TypeScript or JavaScript type casting
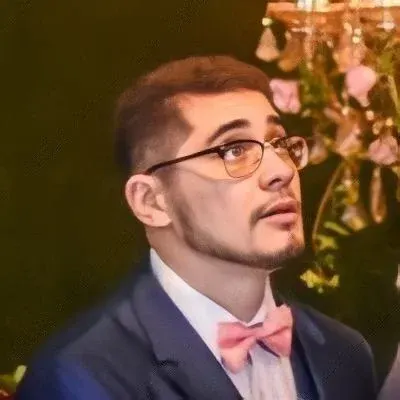
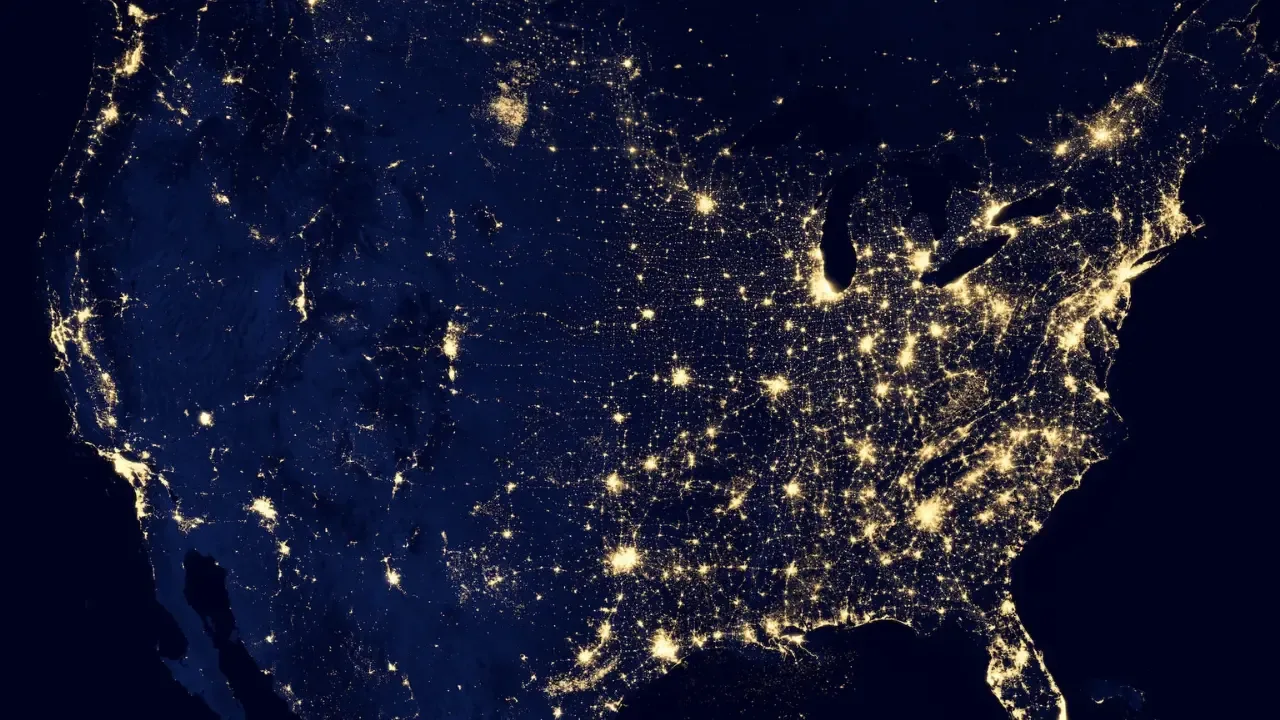
🎯 TypeScript or JavaScript Type Casting: Handling Typecasting from SymbolInfo to MarkerSymbolInfo 🖌️
Have you ever found yourself in a situation where you wanted to handle typecasting from SymbolInfo
to MarkerSymbolInfo
in TypeScript or JavaScript? 🤔 In this blog post, we'll explore common issues faced when dealing with typecasting and provide easy solutions. So, let's dive right in! 💪
The Scenario 📜
Consider the following TypeScript code snippet:
module Symbology {
export class SymbolFactory {
createStyle(symbolInfo: SymbolInfo): any {
if (symbolInfo == null) {
return null;
}
if (symbolInfo.symbolShapeType === "marker") {
return this.createMarkerStyle((MarkerSymbolInfo)symbolInfo);
}
}
createMarkerStyle(markerSymbol: MarkerSymbolInfo): any {
throw "createMarkerStyle not implemented";
}
}
}
In the given code, we have a SymbolFactory
class with two methods: createStyle
and createMarkerStyle
. The createStyle
method takes an argument of type SymbolInfo
. If the symbolShapeType
of the symbolInfo
object is "marker"
, we want to typecast it to MarkerSymbolInfo
and call the createMarkerStyle
method.
