Why malloc+memset is slower than calloc?
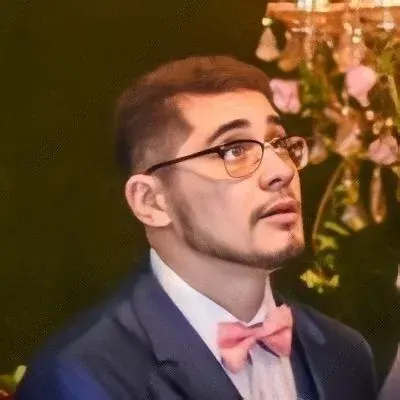
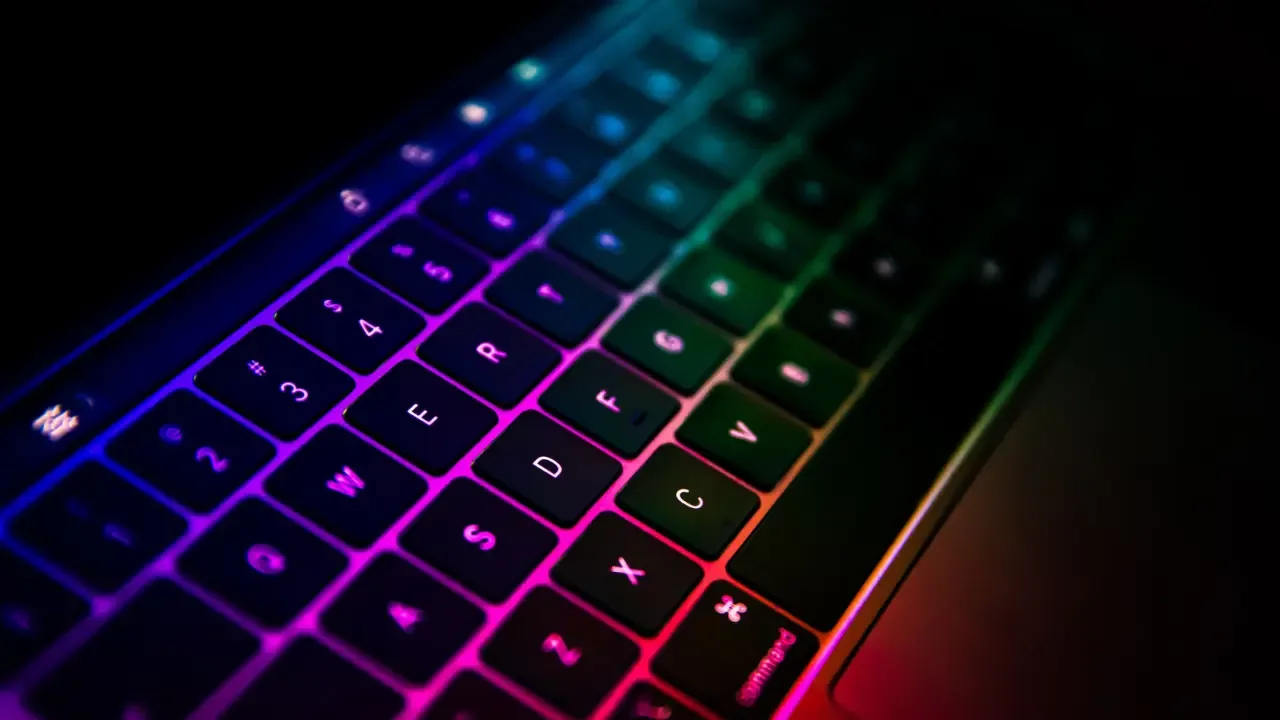
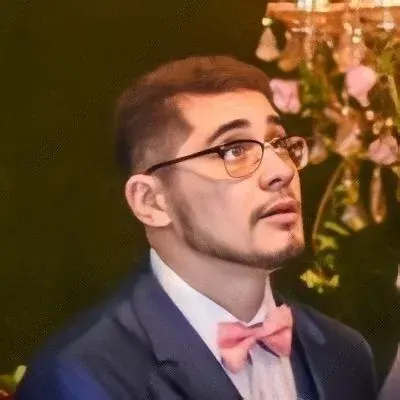
🔍 Why malloc+memset is slower than calloc?
Have you ever wondered why calloc
seems to be faster than malloc
followed by memset
? 🤔 In theory, they both allocate memory, but there seems to be a significant performance difference. Let's dive into it and discover why this happens and how calloc
manages to achieve it! 💡
📚 Understanding the Difference
First, let's understand the difference between calloc
and malloc
. When you use calloc
, it not only allocates the requested memory, but it also initializes the allocated memory to zero. On the other hand, malloc
just allocates the memory without any initialization.
In code terms, you can consider calloc
as a combination of malloc
and memset
🙌. So you might be tempted to think that if you manually allocate memory with malloc
and then initialize it with memset
, it should have similar performance, right? Well, turns out, it's not that simple!
💨 The Performance Difference
The benchmark code you provided shows a noticeable difference in performance between calloc
and malloc
+memset
. Let's analyze the code snippets and their outputs to understand why this happens.
Code 1 (calloc):
#include <stdio.h>
#include <stdlib.h>
#define BLOCK_SIZE 1024*1024*256
int main() {
int i = 0;
char *buf[10];
while (i < 10) {
buf[i] = (char*)calloc(1, BLOCK_SIZE);
i++;
}
}
Output of Code 1:
time ./a.out
real 0m0.287s
user 0m0.095s
sys 0m0.192s
Code 2 (malloc+memset):
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#define BLOCK_SIZE 1024*1024*256
int main() {
int i = 0;
char *buf[10];
while (i < 10) {
buf[i] = (char*)malloc(BLOCK_SIZE);
memset(buf[i], '\0', BLOCK_SIZE);
i++;
}
}
Output of Code 2:
time ./a.out
real 0m2.693s
user 0m0.973s
sys 0m1.721s
As you can see, the second code snippet with malloc
+memset
takes significantly more time to execute compared to the first one using calloc
.
💡 The Underlying Optimization
The reason behind this performance difference lies in the optimization strategies applied by the underlying system libraries. When you call calloc
, the system library can take advantage of certain optimizations, like memory mapping or pre-zeroing, to quickly allocate and initialize the memory in a more efficient way. On the other hand, when you separately call malloc
and memset
, the system library may not be able to apply the same level of optimization, leading to slower execution.
🔧 Possible Solutions
If you need to allocate memory and initialize it to zero, using calloc
is the most straightforward and efficient choice. However, if you really want to use malloc
followed by memset
for some reason, there are a few things you can try to improve the performance:
Allocate a larger memory block once instead of allocating smaller blocks multiple times. Less frequent allocations can improve efficiency.
Experiment with different allocation sizes. Some systems might have performance variations depending on the requested memory block size.
Consider using compiler-specific optimization flags. Certain compilers provide flags that can help optimize memory-related operations.
Profiling your code can identify any other bottlenecks that might be affecting performance. There could be other parts of your code impacting the overall execution time.
📣 Engage with Us!
We hope this article helps you understand why malloc
+memset
is slower than calloc
and provides some possible solutions to improve performance if you choose to use malloc
with memset
.
Do you have any other questions about memory allocation or performance optimization? Share your thoughts, experiences, or questions in the comments below! Let's have a vibrant discussion and learn from each other! 🚀