Enumerate or map through a list with index and value in Dart
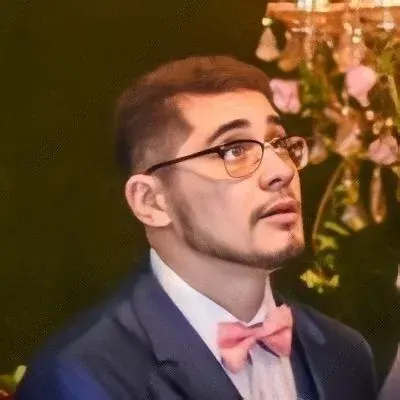
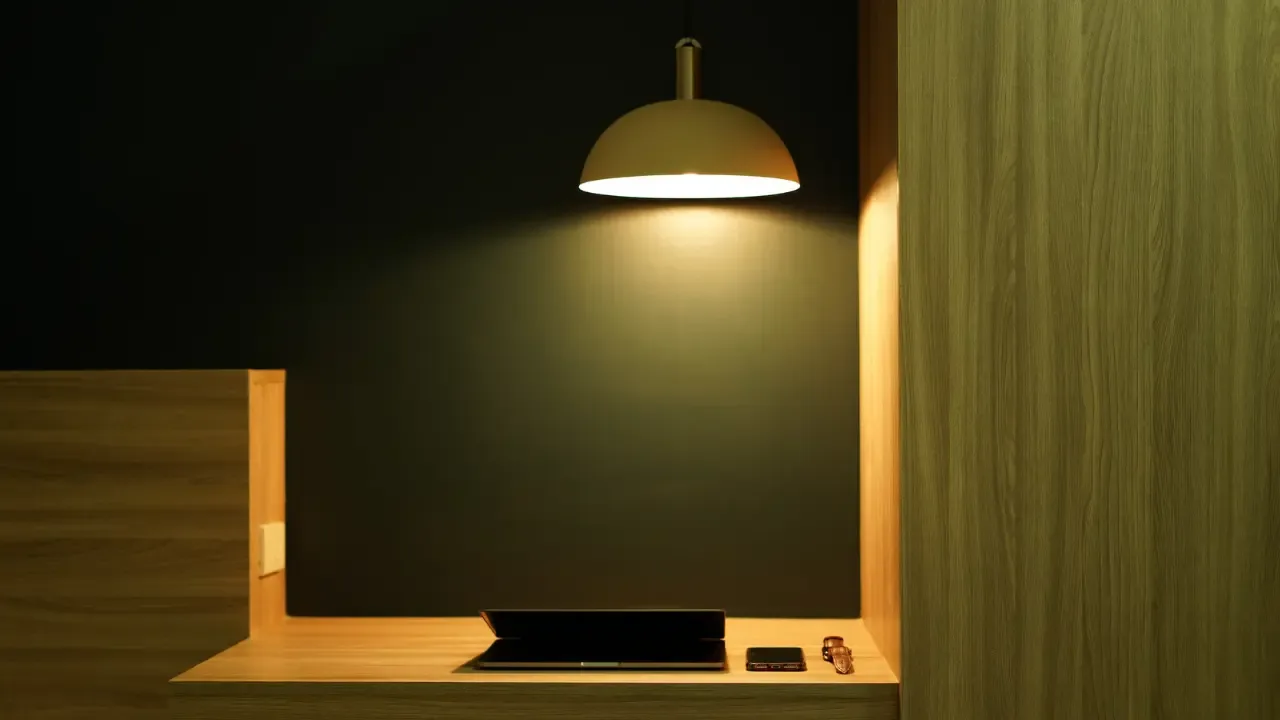
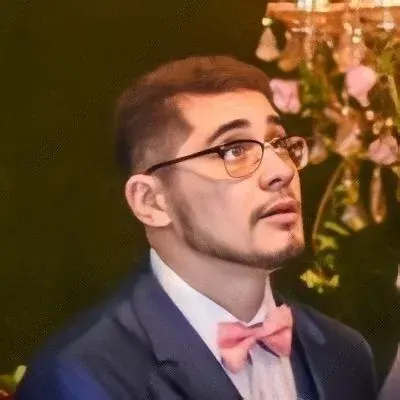
Mapping Through a List with Index and Value in Dart: A Complete Guide
Do you often find yourself in a situation where you need to iterate through a list in Dart and access both the index and the value? Maybe you're wondering if there's a built-in function like enumerate
in Python that can simplify this task. Well, you're not alone! Many developers have expressed the same concern.
In this blog post, we will explore this common issue and provide you with easy solutions to map through a list with index and value in Dart. So, let's dive in and find out how we can tackle this problem!
The Quest for enumerate
or map
Functionality in Dart
Before we continue, it is important to note that Dart doesn't provide a built-in enumerate
or map
function like the ones present in other languages. However, fear not! There are alternative approaches that can help us achieve the desired functionality.
One of the simplest and most straightforward ways to map through a list in Dart is by using the Iterable
class, generate
method, and forEach
loop. Here's an example to help you visualize the solution:
Iterable<int>.generate(list.length).forEach((index) {
// Access the value using list[index]
// Perform your desired operation with index and value
});
In the code snippet above, we use Iterable<int>.generate
to create an iterable range of numbers from 0 to list.length - 1
. Then, we run a forEach
loop on this range, allowing us to access the index. Inside the loop, you can easily access the corresponding value using list[index]
and perform any necessary operations.
Exploring the Solution with Examples
To give you a clear understanding of how this solution works, let's walk through a couple of examples.
Example 1: Printing Index and Value
Let's say we have a list of names and we want to print each name along with its index. Using our solution, we can achieve this as follows:
List<String> names = ['Alice', 'Bob', 'Charlie'];
Iterable<int>.generate(names.length).forEach((index) {
print('Index: $index, Name: ${names[index]}');
});
Output:
Index: 0, Name: Alice
Index: 1, Name: Bob
Index: 2, Name: Charlie
Example 2: Creating a New List with Modified Values
Suppose we have a list of numbers and we want to create a new list where each value is multiplied by its index. Our solution can help us accomplish this task effortlessly:
List<int> numbers = [1, 2, 3, 4, 5];
List<int> multipliedList = [];
Iterable<int>.generate(numbers.length).forEach((index) {
int multipliedValue = numbers[index] * index;
multipliedList.add(multipliedValue);
});
print(multipliedList);
Output:
[0, 2, 6, 12, 20]
In the example above, we iterate through each index of the numbers
list and multiply the corresponding value by the index. The modified values are then added to the multipliedList
. Finally, we print the resulting list.
It's Not enumerate
, But It Gets the Job Done!
Although Dart doesn't offer a built-in enumerate
or map
function, our alternative solution leveraging Iterable<int>.generate
and forEach
enables us to iterate through a list while accessing the index and value effortlessly. The examples provided should give you a good starting point to work with.
If you have any other clever techniques or suggestions for mapping through a list with index and value in Dart, we would love to hear from you! Share your thoughts in the comments below and let's help each other level up our Dart skills.
So, what are you waiting for? Give it a try in your Dart projects and conquer the world of list iteration!
🌟 Your Turn: Get Creative and Share Your Experience!
Now that you have learned how to map through a list with index and value in Dart, it's time to put your skills to the test! Think of a real-world scenario where this functionality could come in handy, and share your experience with us. We can't wait to hear all about it!
Remember, sharing your knowledge not only helps others but also enhances your own understanding of the topic. So, go ahead and leave a comment below. Let's engage in a fruitful discussion and learn from each other.
Happy coding! ✨🚀