Can I access constants in settings.py from templates in Django?
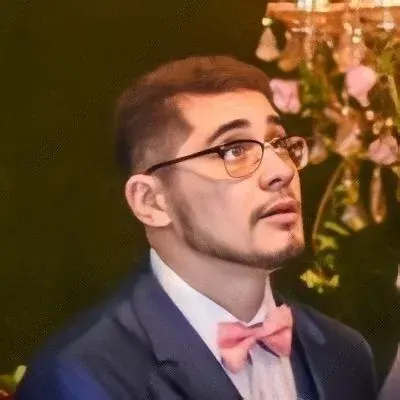
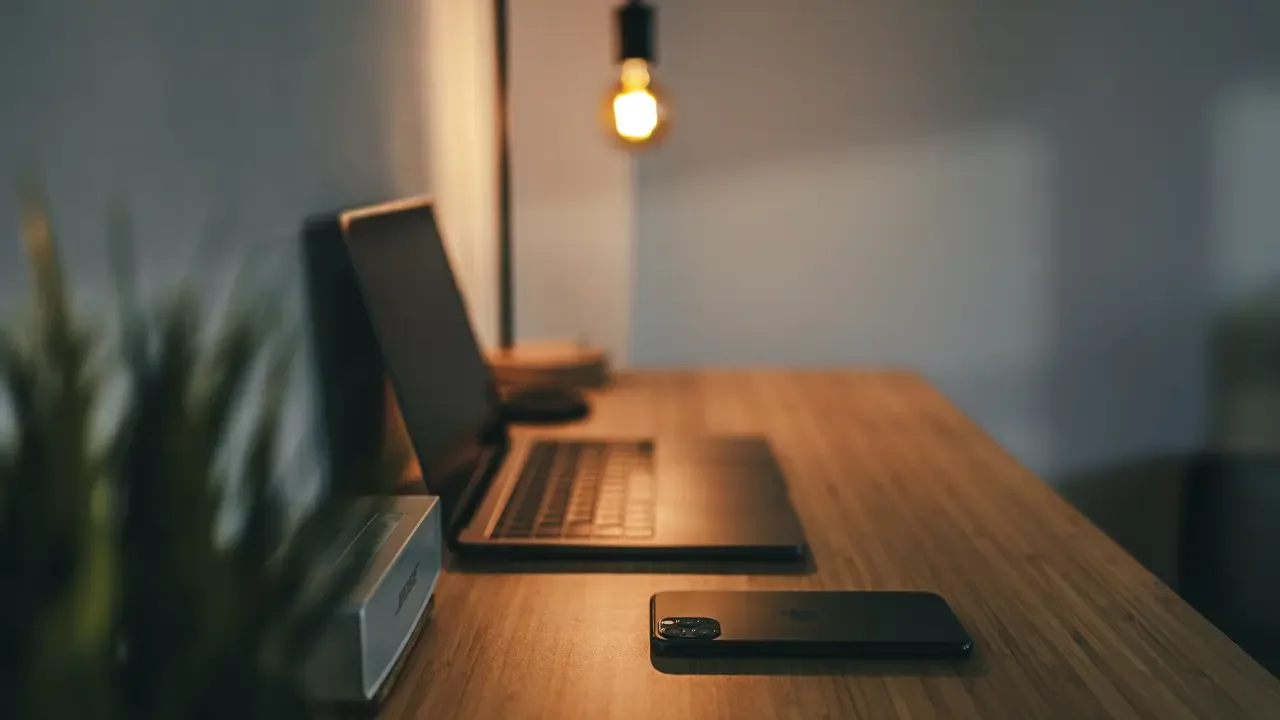
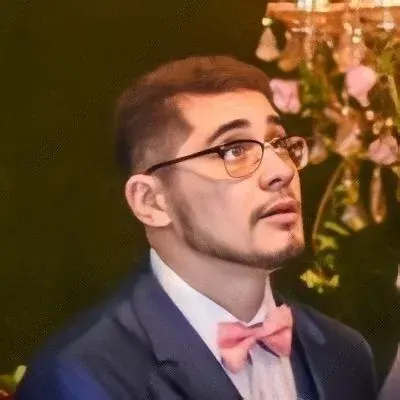
💡 Can I access constants in settings.py
from templates in Django?
If you've ever found yourself in a situation where you needed to access constants defined in your settings.py
file from your Django templates, you're not alone. This can be a common issue when trying to display dynamic content or configure your templates based on values stored in your project's settings. Fortunately, there are a few easy solutions that can help you achieve this.
The Problem
The issue at hand is that simply using {{ CONSTANT_NAME }}
in your template won't work, as template rendering only has access to the context variables passed to it. Django doesn't directly expose the settings module to the template engine, so we need to find an alternative approach. Let's dive into the solutions!
Solution 1: Using the context_processors
setting
The first solution involves using the context_processors
setting in your Django project. This setting defines a list of callables that takes a request
object and returns a dictionary of items to be merged into the template's context.
Create a new context processor file (e.g.,
myapp/context_processors.py
) and define a function that returns the desired constant as a dictionary. For example:
def constant(request):
from django.conf import settings
return {'MY_CONSTANT': settings.MY_CONSTANT}
Register the context processor in your project's settings module. Add
'myapp.context_processors.constant'
to theTEMPLATES
key underOPTIONS['context_processors']
.
Now, in your template, you can access the constant using {{ MY_CONSTANT }}
.
Solution 2: Using a custom template tag
Another approach is to create a custom template tag that directly retrieves the constant value from the settings.py
file.
Create a new file for your custom template tags (e.g.,
myapp/templatetags/my_tags.py
) and define a new class that inherits fromdjango.template.Library
. For example:
from django import template
from django.conf import settings
register = template.Library()
@register.simple_tag
def get_constant():
return settings.MY_CONSTANT
In your template, load your custom template tags using
{% load my_tags %}
and then use the custom tag to access the constant value:{% get_constant %}
.
Solution 3: Using a context processor decorator
If you prefer a more concise approach, you can use a decorator to simplify the process of defining and registering your context processor.
Install the
django-context-decorator
package:pip install django-context-decorator
.Import the
context_decorator
function into yourcontext_processors.py
module:from context_decorator import context
.Define your context processor function using the
@context
decorator:
from django.conf import settings
from context_decorator import context
@context(additional=True)
def constant(request):
return {'MY_CONSTANT': settings.MY_CONSTANT}
Register the context processor using the
context()
function in your project's settings module. Addcontext('myapp.context_processors.constant')
to theTEMPLATES
key underOPTIONS['context_processors']
.
Choose whichever solution best fits your project's needs and preferences, and you'll be able to access constants from your settings.py
file directly in your Django templates.
💬 Call-to-Action: Share your experiences!
Have you ever needed to access constants from your settings.py
file in Django templates? Which solution worked best for you? Share your experiences and any additional tips in the comments below. Let's help each other tackle Django's challenges together! 👇🎉