Declare and initialize a Dictionary in Typescript
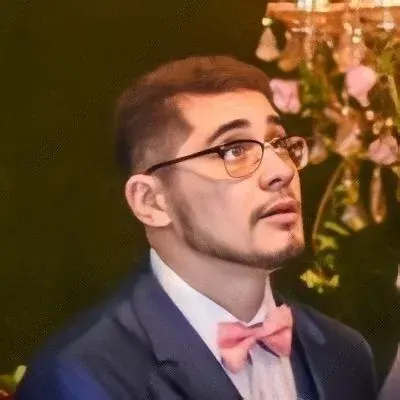
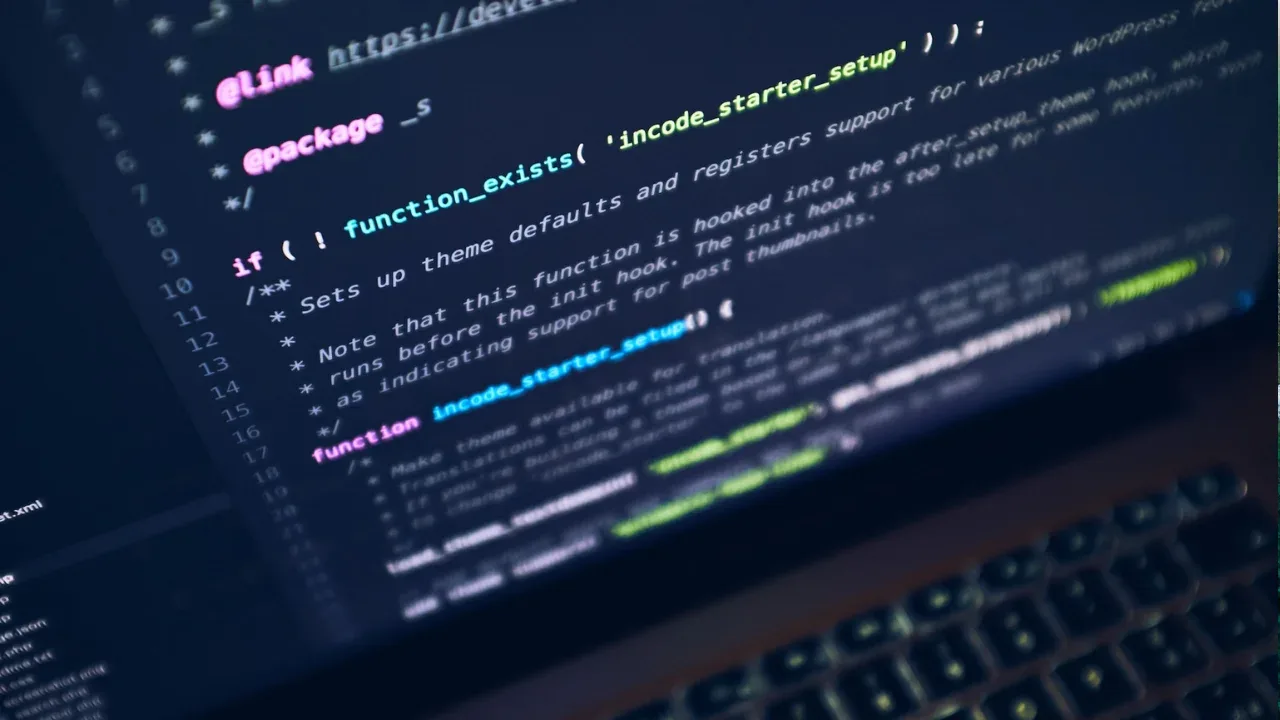
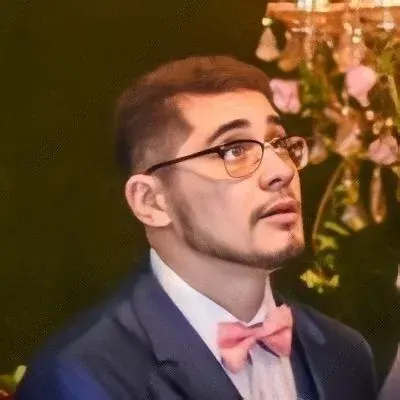
📖 Mastering TypeScript: Declaring and Initializing Dictionaries
Do you find yourself scratching your head when it comes to declaring and initializing dictionaries in TypeScript? 🤔 Don't worry, you're not alone! Many developers, especially those new to TypeScript, struggle with this concept. But fear not! In this blog post, we'll demystify the process and provide you with easy solutions to common issues. Let's dive in! 💪🏼
The Problem
Consider the following code snippet:
interface IPerson {
firstName: string;
lastName: string;
}
var persons: { [id: string]: IPerson; } = {
"p1": { firstName: "F1", lastName: "L1" },
"p2": { firstName: "F2" }
};
Now, let's focus on the second object {"p2": { firstName: "F2" }}
. As you can see, it doesn't have the lastName
property. So, why isn't the initialization rejected? 🤷🏻♂️
The Explanation
The reason behind this behavior lies in TypeScript's type system. By explicitly specifying the type of the persons
variable as { [id: string]: IPerson; }
, we are essentially telling TypeScript that it is a dictionary (also known as an index signature) with string
keys and IPerson
values. This type declaration allows for flexible assignments.
In other words, when initializing a dictionary, TypeScript only enforces that the assigned values adhere to the specified value type (IPerson
in this case). The keys in the dictionary are not checked for correctness during initialization.
Easy Solutions
Solution 1: Declare a Partial IPerson Type
If you want to ensure that all properties of IPerson
are required during initialization, one solution is to declare a "partial" version of the IPerson
type. This partial type will mark all properties as optional using the Partial<T>
utility type. Here's how you can do it:
interface IPersonPartial {
firstName?: string; // Making properties optional using '?'
lastName?: string;
}
var persons: { [id: string]: IPersonPartial; } = {
