Django: Get list of model fields?
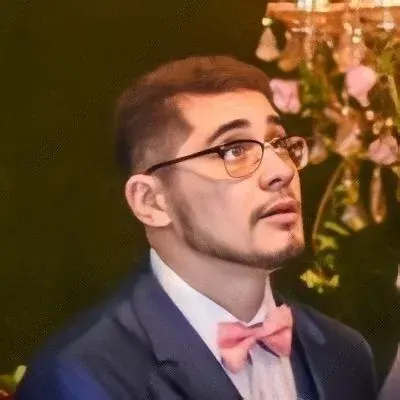
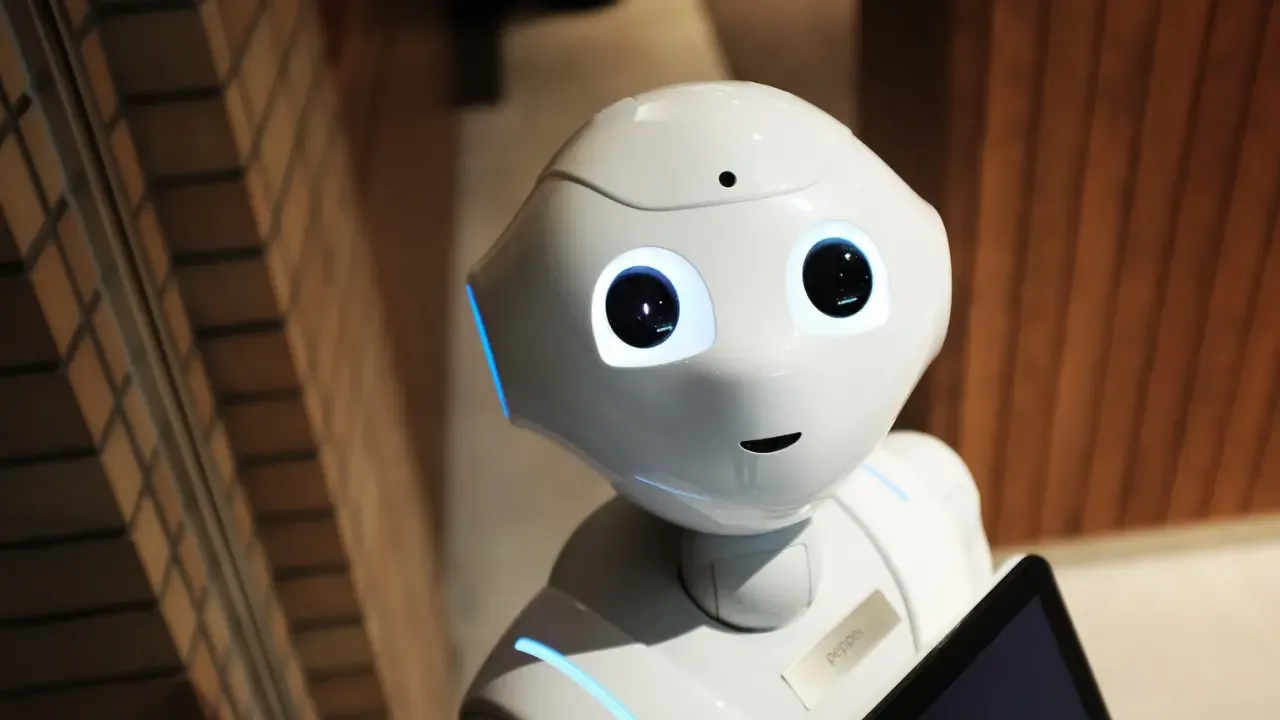
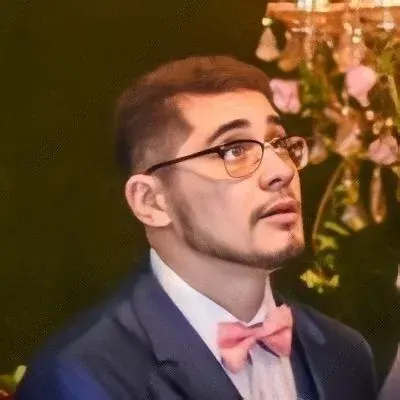
Django: Get list of model fields 📋
So you're working with Django and you want to retrieve a list of all the fields defined for a model. We've all been there! Don't worry, I've got you covered. In this blog post, I'll walk you through the common issues you might encounter and provide easy solutions to get that list of model fields you're looking for. Let's dive right in! 💪
The Problem 😕
Here's a summary of the problem at hand: You have a User
class that inherits from models.Model
in Django, and you want to retrieve a list of all the fields defined for this model. All you really care about is anything that inherits from the Field
class.
You might be tempted to use the inspect.getmembers(model)
function to get these fields, but unfortunately, it doesn't return the desired results. Django seems to have already processed the class, added its magic attributes, and stripped out the fields you're interested in.
The Solution 💡
So how can we get the list of model fields? Fortunately, Django provides us with a handy function called get_fields()
that we can use to retrieve these fields. Here's how you can do it:
from django.db import models
fields = [field for field in models.User._meta.get_fields() if isinstance(field, models.Field)]
Let's break this down:
We import the
models
module fromdjango.db
.We use the
_meta
attribute of theUser
model to access its metadata.We call the
get_fields()
function to retrieve all the fields defined for the model.We filter the fields to only include instances of the
Field
class.
And there you have it! The fields
list now contains all the model fields you were looking for. 🎉
An Example 🌟
To illustrate this further, let's consider an example where we have a User
model with a name
field and an email
field:
from django.db import models
class User(models.Model):
name = models.CharField(max_length=100)
email = models.EmailField(max_length=255)
Using the solution mentioned earlier, we can get the list of fields like this:
from django.db import models
fields = [field for field in models.User._meta.get_fields() if isinstance(field, models.Field)]
The resulting fields
list will contain the name
field and the email
field.
Engage with the Community 🤝
Now that you know the easy solution to retrieve the list of model fields in Django, why not share it with the community? Leave a comment down below and let me know if this solution worked for you. If you have any other tips or tricks, feel free to share them as well! Let's help each other become Django masters! 🚀
Remember, never stop learning, and happy coding! 💻🎓
References:
Django Documentation: Models | Django documentation
Stack Overflow: Django: Get list of model fields