Why use double indirection? or Why use pointers to pointers?
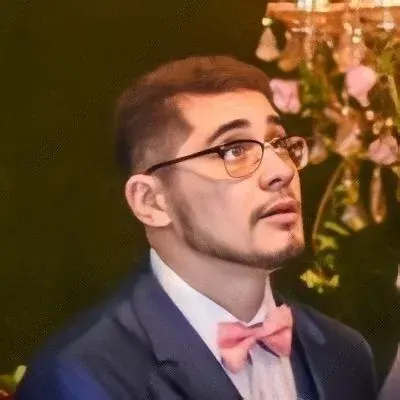
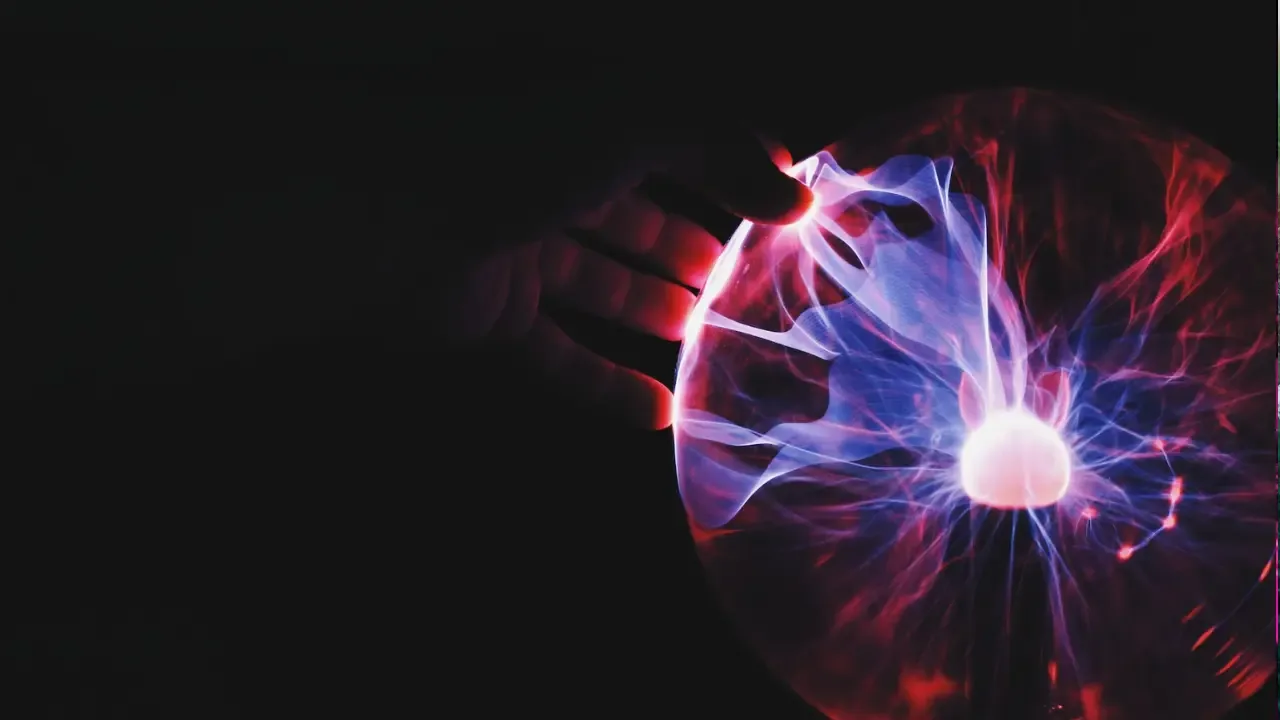
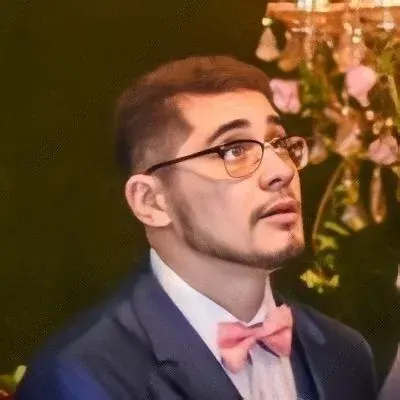
Double Indirection: Unraveling the Mystery of Pointers to Pointers 🎯
Are you confused about the concept of double indirection or pointers to pointers? 🤔 Don't worry, you're not alone! Many developers find this topic challenging, but fear not – we're here to unravel the mystery and help you understand why and when you should use double indirection in C. 🚀
Demystifying Double Indirection
Let's start with the basics. In C, a pointer is a variable that holds the memory address of another variable. It allows us to indirectly access and manipulate that variable. To get the value stored at a memory location, we use the dereference operator (*
), and to access the memory address itself, we use the address-of operator (&
).