"p1": { firstName: "F1", lastName: "L1" },
"p2": { firstName: "F2" }
};
Now, TypeScript will complain if you try to assign an incomplete object to the persons
dictionary.
Solution 2: Use Type Assertions
Another way to tackle this issue is by using type assertions. Type assertions allow you to override TypeScript's inferred type and explicitly specify the intended type. You can achieve this by using the as
keyword. Here's an example:
var persons: { [id: string]: IPerson; } = {
"p1": { firstName: "F1", lastName: "L1" },
"p2": { firstName: "F2" } as IPerson // Type assertion to explicitly specify the type
};
By asserting the type of {"p2": { firstName: "F2" }}
as IPerson
, you're essentially telling TypeScript that you know what you're doing and that the missing lastName
property is intentional.
Your Turn! 💡
Now that you've learned how to declare and initialize dictionaries in TypeScript, it's time to put your knowledge into practice! Head over to your favorite TypeScript project and try these solutions out. If you come across any hurdles or have any questions, feel free to leave a comment below. We're always here to help!
So what are you waiting for? Start playing around with dictionaries in TypeScript and unlock a whole new world of possibilities! 🔓✨
📢 Let's Connect! Are you hungry for more TypeScript knowledge? Make sure to subscribe to our newsletter for regular updates on TypeScript tips, tricks, and best practices. Also, follow us on Twitter and join our vibrant community of TypeScript enthusiasts. We can't wait to see what you build with TypeScript! 🚀🔥
🗣️ Share the Love If you found this blog post helpful, share it with your developer friends! Let's spread the TypeScript love and empower more developers in our community. Together, we can make TypeScript the go-to language for web development! ❤️
Happy coding! 💻✨
Disclaimer: The sample code provided is for educational purposes only and may not reflect best practices in a production environment. Always review and test the code thoroughly before applying it to your own projects.