How can I make Bootstrap columns all the same height?
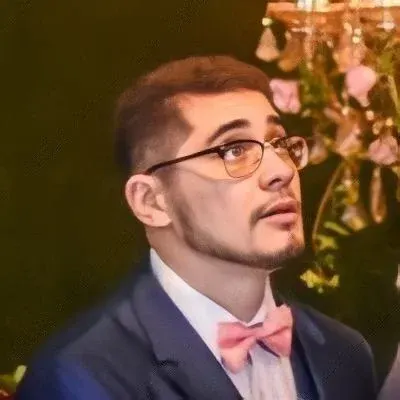
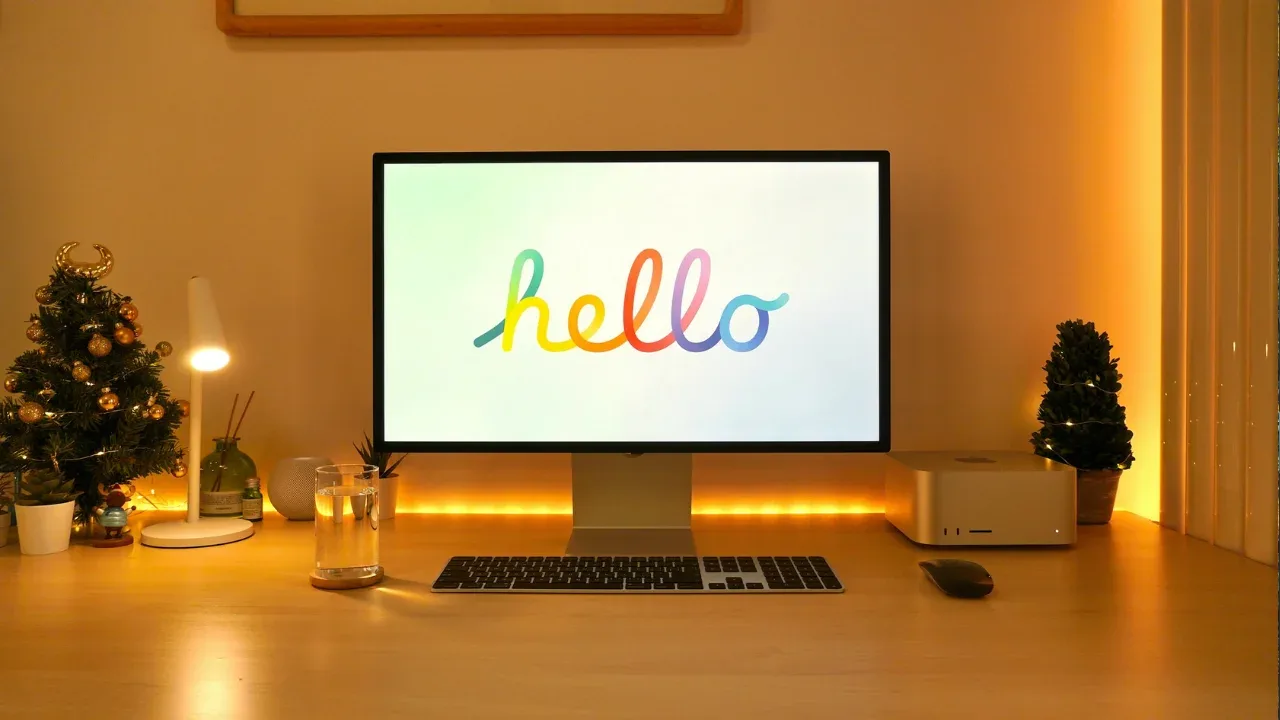
How to Make Bootstrap Columns All the Same Height? 🏗️📏
So, you're using Bootstrap and you want to make those pesky columns all the same height, huh? Don't worry, we've got your back! 👍
The Problem 😫
Let's take a look at the problem first. In the code snippet and screenshot you provided, the blue and red columns are not the same height as the yellow column. And that's not what you want, right?
The Solution 💡
Here are a couple of easy solutions to make those columns stand at the same height. 💪
1. Using Flexbox
One way to solve this issue is by using the power of Flexbox. By applying Flexbox to the row containing the columns, we can ensure that all columns will have the same height.
