Flutter: Run method on Widget build complete
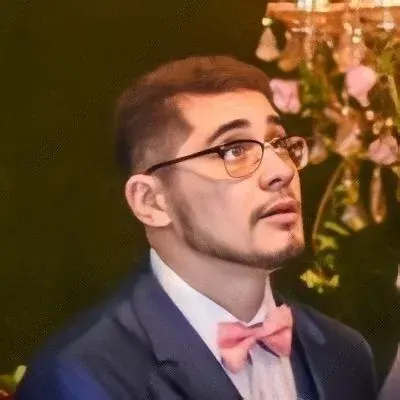
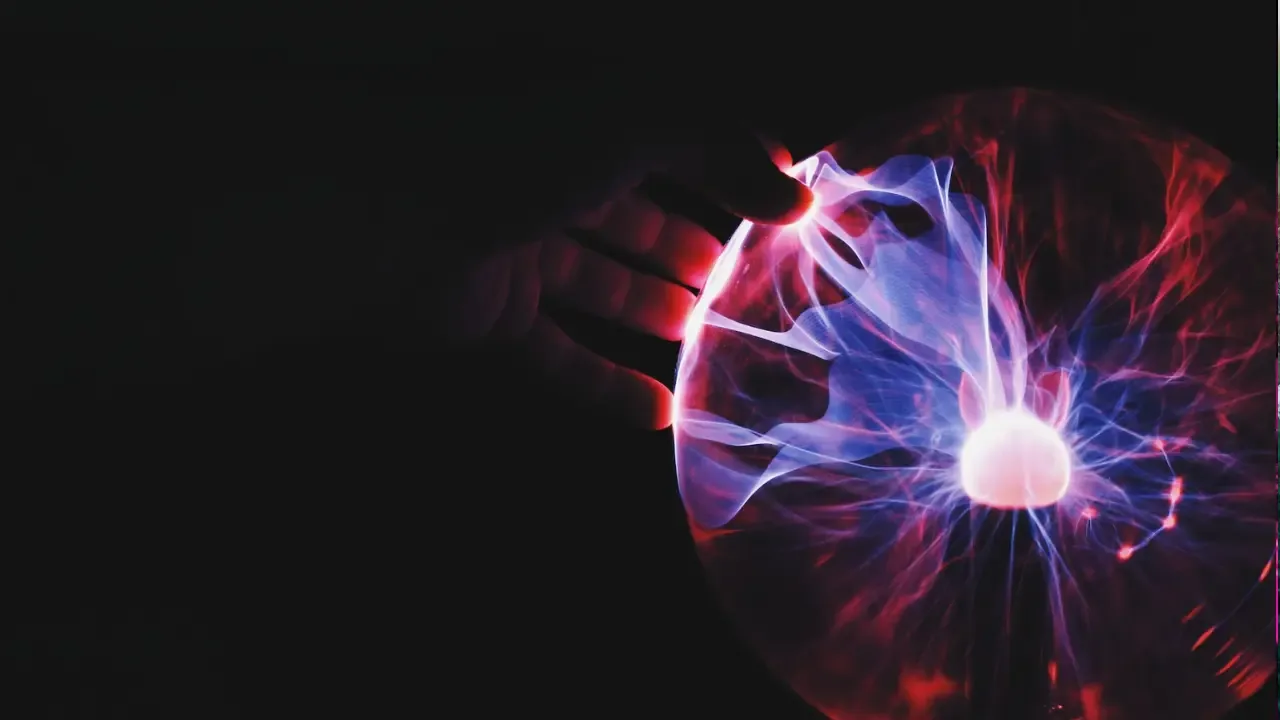
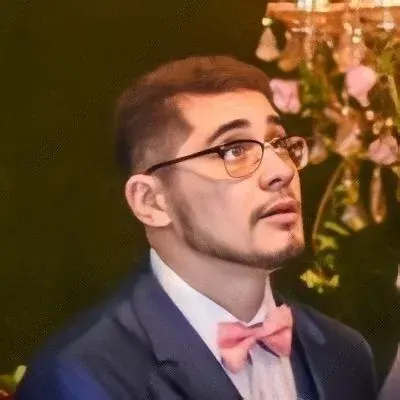
Flutter: Running a Method on Widget Build Complete 💥🔧
Have you ever wanted to execute a method once a Flutter widget has finished building and loading? 🤔 Maybe you're facing a situation where you need to check if a user is authenticated and redirect them to a login view if they're not. But here's the catch - you don't want to check this before and navigate to the login view or the main view. You want it to happen after the main view has finished loading. 🚀
In this blog post, we'll dive into some common issues and explore easy solutions to help you achieve your desired outcome. So let's get started! 🎉
Understanding the Problem
When you build a Flutter widget, the build()
method is called to generate the widget's UI on the screen. However, there isn't a built-in method that runs after the build process is complete. This can make it challenging to perform actions right after the widget has finished loading. 😬
Solution 1: Using a PostFrameCallback
One straightforward solution to this problem is using a PostFrameCallback
. This class allows you to register a callback function that runs immediately after the widget's frame (or UI) has been painted on the screen. 🖌️ This is the perfect opportunity to execute your desired method.
Here's an example of how you can implement this solution:
import 'package:flutter/scheduler.dart';
// Inside your widget's class
@override
void initState() {
super.initState();
WidgetsBinding.instance.addPostFrameCallback((_) {
// Your method here
checkAuthentication();
});
}
The addPostFrameCallback
takes a function as an argument, which will be executed once the widget's frame has been painted. In our example, we call the checkAuthentication()
method.
This solution ensures that your method will only run once, right after the widget has finished building and loading. 🎯
Solution 2: Utilizing the Future.delayed
Method
Another way to achieve your desired outcome is by utilizing the Future.delayed
method. This method allows you to introduce a small delay before executing a particular function. By using a delay of 0 seconds, you can ensure the method runs after the widget has completed its build process.
Here's how you can use Future.delayed
to achieve this:
import 'dart:async';
// Inside your widget's class
@override
void initState() {
super.initState();
Future.delayed(Duration.zero, () {
// Your method here
checkAuthentication();
});
}
This code snippet schedules the execution of the checkAuthentication()
method with a delay of 0 seconds. As a result, the method will run right after the widget's build process is complete. 🕒
Engage with the Community 🤝
Now that you've learned a couple of solutions to run a method on Widget build complete, it's time to put this knowledge into action! 🚀
We encourage you to try out these solutions in your Flutter projects and share your experiences with the Flutter community. If you have any questions or suggestions, feel free to leave a comment below. Let's learn and grow together! 🌱💪
Happy Fluttering! ✨🦋