How can I hide letter counter from bottom of TextField in Flutter
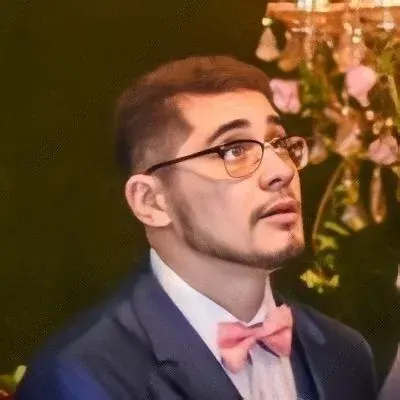
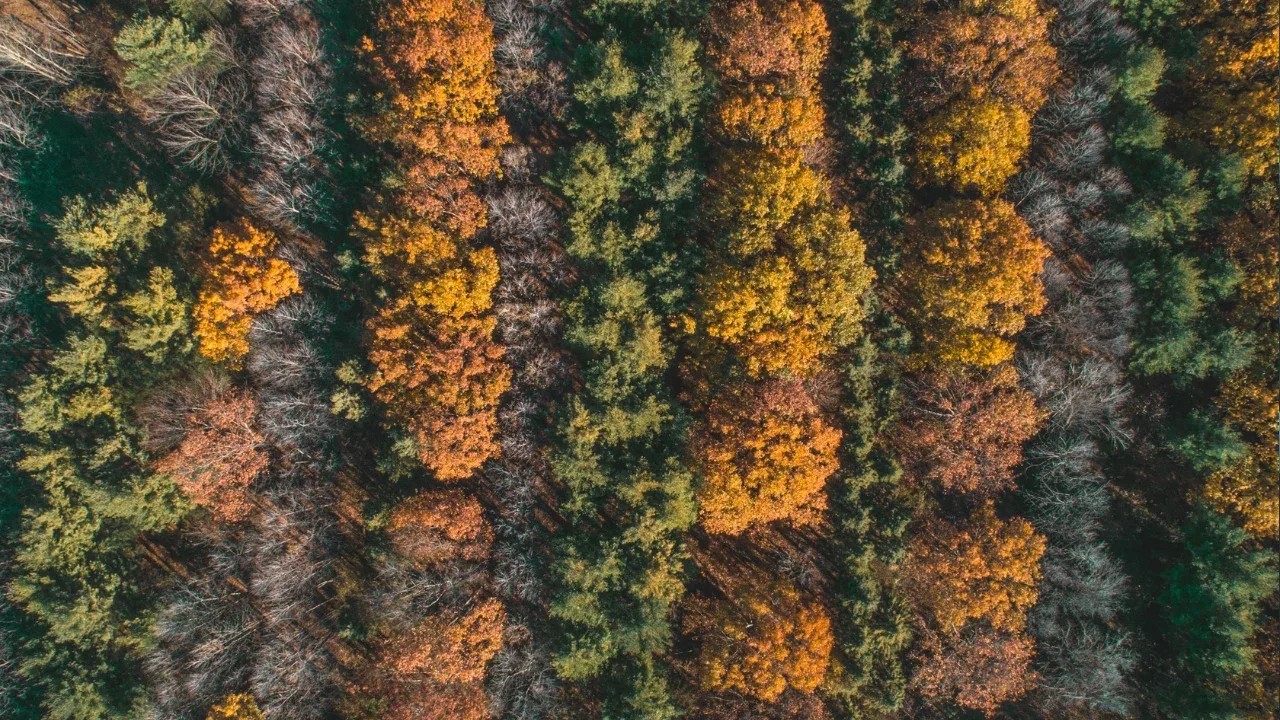
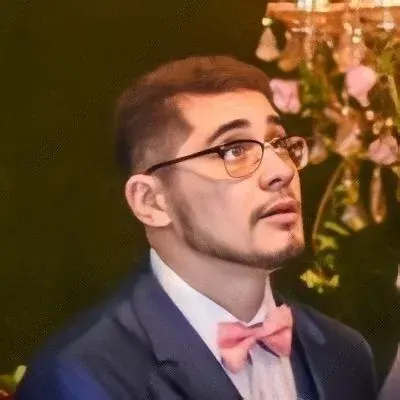
How to Hide the Letter Counter from the Bottom of a TextField in Flutter 🚀
Are you looking to hide that pesky letter counter from the bottom of a TextField in your Flutter app? You're in luck! In this guide, we'll walk you through the process step-by-step. Say goodbye to the unnecessary clutter and hello to a cleaner user interface!
The Problem 🤔
So, you've set the maxLength
property of your TextField to 1, but that pesky letter counter label is still appearing at the bottom. Frustrating, right? But fret not! We've got you covered with a quick and easy solution.
The Solution 💡
To hide the letter counter label from your TextField, follow these simple steps:
Wrap your TextField widget with a
Stack
widget. TheStack
widget allows you to position items on top of each other.
Stack(
children: [
// Your TextField widget goes here
TextField(
maxLength: 1,
// Other TextField properties
),
// Other widgets can be added to the stack
],
)
Add a
Positioned
widget as a child of theStack
widget. ThePositioned
widget allows you to precisely position its child within the stack.
Stack(
children: [
TextField(
maxLength: 1,
// Other TextField properties
),
Positioned(
bottom: -100, // Adjust this value based on your needs
child: Container(
// You can customize the container with your desired styles
color: Colors.transparent,
),
),
],
)
Customize the
Positioned
widget'sbottom
property to push the letter counter label out of view. The negative value ensures that the container is positioned below the visible area of the TextField.Optionally, you can customize the
Container
widget to match the theme of your app. You can change its background color, add a border, or apply any other styles that suit your design.
That's it! You've successfully hidden the letter counter from the bottom of your TextField in Flutter. Enjoy the cleaner and more streamlined user interface!
Share Your Experience! 😃
We hope this guide has helped you solve the problem of hiding the letter counter label from your TextField in Flutter. Give it a try and let us know in the comments below if it worked for you! We'd love to hear about your experience.
If you found this guide helpful, share it with your fellow Flutter developers. Remember, sharing is caring! Let's help each other create amazing Flutter apps.
Happy coding! 💻🔥