Flutter get context in initState method
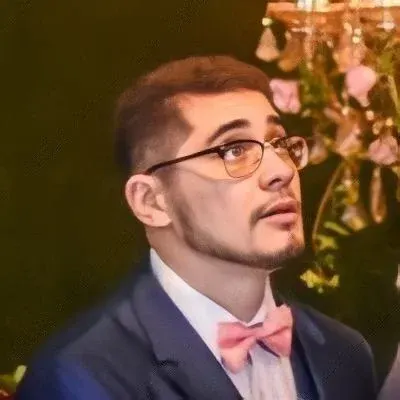
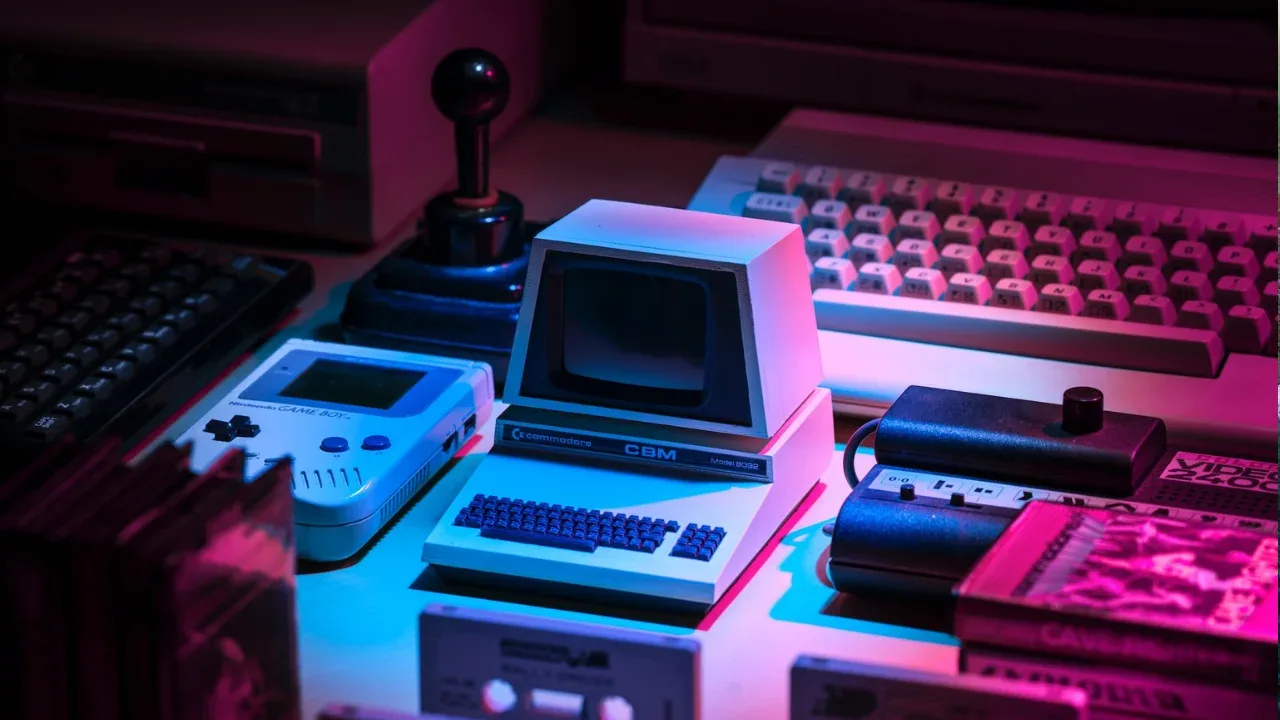
🖥️📝🚀 Hey there, Flutter enthusiasts! Are you struggling to get the context in the initState
method? 🤔 Don't worry, you're not alone! Many developers face this challenge when trying to perform certain checks upon page rendering and displaying an AlertDialog
if necessary. In this blog post, we'll tackle this issue head-on and provide you with easy solutions. So, stick around and let's dive in! 💪
The code snippet you shared demonstrates the usage of the initState
method and the _showConfiguration
function. Your goal is to show the AlertDialog
when the page is rendered, based on certain checks. Let's explore how you can achieve this and improve your implementation. 🕵️
To get the context in the initState
method, you can use the following approach:
@override
void initState() {
super.initState();
WidgetsBinding.instance.addPostFrameCallback((_) {
if (!_checkConfiguration()) {
_showConfiguration(context);
}
});
}
By using WidgetsBinding.instance.addPostFrameCallback
, you can access the context in the initState
method, ensuring that it is available after the first frame is rendered. This way, you won't encounter any issues while displaying the AlertDialog
. 🎉
Now, let's take a closer look at the _showConfiguration
method. You've already done a great job of creating and displaying the AlertDialog
. However, I noticed that you mentioned wanting a callback function that can be assigned to the build
function to be called on render. While there isn't a specific callback for that, you can achieve a similar effect by using the Builder
