Swift: Test class type in switch statement
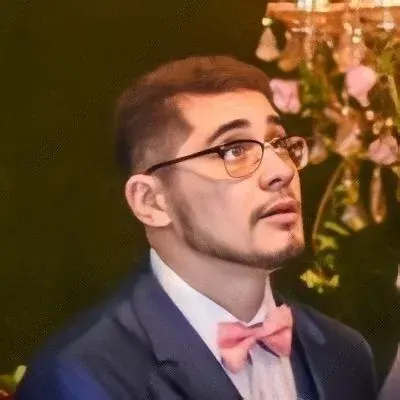
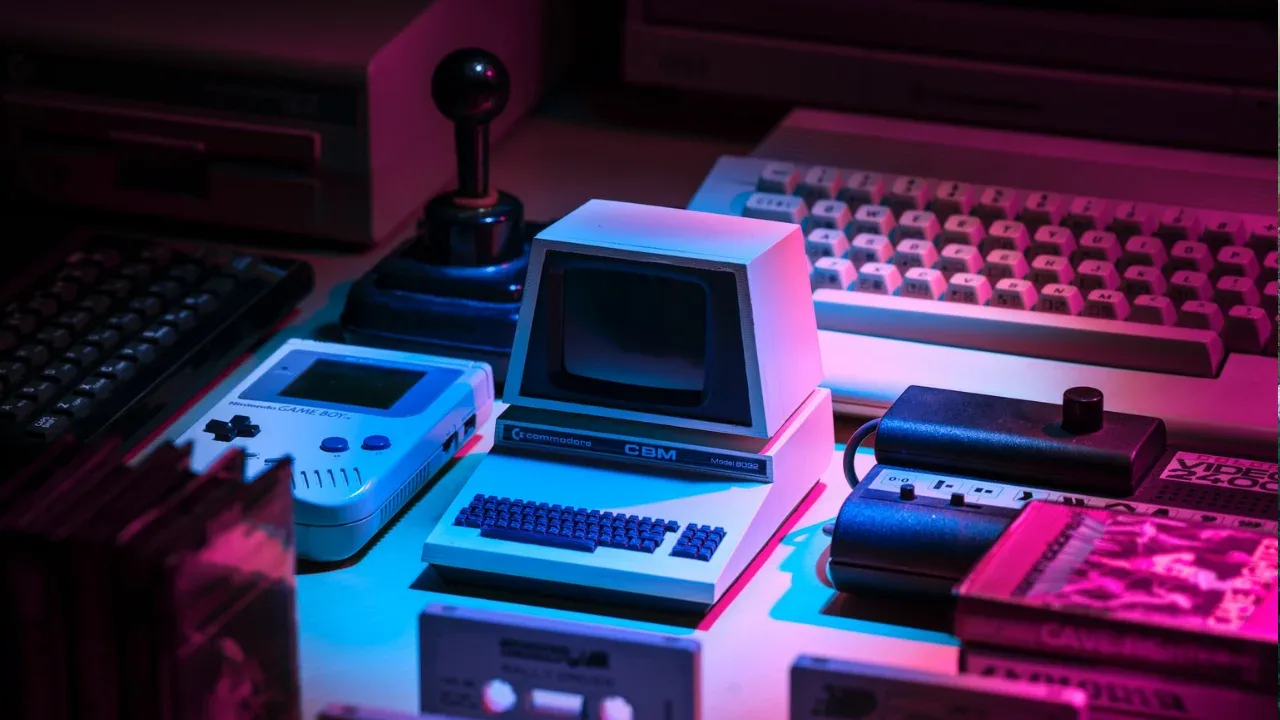
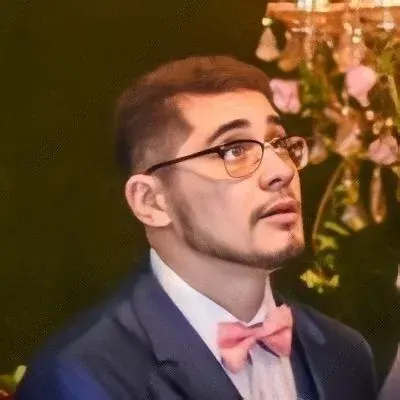
How to Test Class Type in Swift's Switch Statement ๐งช๐ค
Have you ever found yourself wondering how to incorporate class type checks into a switch
block in Swift? ๐ค If you have, you're not alone! This can be a common challenge for Swift developers when they want to perform different actions based on the type of an object. But fear not, because in this blog post, we will explore the best way to tackle this problem! ๐ช๐
The Challenge: Testing Class Type in a Switch Statement ๐๐ผ
In Swift, you can easily check the class type of an object using the is
keyword. However, incorporating this class type check into a switch
block might not be as straightforward. The question origination from a developer asking if it's possible to use the is
keyword within a switch
structure.
Let's consider a scenario where you have a base class called Animal
and two subclasses: Dog
and Cat
. You want to perform different actions based on the type of animal. Here's an example:
class Animal { }
class Dog: Animal { }
class Cat: Animal { }
let pet: Animal = Dog()
switch pet {
case is Dog:
print("It's a dog, let's play fetch!")
case is Cat:
print("It's a cat, let's cuddle!")
default:
print("It's neither a dog nor a cat, but still an animal!")
}
However, if you run this code, you'll notice that the switch
block only matches the base class Animal
, and not the specific subclasses Dog
or Cat
. ๐ข
The Solution: Use the 'as' Keyword to Cast Types! ๐๐ก
To work around this limitation, we need to cast the object to its specific type before using it in the switch
block. This can be achieved using the as
keyword:
let pet: Animal = Dog()
switch pet {
case is Dog:
let dog = pet as! Dog
print("It's a dog named \(dog.name), let's play fetch!")
case is Cat:
let cat = pet as! Cat
print("It's a cat named \(cat.name), let's cuddle!")
default:
print("It's neither a dog nor a cat, but still an animal!")
}
By first checking the class type using the is
keyword within the switch
block and then casting it using the as
keyword, we can access the specific properties and behaviors of each subclass! ๐
Take it a Step Further! ๐๐
Now that you know how to test class types in a switch
statement, you can explore even more advanced scenarios. For example, you can combine class type checks with pattern matching, where you can check for specific property values or even perform calculations. The possibilities are endless! ๐ซโจ
Conclusion: Test Like a Pro! ๐ฉโ๐ป๐จโ๐ป
Swift's switch
statement provides a powerful way to perform different actions based on class types. By using the as
keyword to cast objects before using them in a switch
block, we can easily identify and handle specific class types within our code.
So go ahead, test class types like a pro in your Swift projects! ๐ If you found this blog post helpful, be sure to share it with your fellow Swift developers. And if you have any other Swift-related questions or topics you'd like us to cover, let us know in the comments below! Happy coding! ๐ป๐ก
References
Did this blog post help you understand how to test class types in Swift's switch statement? Share your thoughts and experiences in the comments below! Let's learn together! ๐ฌ๐ค