How to get the current time as datetime
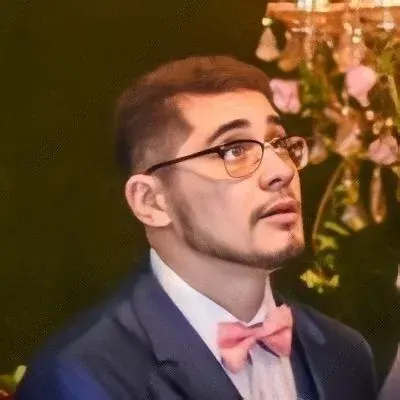
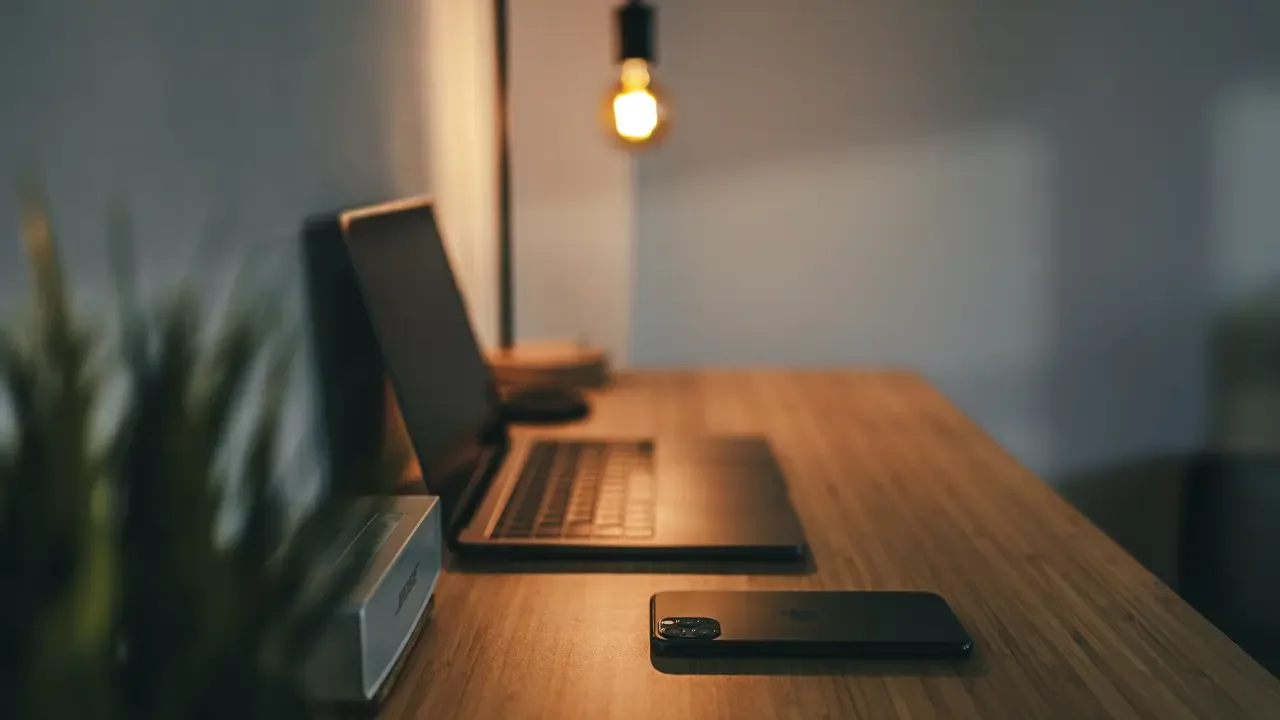
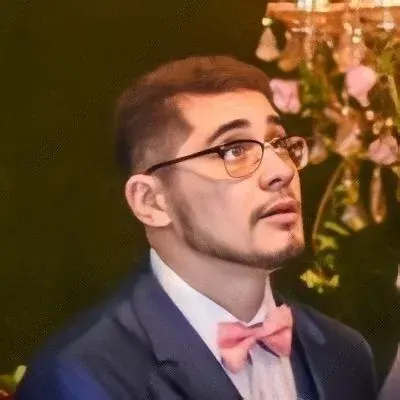
How to Get the Current Time as Datetime in Swift 🕰️
Are you a beginner in app development and struggling to get the current hour in Swift? Don't worry, we've got you covered! In this guide, we'll walk you through the process of obtaining the current time as a datetime object in Swift, addressing common issues and providing easy solutions.
The Challenge 🤔
Here's the context: you've just started working with Swift and want to create a simple app. You've successfully created a date object, but now you're stuck trying to extract the current hour. In other programming languages, you might be familiar with accessing the hour directly from the date object, but it seems different in Swift.
The Solution 💡
Not to worry, Swift provides a straightforward solution for obtaining the current time as a datetime object. Instead of directly accessing the hour from the date object, we can utilize the Calendar
and DateComponents
classes to extract the desired information.
Here's an example of how you can get the current hour in Swift:
import Foundation
let currentDate = Date()
let calendar = Calendar.current
let hour = calendar.component(.hour, from: currentDate)
print(hour) // Output: the current hour in 24-hour format
In the code snippet above, we first create a Date
object using the Date()
initializer, which represents the current date and time. Next, we initialize the Calendar
object using the Calendar.current
property, which returns the current calendar for the user's locale. Finally, we use the component(_:from:)
method of the Calendar
class to extract the hour from the currentDate
.
Note: The component(_:from:)
method allows you to extract different components like hour, minute, second, etc. by specifying the desired component as a parameter.
The Alternative Approach 🔄
An alternative approach for getting the current hour is to use the DateFormatter
class's string(from:)
method. This method allows you to format the date into a string representation according to a desired format.
Here's an example of how you can achieve this:
import Foundation
let currentDate = Date()
let dateFormatter = DateFormatter()
dateFormatter.dateFormat = "HH" // "HH" represents the hour in 24-hour format
let hour = dateFormatter.string(from: currentDate)
print(hour) // Output: the current hour in 24-hour format as a string
In the code snippet above, we create a Date
object as before and initialize a DateFormatter
object. We then set the desired format using the dateFormat
property of the DateFormatter
class. Finally, we use the string(from:)
method to convert the currentDate
into a string representation of the hour.
Conclusion and Call-to-Action 🚀
By utilizing either the Calendar
and DateComponents
classes or the DateFormatter
class, you can easily obtain the current hour in Swift. We hope this guide has helped you overcome the challenge you faced and provided you with valuable insights into Swift's datetime manipulation.
Now take some time to experiment with these approaches in your own projects. If you encounter any issues or have any further questions, we'd love to hear from you in the comments below! 😊
So, what are you waiting for? Start coding and make the most of your newfound knowledge in Swift datetime manipulation! 🎉