Converting timestamp
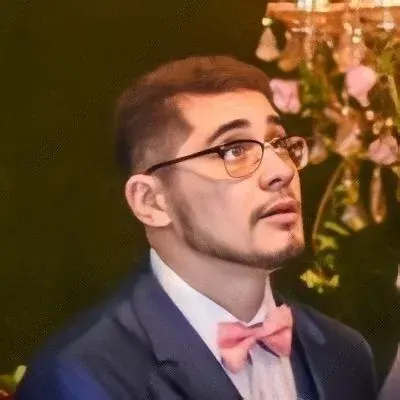
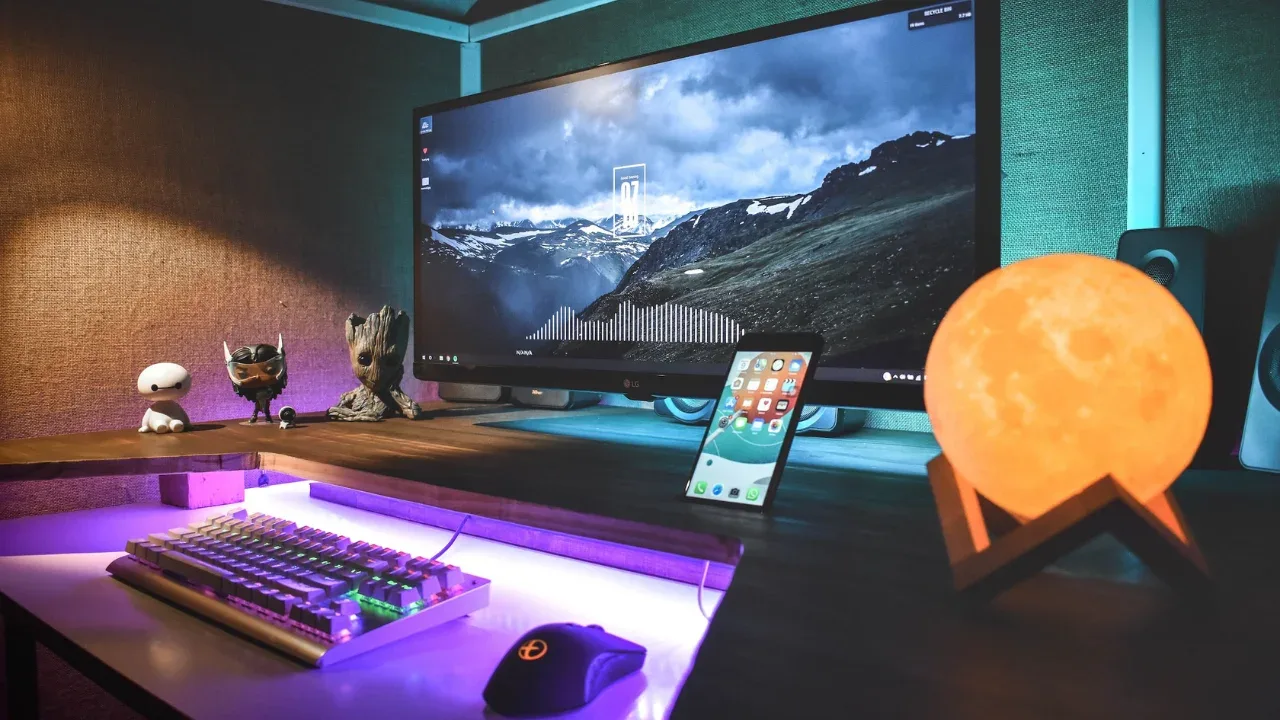
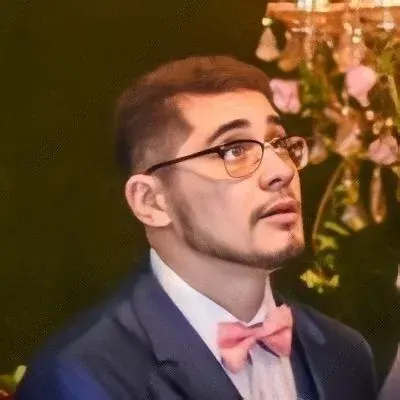
š Converting Timestamps: Easy Solutions for Common Issues š°ļø
š Hey there, tech enthusiasts! If you're struggling with converting timestamps and need easy solutions, you've come to the right place. š
š” Recently, I stumbled upon a question from a fellow developer who was grappling with converting a timestamp from Firebase into a readable date format. The timestamp looked like this: 1522129071. They needed help on how to convert it into a date. Let's dive right in and solve this problem together! šŖ
š¤ A Swift Solution:
The first code snippet shared by the developer showcases a solution in Swift. Here's a simplified breakdown of the steps:
Define the
readTimestamp
function that accepts anInt
parameter,timestamp
.Get the current date and time using
Date()
and store it in thenow
variable.Create an instance of
DateFormatter
to format the date later on.Use
Date(timeIntervalSince1970: Double(timestamp))
to convert the timestamp into aDate
object.Define a set of
Calendar.Component
that you want to extract from the date, in this case: seconds, minutes, hours, days, and weeks.Calculate the time difference between the obtained date and the current date using
Calendar.current.dateComponents
.Initialize an empty string,
timeText
, to store the formatted time.Set the
locale
of thedateFormatter
to.current
and thedateFormat
to the desired time format, in this case: "HH:mm a".Check if the time difference is less than or equal to 0 seconds or if it's greater than 0 seconds and there are no minutes, hours, or days difference. If so, format the date using
dateFormatter.string(from: date)
.If there is a day difference, format the day message accordingly.
If there is a week difference, format the week message accordingly.
Finally, return the formatted
timeText
.
š An Attempt in Dart:
The developer also shared their attempt at solving the same problem in Dart. However, they encountered issues where the converted dates and times seemed way off. Here's the original code snippet:
String readTimestamp(int timestamp) {
var now = new DateTime.now();
