Setting width/height as percentage minus pixels
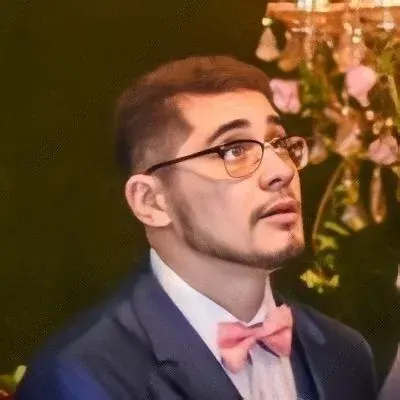
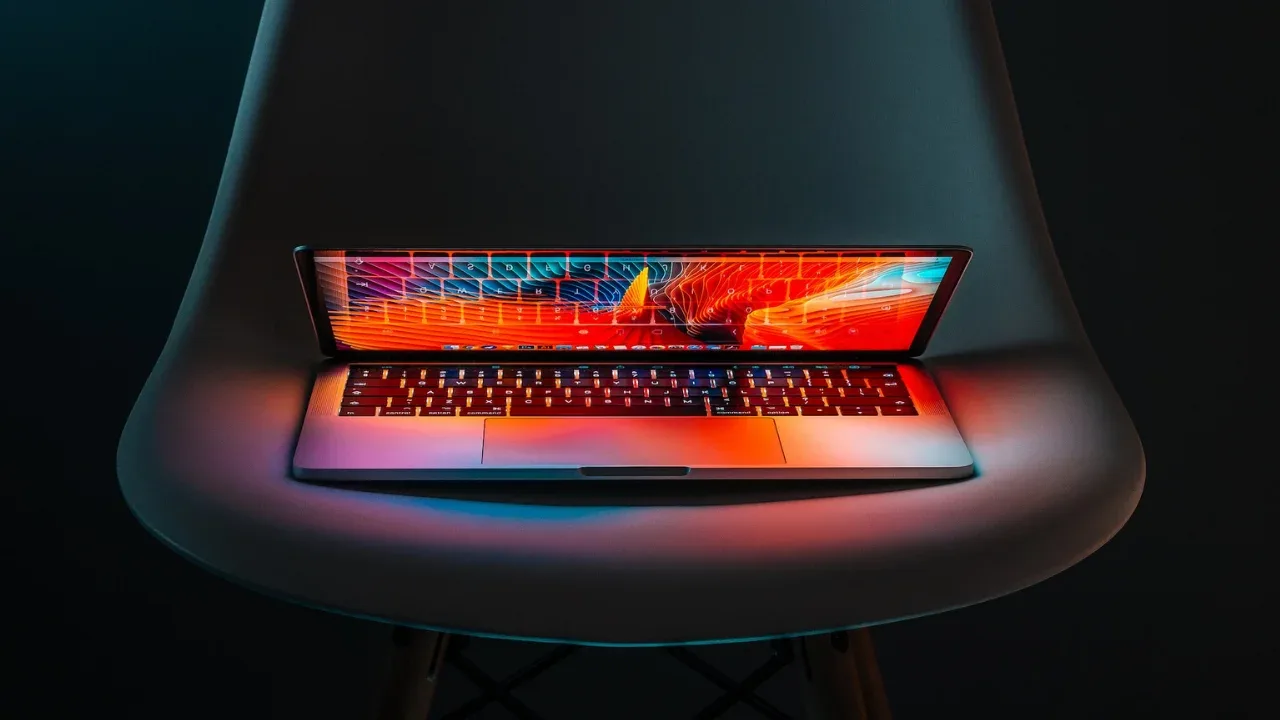
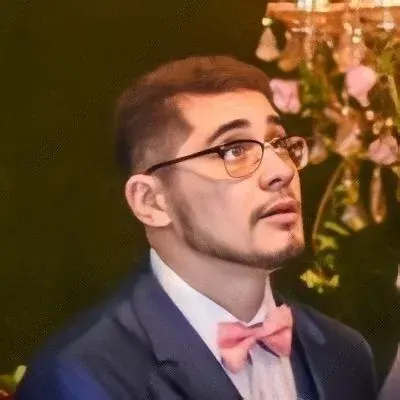
📝 Setting width/height as percentage minus pixels
Are you tired of inconsistent website layouts and cluttered CSS? Do you want to standardize your code and make it more readable? We've got you covered! In this blog post, we'll tackle a common issue that many web developers face: how to set the width or height of an element as a percentage minus pixels. 📏💻
🧩 The Problem
Let's say you have a container <div>
with a dynamic height and inside it, there's a header <div>
and an unordered list. You want the unordered list to take up the remaining space in the container, but the header has a fixed height of 18px. How can you specify the list's height as "the result of 100% minus 18px"? 🤔
🔎 Finding the Solution
You're not alone in this quest for a solution. Many developers have asked the same question on Stack Overflow. However, let's dive into your specific case and find the perfect solution for you! 💪
🏢 The CSS Approach
One way to solve this problem is by using CSS flexbox. With flexbox, you can easily distribute space between elements. Here's an example of how you can achieve the desired result:
.container {
display: flex;
flex-direction: column;
}
.header {
height: 18px;
}
.list {
flex-grow: 1;
}
In this solution, we set the container's display property to flex, which allows us to manipulate the child elements easily. The header's height is fixed at 18px, but the list takes up the remaining space by using the flex-grow property set to 1. Flexbox handles the percentage calculations for you! 🎉
📋 The Math Approach
If you prefer a more mathematical approach, we can use a combination of CSS variables and calc() function. Check out this code snippet:
:root {
--header-height: 18px;
}
.container {
height: calc(100% - var(--header-height));
}
In this solution, we define a CSS variable called --header-height and set it to 18px. Then, we use the calc() function to subtract the header height from 100% and apply it as the container's height. It's a simple and elegant solution! 💫
💡 Final Thoughts
Now that you have two powerful solutions at your disposal, you can choose the one that suits your coding style and requirements. Whether you prefer the CSS flexbox approach or the math-based approach using CSS variables, you can finally achieve the consistent and clutter-free layouts you've always wanted. 😍
🤝 Your Turn
We would love to hear from you! Have you encountered this problem before? What other CSS challenges are you facing in your projects? Share your thoughts, experiences, and questions in the comments section below. Let's solve problems together and make the web a more beautiful place! 🌐💖