So, what happens in the case of double indirection? Double indirection occurs when we have a pointer that points to another pointer. In simpler terms, it's a way to indirectly access the memory address of a pointer. 😮
The Power of Pointers to Pointers
Now comes the burning question: why would we ever need to use a pointer to a pointer? 🤷♀️ Let's explore a couple of scenarios where double indirection is particularly useful, starting with dynamic memory allocation.
Dynamic Memory Allocation
In C, we sometimes need to allocate memory dynamically at runtime using functions such as malloc()
or calloc()
. The memory returned by these functions is typically assigned to a pointer variable. But what if we want to allocate memory for an array of pointers or a two-dimensional array? This is where double indirection comes into play!
By using a pointer to a pointer, we can dynamically allocate memory for an array of pointers. Each element of this array can then be assigned to point to another block of dynamically allocated memory. This is often seen in scenarios like dynamically allocating a jagged array or implementing dynamic data structures like linked lists or trees. 😎
Modifying Pointers in Functions
Another common use case for double indirection is when we need to modify a pointer variable passed to a function. In C, function parameters are generally passed by value, meaning any modifications made to a parameter within a function won't affect the original variable. However, by passing a pointer to a pointer, we can modify the original pointer itself. 🔄
Consider a scenario where we want to update a pointer to point to a different memory address. By passing a pointer to a pointer as an argument, we can modify the original pointer and make it point to a new memory location. This can be handy when dynamically changing the size of an array or reassigning a pointer to a different variable or data structure.
Example: A Visual Guide
To illustrate the power of double indirection, let's walk through a simple example. Imagine we have an array of strings, and we want to sort them in alphabetical order. Here's how we can achieve this using double indirection:
void sortStrings(char** strings, int length) {
// Sort the strings using an appropriate algorithm
}
int main() {
char* names[] = { "Lucy", "Charlie", "Bob", "Alice" };
int length = sizeof(names) / sizeof(char*);
sortStrings(names, length);
// Now, the 'names' array is sorted in alphabetical order!
return 0;
}
In the sortStrings
function, we receive a pointer to a pointer (char**
) representing the array of strings. By manipulating this double indirection, we can sort the strings in place directly within the names
array.
This is just a simple example, but it demonstrates how useful double indirection can be in solving real-world problems, like sorting strings or managing dynamic memory allocations.
Your Turn to Level Up! 💪
Now that you have a better understanding of why and when to use double indirection, it's time to put your knowledge into action! Challenge yourself with the following tasks:
Practice Pointers to Pointers: Write simple programs to explore different use cases of double indirection. Experiment with dynamic memory allocation, modifying pointers in functions, and any other scenarios that intrigue you.
Ask for Help: If you still have questions or are facing difficulties, don't hesitate to ask for help from the vibrant developer community. Engage in discussions on forums, join coding communities, or seek guidance from experienced developers. Learning together is always more fun!
Remember, mastering pointers to pointers takes practice, patience, and perseverance. You're on the path to becoming a rockstar C developer! 🎸
So, why wait? Start unraveling the double indirection mystery today and level up your C programming skills! Share your experience and ask questions in the comments below, and don't forget to spread the knowledge by sharing this post with your fellow tech enthusiasts. 🌟✨
Happy coding! 💻🚀