How do I right align div elements?
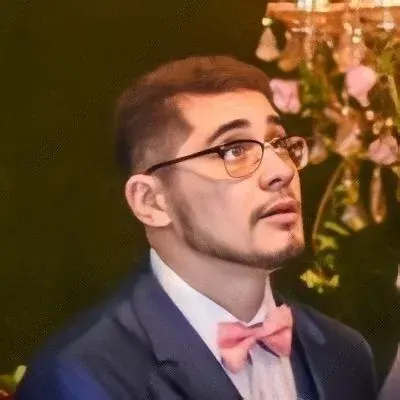
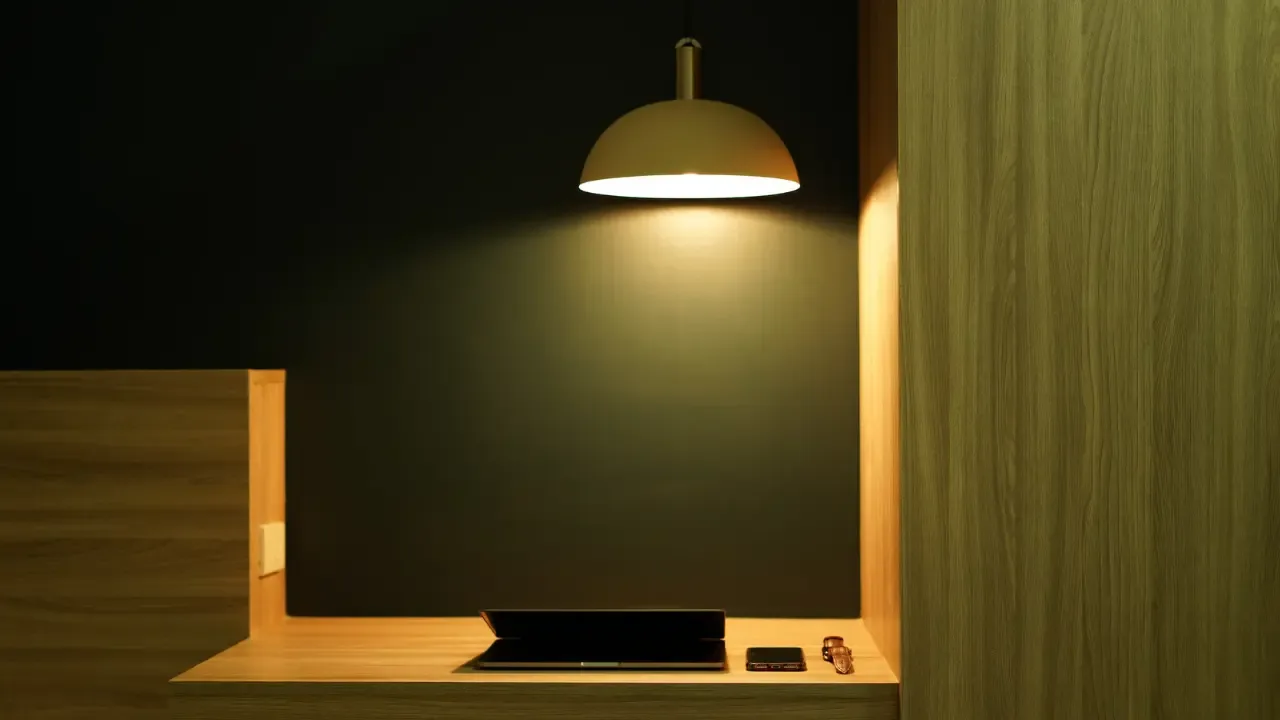
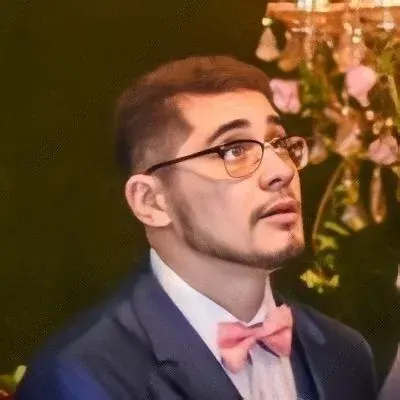
How to Right Align Div Elements in HTML
So you want to right align your button and form elements, but they end up next to each other horizontally instead of following each other vertically? Don't worry, we've got you covered! In this guide, we'll walk you through the process step by step to achieve the desired result.
The Issue
Let's take a look at the code you have so far:
<div id="button">
<button onclick="showForm()" type="button" id="cTask">
Create Task
</button>
</div>
<div id="addEventForm">
<form>
<!-- form fields here -->
</form>
</div>
And the CSS:
#button {
position: relative;
float: right;
}
#addEventForm {
position: relative;
float: right;
border: 2px solid #003B62;
font-family: verdana;
background-color: #B5CFE0;
padding-left: 10px;
}
The problem here is that when you float both the button and form to the right, they end up next to each other horizontally instead of vertically aligning one below the other.
The Solution
To fix this issue and align the button and form elements properly, you need to make a few adjustments to your CSS.
First, remove the <p>
tag around your <div>
elements. The <div>
element is a block-level element, and the <p>
tag is not necessary here.
Next, add a container <div>
around the button and form elements to group them and ensure that they follow each other vertically. Let's call this container div #container
:
<div id="container">
<div id="button">
<button onclick="showForm()" type="button" id="cTask">
Create Task
</button>
</div>
<div id="addEventForm">
<form>
<!-- form fields here -->
</form>
</div>
</div>
Now, let's update the CSS accordingly:
#container {
display: flex;
flex-direction: column;
}
#button {
margin-left: auto;
}
#addEventForm {
margin-left: auto;
border: 2px solid #003B62;
font-family: verdana;
background-color: #B5CFE0;
padding-left: 10px;
}
Here's an explanation of the changes we made:
We added a new
#container
CSS selector to group the button and form elements and useddisplay: flex;
to make the elements follow each other vertically.We set
margin-left: auto;
for both the button and form elements (#button
and#addEventForm
). This pushes them to the right side of the container div, achieving the right alignment effect you desire.We removed the
float: right;
property since we're now using flexbox for layout.
The Result
With these changes applied, your button and form elements will now be right aligned and follow each other vertically, as you intended.
Share Your Success
We hope this guide helped you successfully right align your div elements! If you found this post helpful, make sure to share it with your friends and colleagues who might be facing the same issue. Let them know they don't have to struggle with aligning div elements anymore!
And if you have any other questions or need further assistance, feel free to reach out in the comments below. We're here to help. Happy coding! 👩💻💪
<p>Your code should now look like this:</p>
<div id="container">
<div id="button">
<button onclick="showForm()" type="button" id="cTask">
Create Task
</button>
</div>
<div id="addEventForm">
<form>
<!-- form fields here -->
</form>
</div>
</div>
#container {
display: flex;
flex-direction: column;
}
#button {
margin-left: auto;
}
#addEventForm {
margin-left: auto;
border: 2px solid #003B62;
font-family: verdana;
background-color: #B5CFE0;
padding-left: 10px;
}
Don't forget to check your browser to see the beautiful right-aligned button and form elements! 🎉✨