What is an ORM, how does it work, and how should I use one?
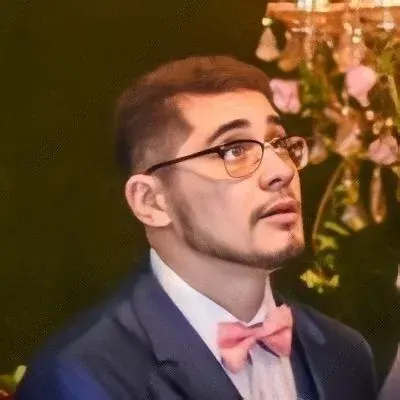
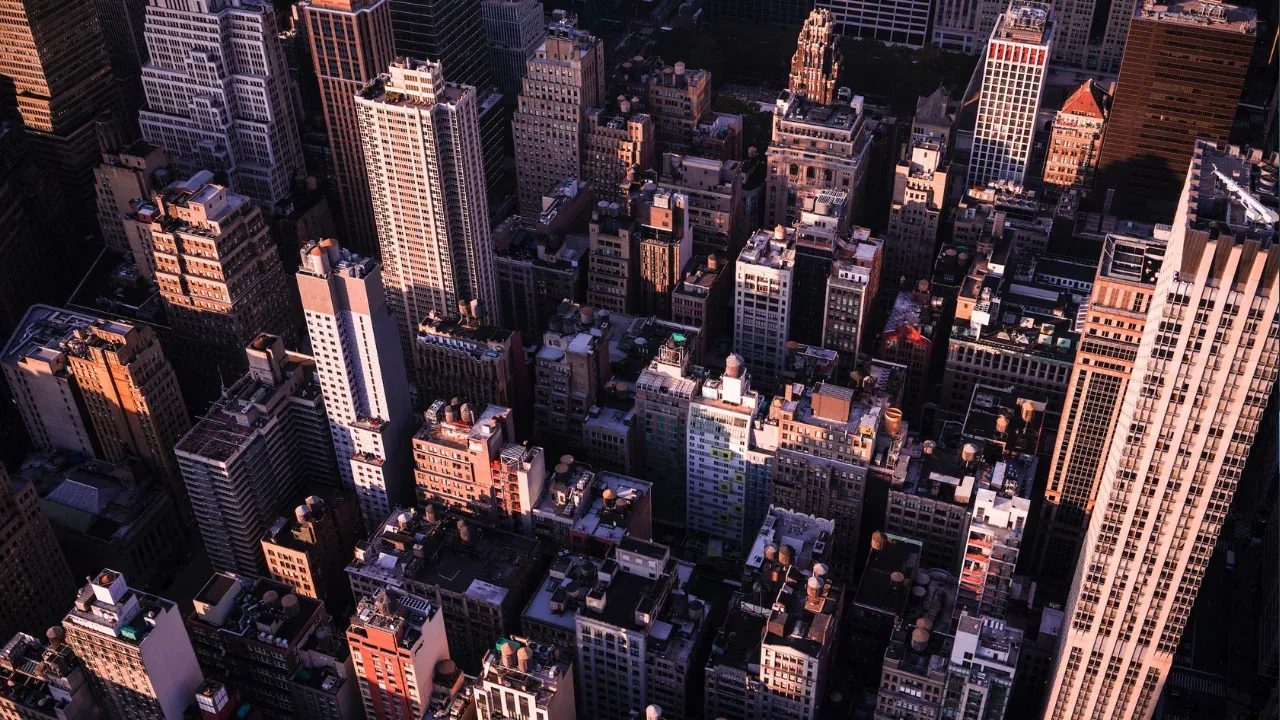
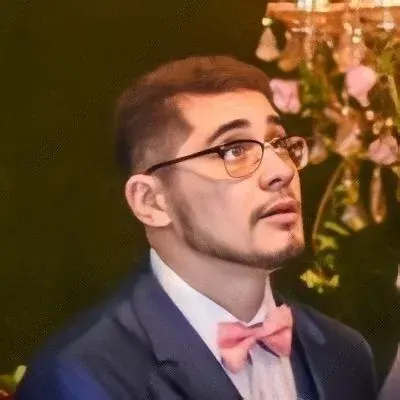
What is an ORM, how does it work, and how should I use one?
You've probably heard the term "ORM" thrown around in the world of software development, but what exactly does it mean? π€ An ORM, short for Object-Relational Mapping, is a fancy way of saying it's a way to interact with your database using objects instead of raw SQL queries. ποΈβ¨
Understanding ORM π
Traditionally, when working with a database, you would need to write SQL queries to retrieve and manipulate data. While SQL is a powerful language, it can be quite complex and hard to manage as your application grows. This is where an ORM comes in to save the day! π¦ΈββοΈ
An ORM acts as a bridge between your application's code and the database, handling all the database interactions for you. Instead of manually writing SQL queries, you can work with objects and methods that closely resemble the structure of your database tables. π’π§
How does an ORM work? π
Under the hood, an ORM uses a technique called "mapping" to translate the objects in your code to rows in the database tables and vice versa. It provides a layer of abstraction, hiding away the complexities of the database and allowing you to focus on writing clean and maintainable code. π οΈπ»
Here's a simplified example to illustrate how an ORM works:
# Define a User class using an ORM (Python example)
class User:
def __init__(self, name, email):
self.name = name
self.email = email
# Create a new user
new_user = User(name="John Doe", email="john.doe@example.com")
# Save the new user to the database
new_user.save()
In this example, instead of manually writing an SQL INSERT query, the ORM creates the query for you based on the attributes of the User
object. It saves you from dealing with the nitty-gritty details of the database, like establishing connections, handling transactions, and executing queries. π
How should I use an ORM? π€·ββοΈ
When it comes to using an ORM, there are a few best practices to keep in mind:
Choose the right ORM: There are various ORM options available for different programming languages, such as Django ORM for Python, Hibernate for Java, and Sequelize for JavaScript. Research and pick the one that aligns with your project's requirements and programming language of choice. ππ
Model your database: Before diving into using an ORM, take the time to design your database schema correctly. Define your tables, relationships, and constraints to ensure your ORM can map your objects accurately. Planning ahead will save you headaches down the road. πβοΈ
Learn the ORM's syntax: Each ORM has its own syntax and conventions for interacting with the database. Familiarize yourself with the documentation and learn about the available methods and how to use them effectively. The ORM's website and community forums can be excellent resources for learning. π©βπ«π¨βπ«
Optimize queries: While ORM simplifies your database interactions, inefficient queries can still impact performance. Ensure you know how to write efficient queries using the ORM's features like indexing, joins, and caching. This will help your application run smoothly even with a large dataset. β±οΈπͺ
That's it! You're now equipped with the basics of ORM and how to get started using one. Remember, the key to mastering an ORM is practice and experimentation. Don't hesitate to start a small project or experiment with a tutorial to gain hands-on experience. π
Conclusion π
Using an ORM can streamline your development process, reduce the amount of boilerplate code, and make your life as a developer much easier. So why not give it a try on your next project? Embrace the power of ORM and let it handle the heavy lifting while you focus on building amazing applications! πͺπ»
Do you have any questions or experience with using an ORM? Share your thoughts and let's start a discussion in the comments below! ππ£οΈ