Iterating Through a Dictionary in Swift
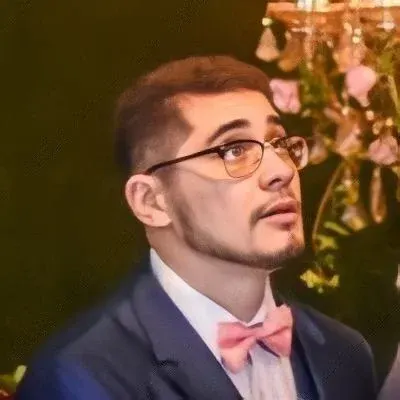

💡 Demystifying Dictionary Iteration in Swift
Hey tech enthusiasts! 👋 Let's dive into the fascinating world of iterating through dictionaries in Swift. 🚀 In this blog post, we'll demystify a common issue faced by developers and provide easy solutions to help you navigate through the dictionary jungle. 🌴
The Dilemma 🤔
One of our readers had a query about the behavior of their code while iterating through a dictionary. They were perplexed by the varying results obtained each time they ran their experiment. Let's take a closer look at their code snippet:
let interestingNumbers = [
"Prime": [2, 3, 5, 7, 11, 13],
"Fibonacci": [1, 1, 2, 3, 5, 8],
"Square": [1, 4, 9, 16, 25]
]
var largest = 0
for (kind, numbers) in interestingNumbers {
for number in numbers {
if number > largest {
largest = number
}
}
}
largest
Decoding the Code 🔍
The code snippet provided above aims to find the largest number among the arrays within the dictionary. The outer loop iterates through each key-value pair in the dictionary, where (kind, numbers)
represents the individual key-value pairs. The inner loop then iterates through the numbers within each key's corresponding array. Each number encountered is checked against the current value of largest
. If a larger number is found, it gets assigned to largest
.
Cracking the Mystery 🕵️♀️
Our reader was puzzled as they noticed different values for largest
every time they ran the code. Let's unravel the mystery behind this behavior! 🧩
The key to understanding this lies in the fact that the code snippet is designed to find the overall largest number across all arrays. The code doesn't account for individual largest numbers within each array.
The variable largest
is initially set to 0 before iterating through the dictionary. As the code traverses the dictionary, it updates largest
whenever it encounters a larger number in any of the arrays. However, it doesn't retain the value of the largest number in each individual array. It's important to remember that largest
represents the overall largest number found so far, not the largest number in each specific array.
Solution to the Confusion 💡
To align expectations with the desired behavior, we can modify the code slightly to find the largest number for each array within the dictionary. Here's how we can achieve this:
let interestingNumbers = [
"Prime": [2, 3, 5, 7, 11, 13],
"Fibonacci": [1, 1, 2, 3, 5, 8],
"Square": [1, 4, 9, 16, 25]
]
var largestNumbers: [String: Int] = [:]
for (kind, numbers) in interestingNumbers {
var largest = 0 // Create a new variable to store the largest number for each array
for number in numbers {
if number > largest {
largest = number
}
}
largestNumbers[kind] = largest // Store the largest number for each array in a new dictionary
}
largestNumbers
By introducing a new dictionary, largestNumbers
, we can now store the largest number for each respective array. This clarifies any ambiguity and provides a more accurate representation of the largest numbers within each key's array.
Let's Recap! 📚
Iterating through dictionaries in Swift involves using a
for-in
loop.Be mindful of the purpose of your code and the behavior it aims to achieve.
The original code snippet searches for the overall largest number across all arrays, not the largest number in each individual array.
If you need the largest number for each array within the dictionary, consider using a separate dictionary to store the largest numbers specifically.
Stay Curious, Stay Empowered! 💪
Now that you understand how to iterate through dictionaries in Swift with precision, you're ready to tackle any dictionary-related challenge that comes your way! 🎉
Remember, the journey of a developer involves continuous learning and exploring new technologies. If you found this blog post insightful, share it with your friends and colleagues who might find it helpful. Let's spread the knowledge! 🌟
Keep coding, keep inspiring! 🚀
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
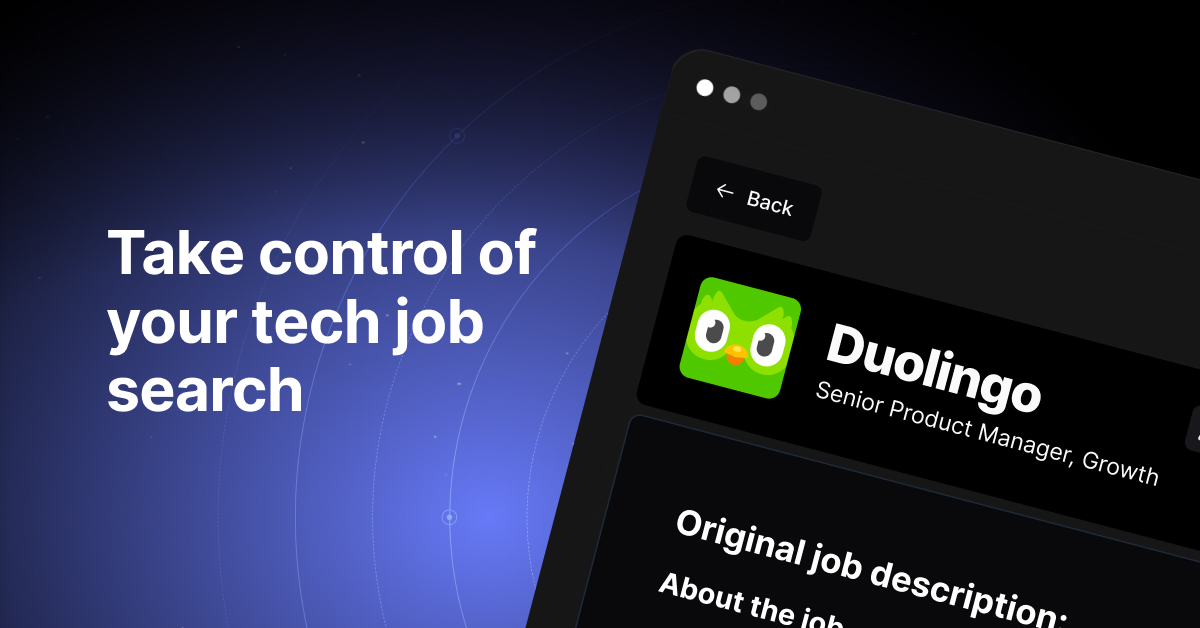