Now, let's see how we can handle this typecasting issue effectively. 👇
Handling Type Casting ✋
In TypeScript, we can handle typecasting using the as
operator, also known as an assertion. Here's how we can modify the code to handle typecasting from SymbolInfo
to MarkerSymbolInfo
:
module Symbology {
export class SymbolFactory {
createStyle(symbolInfo: SymbolInfo): any {
if (symbolInfo == null) {
return null;
}
if (symbolInfo.symbolShapeType === "marker") {
return this.createMarkerStyle(symbolInfo as MarkerSymbolInfo);
}
}
createMarkerStyle(markerSymbol: MarkerSymbolInfo): any {
throw "createMarkerStyle not implemented";
}
}
}
By using the as
keyword followed by the target type (MarkerSymbolInfo
in our case), we can perform the typecasting operation seamlessly.
Handling Typecasting in JavaScript 🔄
JavaScript, being a loosely typed language, doesn't feature strict typecasting. However, we can use some techniques to achieve typecasting-like behavior.
const symbolFactory = {
createStyle(symbolInfo) {
if (symbolInfo == null) {
return null;
}
if (symbolInfo.symbolShapeType === "marker") {
return this.createMarkerStyle(symbolInfo);
}
},
createMarkerStyle(markerSymbol) {
throw "createMarkerStyle not implemented";
}
};
In JavaScript, we can directly pass the symbolInfo
object to the createMarkerStyle
method, without the need for any explicit typecasting. JavaScript will dynamically determine the type based on the values present in the object.
Conclusion 🏁
Handling typecasting from SymbolInfo
to MarkerSymbolInfo
can be an essential part of your TypeScript or JavaScript project. By using the as
operator in TypeScript or leveraging JavaScript's dynamic typing, you can overcome these typecasting issues with ease. 🎉
So, next time you come across typecasting challenges, don't sweat it! Stay calm, code smart, and remember the techniques we discussed in this blog post.
Feel free to share your thoughts or ask any questions in the comments section below. Let's level up our typecasting game together! 💪🚀
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
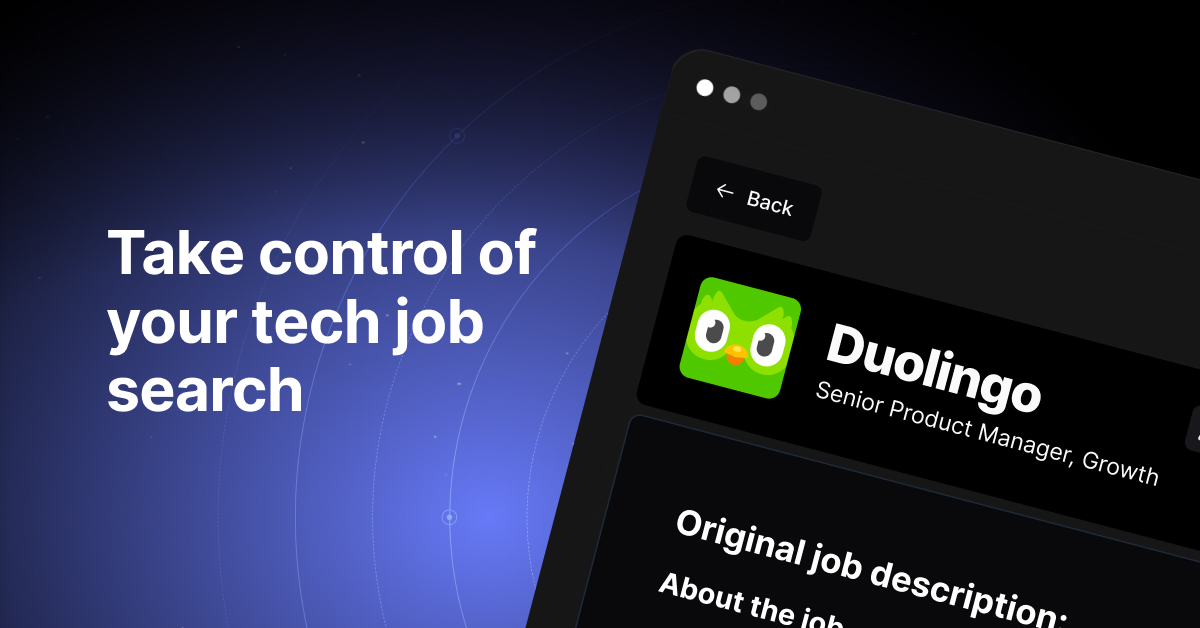