Checking for empty queryset in Django
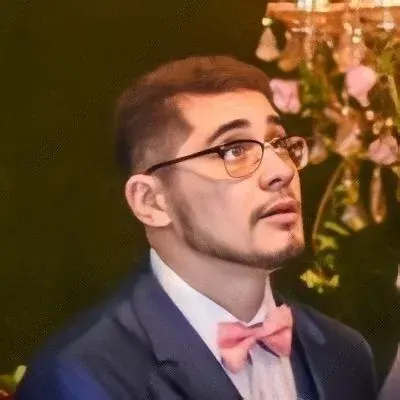
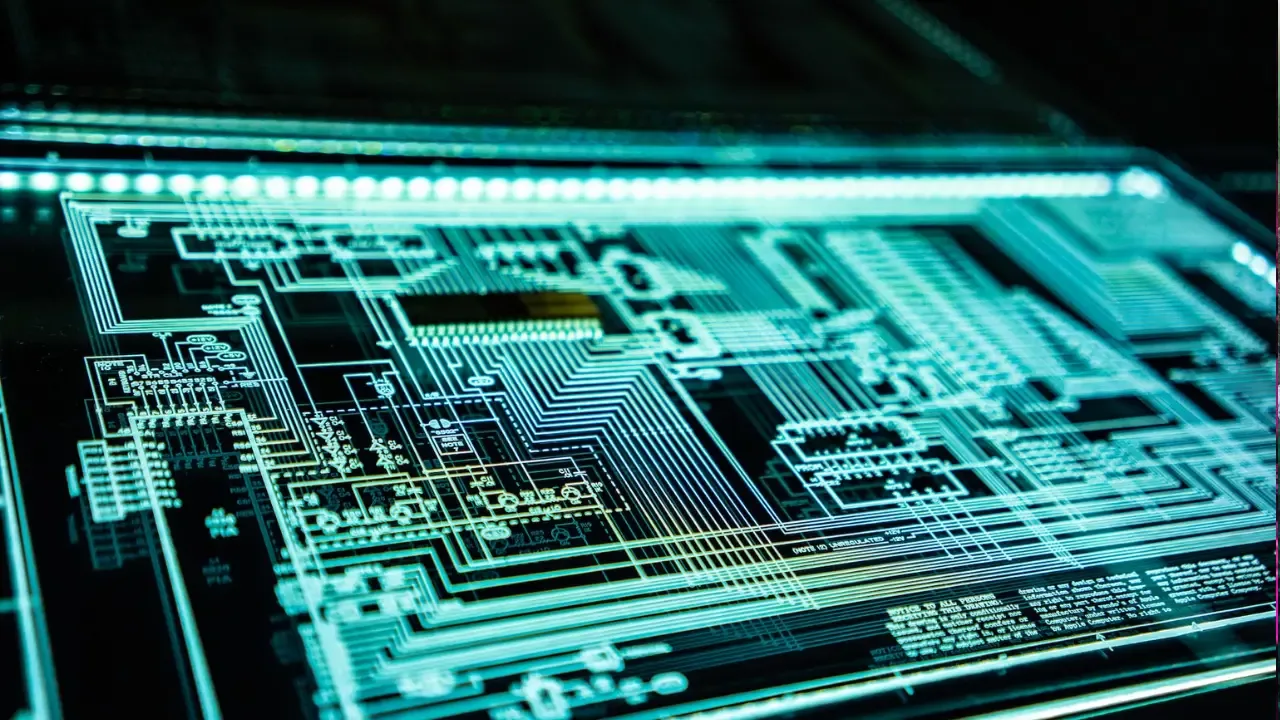
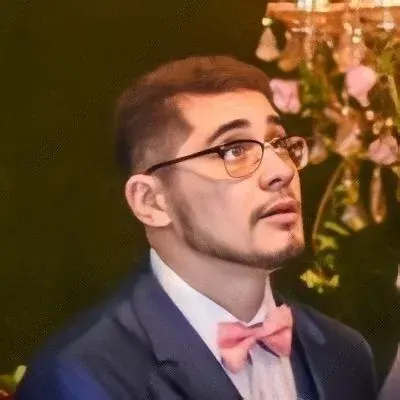
How to Check for an Empty QuerySet in Django
š Hey there, fellow Django developer! Have you ever found yourself in a situation where you needed to check if a query returned any results? š¤ Well, you're not alone! This is a common issue that many developers face, and in this blog post, I'm going to show you some easy solutions to tackle it. So, let's dive right in!
The Challenge
Let's consider the scenario where you have executed a query in Django to fetch some data from your database. Now, you want to perform different actions depending on whether the query returned any results or not. But how do you go about checking if the QuerySet is empty? š§
The Problem
The example you provided illustrates the struggle many developers face when trying to handle empty QuerySets in Django. The code snippet checks for a specific organization's name and is looking for a way to handle the case when no results are found. š¢
Easy Solutions
There are a few ways you can handle this situation, and I will walk you through each solution step-by-step. Choose the one that suits your needs best!
Solution 1: Using the exists()
method
One simple way to check if a QuerySet is empty is by using the exists()
method. Here's how you can do it:
orgs = Organisation.objects.filter(name__iexact='Fjuk inc')
if orgs.exists():
# Do this with the results without querying again.
else:
# Do something else...
By using the exists()
method, you can determine whether the QuerySet contains any results without hitting the database again. This approach is quick, efficient, and easy to understand.
Solution 2: Using the count()
method
Another way to check for an empty QuerySet is by using the count()
method. Here's an example:
orgs = Organisation.objects.filter(name__iexact='Fjuk inc')
if orgs.count() > 0:
# Do this with the results without querying again
else:
# Do something else...
In this solution, we use the count()
method to get the number of results in the QuerySet. If the count is greater than zero, we know that the QuerySet is not empty and can proceed accordingly.
Solution 3: Using Python's truthiness
Python has a concept called "truthiness," which means that objects can be evaluated as either true or false. When it comes to QuerySets, an empty QuerySet evaluates to False
, while a non-empty QuerySet evaluates to True
. Here's an example:
orgs = Organisation.objects.filter(name__iexact='Fjuk inc')
if orgs:
# Do this with the results without querying again
else:
# Do something else...
By using this approach, you can take advantage of Python's truthiness and write more concise code. However, keep in mind that this method might not be as explicit as the previous solutions.
Take Action and Improve Your Django Skills
Now that you have learned multiple ways to check for an empty QuerySet in Django, it's time to put your newfound knowledge into practice! š Experiment with these solutions and see which one works best for your specific use case.
Remember, understanding how to handle empty QuerySets is an essential skill for any Django developer. So keep practicing, stay curious, and never stop learning! š”
If you have any questions, comments, or other tips you'd like to share, feel free to leave a comment below. Let's keep the Django community engaged and thriving together! š¤
Happy coding! š»āØ