How to Move bottomsheet along with keyboard which has textfield(autofocused is true)?
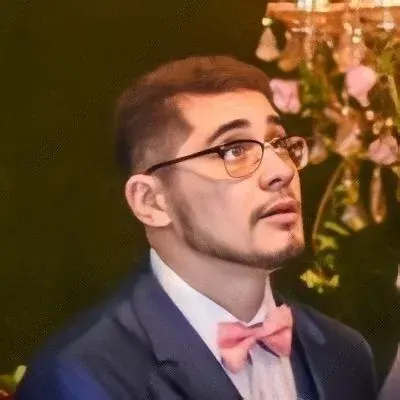
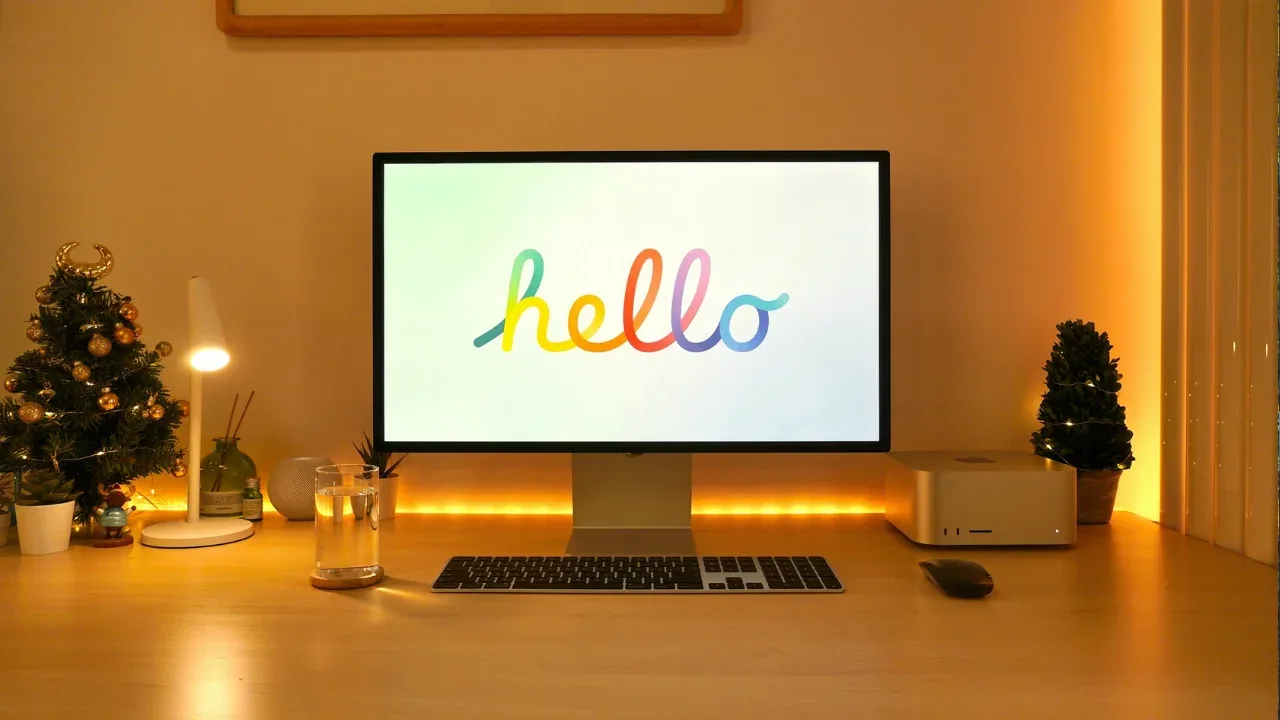
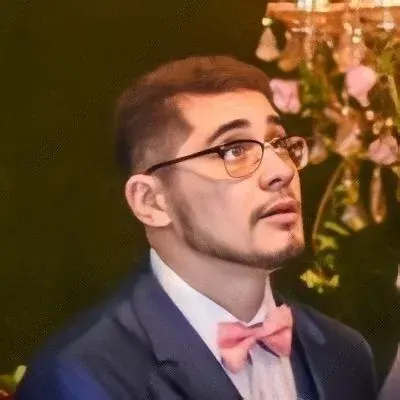
š Title: How to Move Bottom Sheet Above the Keyboard when TextField is Autofocused?
š” Introduction: Do you want to create a bottom sheet with an autofocused text field, but you're finding that the keyboard is overlapping the bottom sheet? No worries! In this article, we will discuss a simple technique to move the bottom sheet above the keyboard when the text field is autofocused. Let's dive in!
š Problem: The bottom sheet is getting overlapped by the keyboard when the text field inside it is autofocused.
šÆ Solution:
To solve this issue, we can make use of the MediaQuery
class to get the bottom offset of the keyboard and apply a padding to the bottom of the bottom sheet. Let's see the code:
Padding(
padding: EdgeInsets.only(bottom: MediaQuery.of(context).viewInsets.bottom),
child: Column(
children: <Widget>[
TextField(
autofocus: true,
decoration: InputDecoration(hintText: 'Title'),
),
TextField(
decoration: InputDecoration(hintText: 'Details!'),
keyboardType: TextInputType.multiline,
maxLines: 4,
),
TextField(
decoration: InputDecoration(hintText: 'Additional details!'),
keyboardType: TextInputType.multiline,
maxLines: 4,
),
],
),
);
By adding the MediaQuery.of(context).viewInsets.bottom
as the bottom padding of the Padding
widget, the text fields in the bottom sheet will be visible above the keyboard.
šØāš» Example and Explanation:
Let's break down the code to understand how it works.
We wrap our bottom sheet content with a
Padding
widget.The
padding
parameter of thePadding
widget is set toEdgeInsets.only(bottom: MediaQuery.of(context).viewInsets.bottom)
. This ensures that the bottom padding is set to the height of the keyboard.Inside the
Column
widget, we have our text fields. The first text field is set to autofocus so that the keyboard pops up automatically.The other text fields may contain additional details or multiline text, depending on your requirements.
šŖ Call-to-Action: Implementing this technique will help you create a more user-friendly experience in your app, where the bottom sheet adapts to the keyboard and remains visible to the user. So why wait? Give it a try in your own project and let us know if you have any questions or face any issues.
š Conclusion: We hope this guide has helped you in moving the bottom sheet above the keyboard when the text field is autofocused. The solution is simple, and it enhances the user experience in your app. Remember to always consider your users' needs and create engaging interfaces. Happy coding! š»š
š„š©š¦šŖšØš§š«šŖšØš©š„š¦šŖšØš©š„šš¦š©š¦šŖšØšŖš«š©š§šŖšØš©š§šØšØšŖ