How do I express a date type in TypeScript?
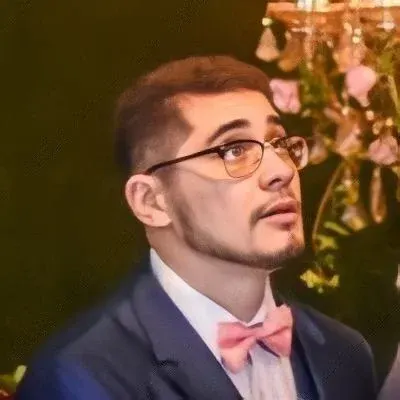
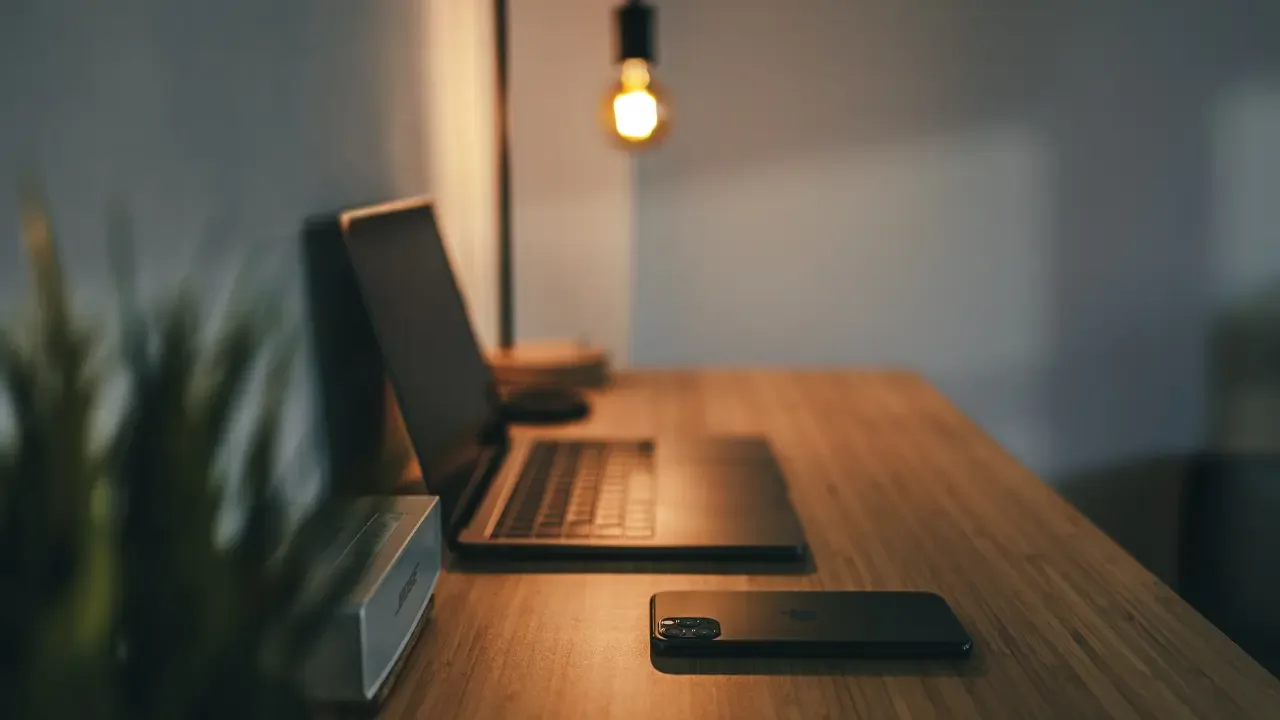
šš How to Express a Date Type in TypeScript
š Hey there! So, you're trying to figure out how to express dates in TypeScript? You're not alone! It's a common question and can sometimes be confusing. But don't worry, I've got your back. Let's dive right in and find the answer together!
š¤ The Issue:
So, you've stumbled upon the fact that dates aren't a built-in TypeScript type. Bummer! Now, you're left thinking if you should use the any
type or the object
type to represent dates in your code. But hey, there must be a better way, right? You're absolutely correct!
