django REST Framework and want to add an additional field to your ModelSerializer. You've come to the right place! 🙌
The Problem
Let's take a look at the code snippet you provided:
class FooSerializer(serializers.ModelSerializer):
my_field = ... # result of some database queries on the input Foo object
class Meta:
model = Foo
fields = ('id', 'name', 'myfield')
Your goal is to include an extra field (my_field
) that requires performing some database lookups on the Foo
object being serialized. But how can you achieve this?
The Solution
Option 1: Using Extra Context
You mentioned that you came across the option to pass in extra "context" to the serializer. This can indeed be a viable solution. Instead of defining my_field
directly in the serializer, you can pass it as part of the context:
class FooSerializer(serializers.ModelSerializer):
class Meta:
model = Foo
fields = ('id', 'name', 'my_field')
def to_representation(self, instance):
data = super().to_representation(instance)
my_field = ... # Perform the necessary database queries here
data['my_field'] = my_field
return data
In the to_representation
method, you can access the serialized data through self.instance
and perform the required database queries to obtain the value for my_field
. Then, simply update the serialized data with the computed value.
Option 2: Using a Callable
Another approach is to define a function in the Foo
model that returns the value of my_field
and connect it to the serializer.
class Foo(models.Model):
name = models.CharField(max_length=100)
def get_my_field(self):
# Perform the necessary database queries here
my_field = ...
return my_field
class FooSerializer(serializers.ModelSerializer):
my_field = serializers.SerializerMethodField()
class Meta:
model = Foo
fields = ('id', 'name', 'my_field')
def get_my_field(self, instance):
return instance.get_my_field()
By using the SerializerMethodField
, you can call the get_my_field
method defined in the Foo
model to retrieve the value of my_field
.
Conclusion
With these two options, you can easily add an additional field to your ModelSerializer in Django REST Framework. Experiment with both approaches and choose the one that suits your needs best.
I hope this guide has helped you overcome any challenges you were facing. If you have further questions or need clarification, feel free to reach out. Happy coding! 😊
For more details, check out the Django REST Framework documentation on including extra context and specifying fields explicitly.