<div class="row d-flex">
<div class="col-xs-4 panel" style="background-color: red">
<!-- some content -->
</div>
<div class="col-xs-4 panel" style="background-color: yellow">
<!-- catz -->
<img width="100" height="100" src="https://lorempixel.com/100/100/cats/" alt="Cats">
</div>
<div class="col-xs-4 panel" style="background-color: blue">
<!-- some more content -->
</div>
</div>
By adding the d-flex
class to the row, the columns will automatically fill the available height and become the same size.
2. Using JavaScript
If you prefer a JavaScript-based solution, you can use a library like MatchHeight.js to make the columns equal in height. This library allows you to specify the elements you want to match in height.
First, make sure to include the jQuery library and the MatchHeight.js script in your HTML file.
<script src="https://code.jquery.com/jquery-3.6.0.min.js"></script>
<script src="https://cdnjs.cloudflare.com/ajax/libs/jquery.matchHeight/0.7.2/jquery.matchHeight-min.js"></script>
Then, add a class (e.g., match-height
) to the columns you want to match in height.
<div class="row">
<div class="col-xs-4 panel match-height" style="background-color: red">
<!-- some content -->
</div>
<div class="col-xs-4 panel match-height" style="background-color: yellow">
<!-- catz -->
<img width="100" height="100" src="https://lorempixel.com/100/100/cats/" alt="Cats">
</div>
<div class="col-xs-4 panel match-height" style="background-color: blue">
<!-- some more content -->
</div>
</div>
Finally, initialize the matchHeight()
function on the columns with the specified class.
$(document).ready(function() {
$('.match-height').matchHeight();
});
Engage with Us! 💬
We hope these solutions helped you make your Bootstrap columns all the same height! If you have any questions or alternative solutions, feel free to share them in the comments below. We love hearing from our readers and helping them out!
Also, don't forget to share this post with your fellow developers who might be facing the same issue. Let's spread the knowledge and make the web a better place! 🌐🚀
Happy coding! 😄👨💻👩💻
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
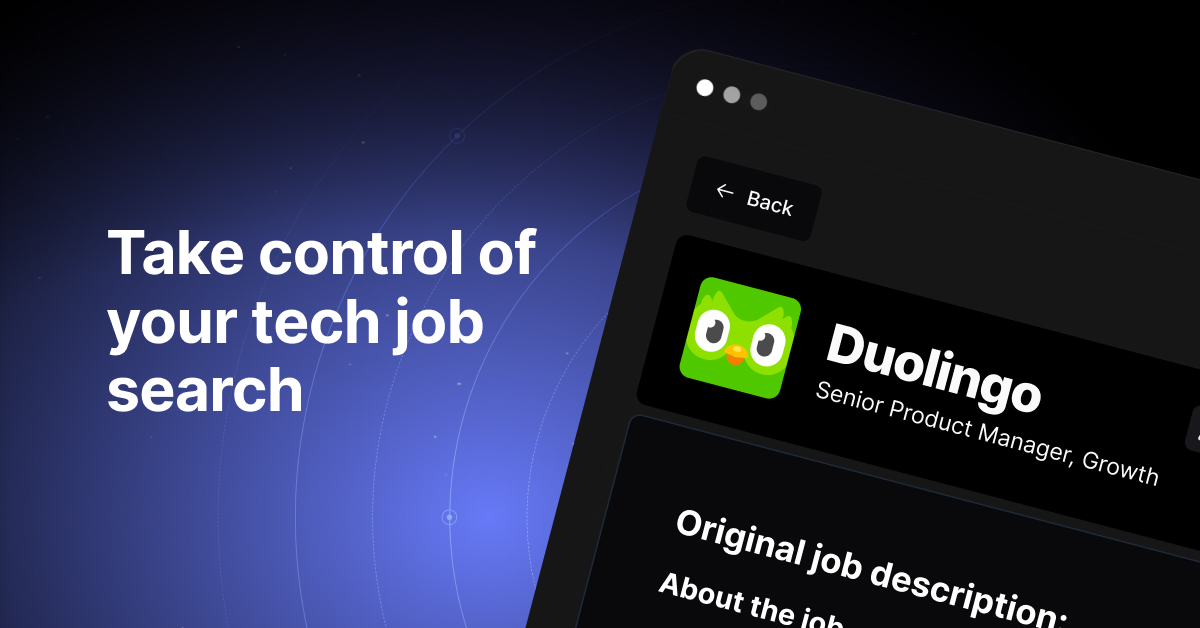