What is the best way to conditionally apply a class?
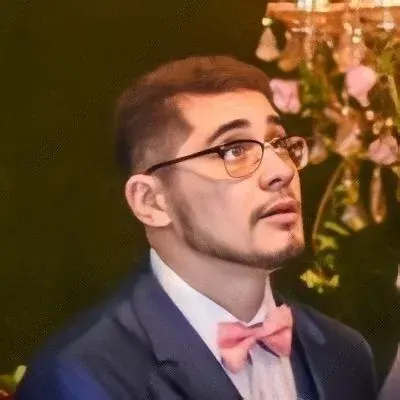
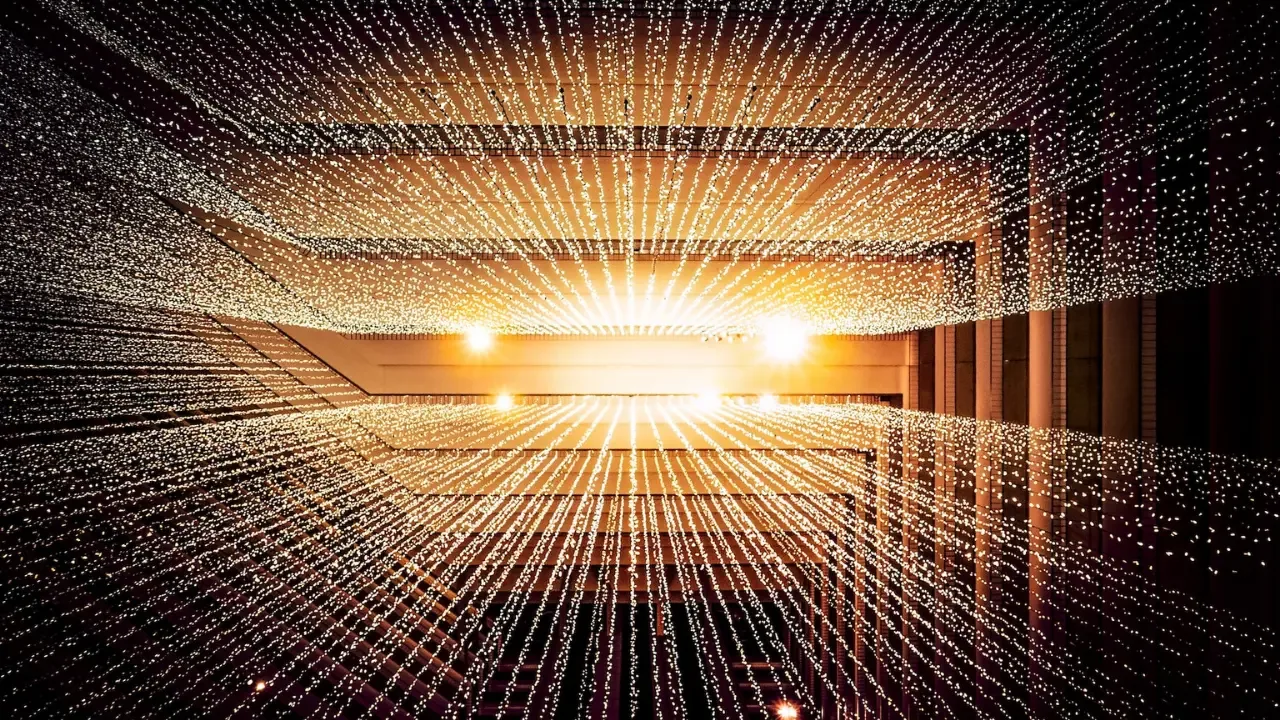
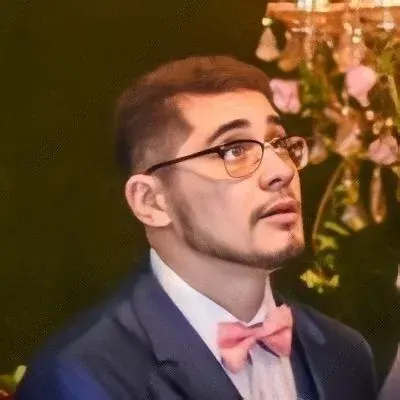
ππ€ What is the best way to conditionally apply a class? ποΈββοΈ
So, you've got an array that you're rendering as a "ul" with "li" elements in it. And you also have a "selectedIndex" property on your controller. Now, the burning question is: what's the best way to dynamically add a class to the "li" element with the index matching the "selectedIndex" value in AngularJS? π€
π‘ The Dilemma
As explained by our friend here, the current approach involves duplicating the "li" element code manually and adding the class to the desired one. This is followed by using either "ng-show" or "ng-hide" directives to show a specific "li" based on the index value.
π The Quest for Simplicity
However, there's a much simpler and more efficient solution to this conundrum! Let's dive into it, shall we? πͺ
π‘ The Solution: "ng-class" Directive
The AngularJS framework offers the "ng-class" directive, which allows you to conditionally apply a class to an element based on a given expression. This directive will be our superhero in solving this problem. π¦ΈββοΈ
Here's how you can incorporate "ng-class" into your code to conditionally apply a class to the desired "li" element:
<ul>
<li ng-repeat="item in items" ng-class="{ 'selected': $index === selectedIndex }">{{ item }}</li>
</ul>
In the code snippet above, we utilize "ng-repeat" to iterate over each item in the "items" array. Then, we apply the "ng-class" directive to each "li" element.
The expression within "ng-class" compares the index of each "li" element with the "selectedIndex" value. If they match, the "selected" class will be applied. Magic! β¨
π Beyond the Basics
But wait, there's more! "ng-class" offers even more flexibility. Instead of just an object literal, you can also use a function to dynamically determine the class to apply. Here's an example:
<ul>
<li ng-repeat="item in items" ng-class="getClass($index)">{{ item }}</li>
</ul>
In this case, we have a function called "getClass" defined in our controller that takes the index as a parameter and returns the desired class name. This function can have complex logic, making it adaptable to various scenarios.
π‘ The Call-to-Action
Now that you've learned the best way to conditionally apply a class in AngularJS using the "ng-class" directive, it's time for you to level up your coding skills! Try implementing this technique in your upcoming projects and see the difference it makes. Feel free to share your discoveries and ask any questions in the comments below. Let's embark on this class-applying adventure together! ππ»
So, what are you waiting for? πͺ Start coding with style and impress your teammates and friends with your newfound AngularJS superpowers! ππ