var format = new DateFormat('HH:mm a');
var date = new DateTime.fromMicrosecondsSinceEpoch(timestamp);
var diff = date.difference(now);
var time = '';
if (diff.inSeconds <= 0 || diff.inSeconds > 0 && diff.inMinutes == 0 || diff.inMinutes > 0 && diff.inHours == 0 || diff.inHours > 0 && diff.inDays == 0) {
time = format.format(date); // Doesn't get called when it should be
} else {
time = diff.inDays.toString() + 'DAYS AGO'; // Gets call and it's the wrong date
}
return time;
}
š Troubleshooting the Dart Solution:
Upon analysis, the issue with the Dart code seems to lie in correctly converting the timestamp to a DateTime
object. The timestamp
value provided is in seconds, but the fromMicrosecondsSinceEpoch
method expects the timestamp in microseconds. To fix this, we need to multiply the timestamp by 1000.
Additionally, there's a minor typo in the day formatting. The code uses 'DAYS AGO'
, but it should be ' DAY AGO'
for singular day cases.
Check out the updated code snippet below:
String readTimestamp(int timestamp) {
var now = new DateTime.now();
var format = new DateFormat('HH:mm a');
var date = new DateTime.fromMicrosecondsSinceEpoch(timestamp * 1000); // Multiply the timestamp by 1000 to convert it into microseconds
var diff = date.difference(now);
var time = '';
if (diff.inSeconds <= 0 || diff.inSeconds > 0 && diff.inMinutes == 0 || diff.inMinutes > 0 && diff.inHours == 0 || diff.inHours > 0 && diff.inDays == 0) {
time = format.format(date); // Gets called correctly now
} else {
if (diff.inDays == 1) {
time = diff.inDays.toString() + ' DAY AGO'; // Corrected typo for singular day
} else {
time = diff.inDays.toString() + ' DAYS AGO';
}
}
return time;
}
š Congrats! You're all set with a revised and working Dart solution. š
⨠In conclusion, converting timestamps doesn't have to be a headache. Whether you're working with Swift or Dart, the key lies in understanding the underlying concepts and being aware of any nuances, such as unit conversion or formatting discrepancies.
š¤ If you found this guide helpful or have any other doubts, feel free to leave a comment below. Let's geek out together and solve problems as a community! š¬š©āš»šØāš»
āļø Keep on coding and converting timestamps like a pro! ā°ā¤ļø