widget and creating a separate function to handle your configuration checks:
void _checkAndShowConfiguration(BuildContext context) {
if (!_checkConfiguration()) {
_showConfiguration(context);
}
}
@override
Widget build(BuildContext context) {
return Scaffold(
// Other widget configurations
body: Builder(
builder: (BuildContext context) {
_checkAndShowConfiguration(context);
return Container(
// Your widget tree
);
},
),
);
}
By using the Builder
widget, you can ensure that the BuildContext
is available, which allows you to call the _checkAndShowConfiguration
function within the build
method. This way, your checks will be performed whenever the widget tree is built, ultimately displaying the AlertDialog
if necessary. 📊
Remember that it's crucial to avoid infinite loops when calling functions inside the build
method. Make sure to only call them based on specific conditions or flags to prevent unnecessary re-renders. 🔄
If you're still facing any issues or would like to explore alternative solutions, check out the related stack overflow question you mentioned: Flutter Redirect to a page on initState. It might provide additional insights from the Flutter community. 😊
Now that you're armed with these easy solutions, go ahead and implement them in your code. Don't forget to share your success stories and any other questions you might have in the comments section below. Let's learn and grow together as Flutter developers! 👩💻👨💻
Happy Fluttering! 🚀✨
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
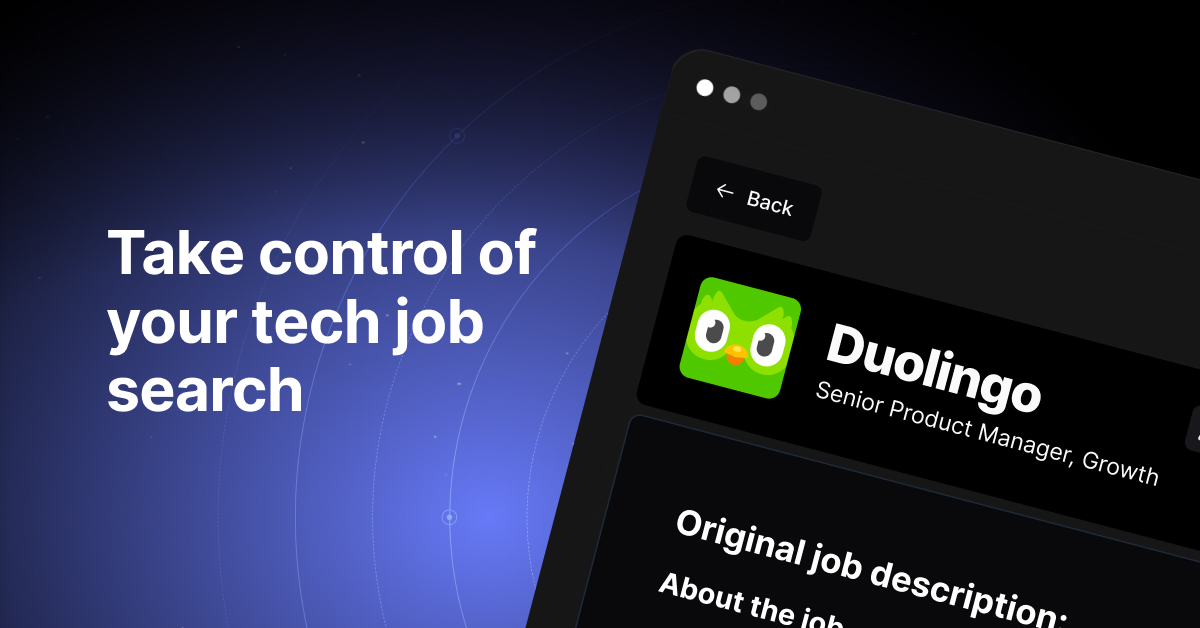