š” The Solution: Instead of resorting to generic types, TypeScript provides a solution for expressing date types in a more accurate and type-safe manner.
šµļøāāļø Type Libraries:
Fortunately, there are some widely used type libraries available that extend TypeScript's capabilities. One of the most popular ones is moment.js
. This library allows you to handle, manipulate, and format dates effortlessly.
š¦ Installing moment.js:
To start using moment.js
, make sure to install it in your project. Open your terminal, navigate to your project directory, and run the following command:
npm install moment
š Importing moment.js:
After installation, import moment.js
into your TypeScript file:
import * as moment from 'moment';
š
Expressing Dates:
Now, you can express dates using moment.js
's Moment
type. Here's an example:
let myDate: moment.Moment = moment();
console.log(myDate.format('YYYY-MM-DD')); // Output: 2022-01-01
In this example, we're creating a new Moment
object representing the current date and time. We can then format it using various patterns based on our requirements.
⨠Call-to-Action:
Now that you know how to express date types in TypeScript using moment.js
, why not give it a try? Install moment.js
in your project and start manipulating and formatting dates with ease!
š Remember, TypeScript is all about making your code more robust and less error-prone. By using appropriate types, like moment.Moment
for dates, you ensure better code quality and maintainability.
š If you found this guide helpful, share it with your fellow developers who might have struggled with expressing date types in TypeScript, just like you did! Happy coding! š
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
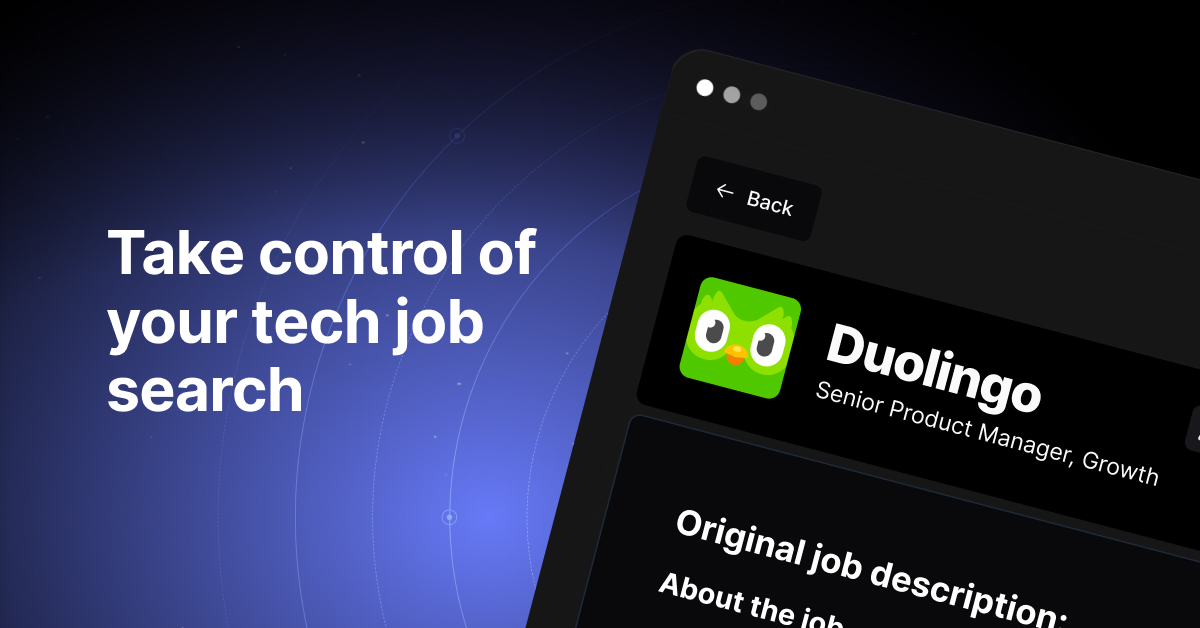