Creating email templates with Django
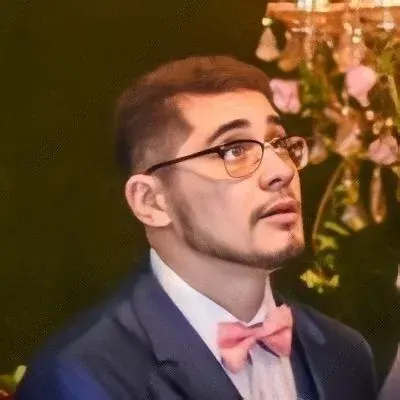
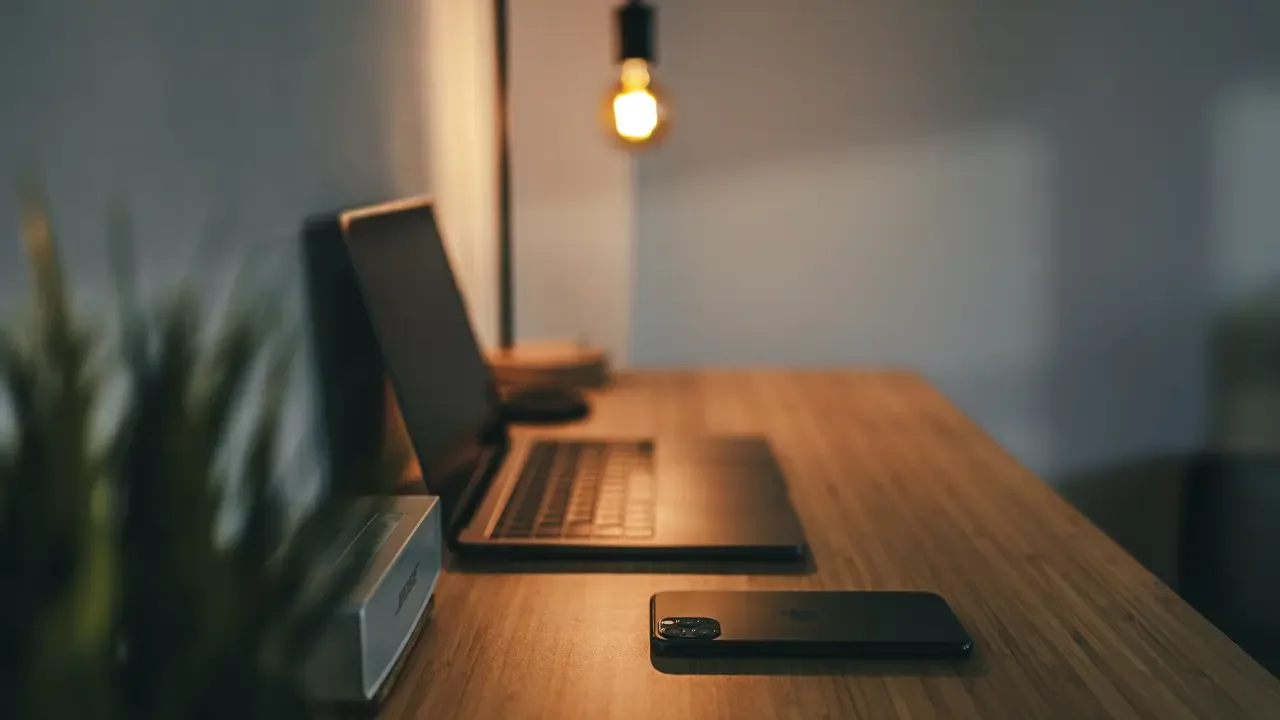
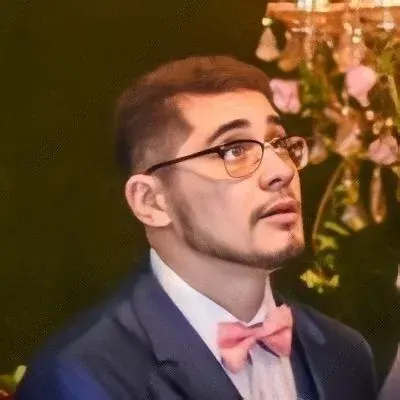
Creating Dynamic Email Templates with Django 📧💻
So, you want to send beautiful and dynamic HTML emails using Django templates? Look no further, because we've got you covered! 🚀 In this blog post, we'll address the common issue of using Django's template engine to generate email templates and provide easy solutions to help you achieve your goal effortlessly.
The Problem 😕
You may have come across the challenge of wanting to send emails with dynamic content, such as personalized greetings or incorporating user-specific data like usernames, while also maintaining the flexibility of Django's template system. The provided example shows a basic email template with a dynamic variable {{username}}
, but how do you actually generate and send such emails using Django?
Solution 1: Django's built-in send_mail
function 💡📧
Django provides a handy built-in function called send_mail
which allows you to send plain text emails. However, the challenge arises when you want to send HTML-formatted emails with dynamic content. But worry not, we have a solution for you!
Solution 2: Using Django's EmailMessage
class 💌📝
To overcome the limitations of plain text emails, Django offers the EmailMessage
class, which gives you more control over the email body. Let's see how to use it:
from django.core.mail import EmailMessage
def send_dynamic_email(recipient_email, username):
email = EmailMessage(
'Your Account Activation',
'Hello <strong>{}</strong>, your account has been activated.'.format(username),
'sender@example.com',
[recipient_email],
)
email.content_subtype = 'html'
email.send()
In this example, we're creating an instance of EmailMessage
and setting the subject, the HTML content with the dynamic username
variable, sender email address, and recipient email address. By setting email.content_subtype
to 'html'
, we are indicating that the email body contains HTML content.
Solution 3: Using Django's get_template
function 🎨📨
While the above solutions work perfectly fine, they lack the power and flexibility of Django's template system. If you want to leverage the full potential of Django's template engine, you can use the get_template
function from django.template.loader
module.
from django.core.mail import EmailMultiAlternatives
from django.template.loader import get_template
from django.template import Context
def send_dynamic_email(recipient_email, username):
subject = 'Your Account Activation'
text_template = get_template('email_template.txt')
html_template = get_template('email_template.html')
text_content = text_template.render(Context({'username': username}))
html_content = html_template.render(Context({'username': username}))
email = EmailMultiAlternatives(subject, text_content, 'sender@example.com', [recipient_email])
email.attach_alternative(html_content, 'text/html')
email.send()
In this approach, we have two separate template files: email_template.txt
(for plain text) and email_template.html
(for HTML content). We use the get_template
function to load these templates and then render them using the Context
object, passing the dynamic username
variable. Finally, we attach the HTML content to the email using email.attach_alternative
before sending it.
Wrapping Up 🎁💡
Congratulations! You're now equipped with multiple solutions to create engaging and dynamic email templates using Django. Whether you choose the simplicity of EmailMessage
or the flexibility of Django's template engine, sending beautiful and personalized emails is no longer a daunting task!
So, go ahead and level up your email game with these techniques. Start sending eye-catching, dynamic emails that will impress your users and enhance their experience with your application. 🚀✉️
Got any questions or other cool strategies for creating email templates with Django? Share them in the comments below! Let's help each other and make email communications a delightful experience for all! 💌💬