Why does the C preprocessor interpret the word "linux" as the constant "1"?
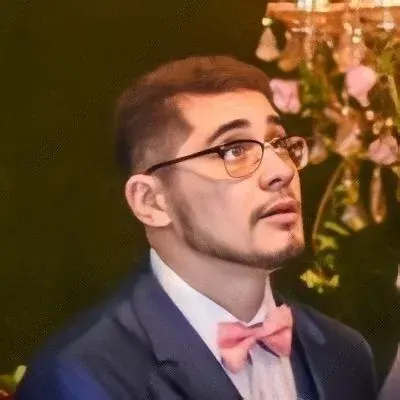
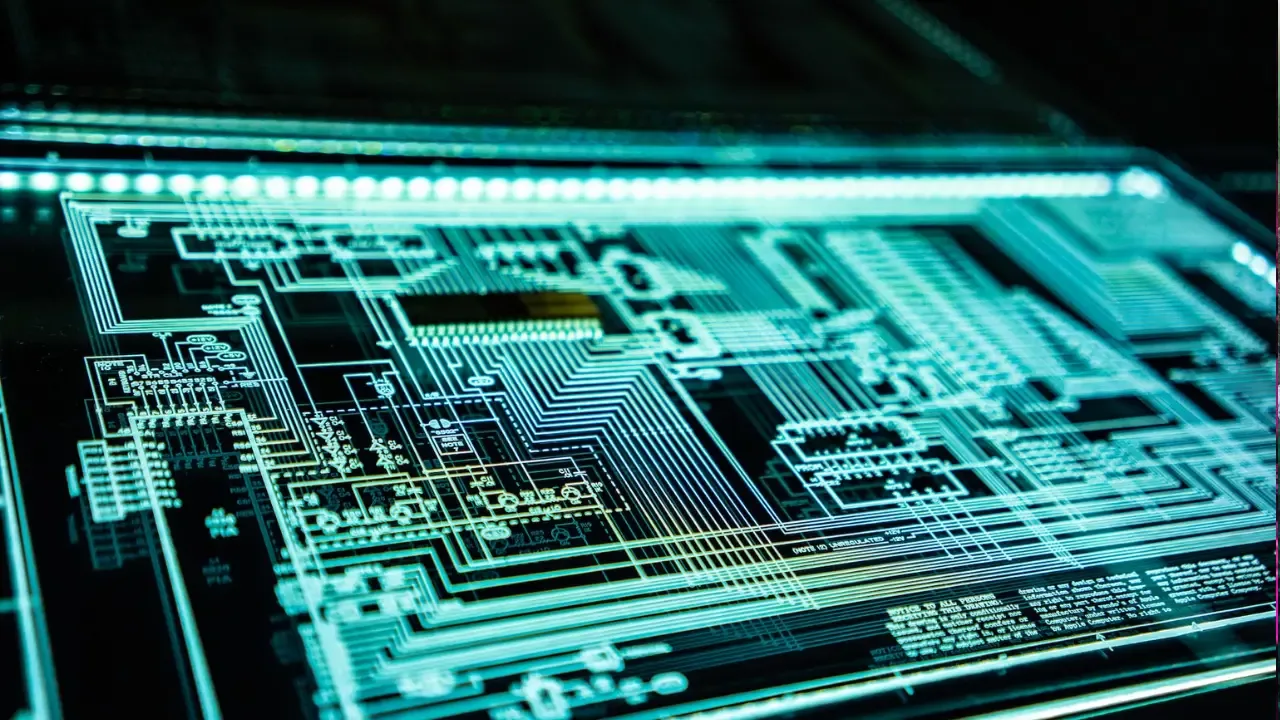
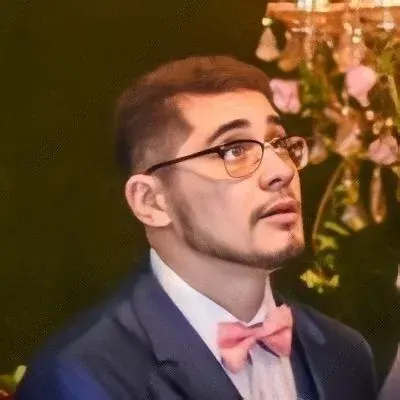
👋 Hey there! Welcome to my tech blog! Today, we're diving into the intriguing question 🤔: Why does the C preprocessor interpret the word "linux" as the constant "1"? Let's explore this common issue and find some easy solutions to help you out! 💪
You might have come across this unexpected behavior when using the C preprocessor in GCC. In the provided example, we have a C file called "test.c" where we declare an integer variable named "linux" and assign it a value of 5. 🖥️
But when we run the command gcc -E test.c
to perform the preprocessing stage, we encounter a puzzling result. The preprocessor replaces all occurrences of the word "linux" with the constant "1". 🤯 This leads to a compilation error when the code is compiled later on. 💥
Let's take a closer look at what's happening in our code snippet:
#include <stdio.h>
int main(void)
{
int linux = 5;
return 0;
}
After preprocessing, it becomes:
...
int main(void)
{
int 1 = 5;
return 0;
}
Now, you might be wondering, why does this substitution happen? Is there a predefined macro or something special about the word "linux"? 🤔
Well, here's the deal: in the C language, identifiers starting with a number are not allowed. However, the C preprocessor interprets any unrecognized identifier as having the value "1" by default. That's why our variable name "linux" gets replaced by "1". 🔄
To address this issue, you might be tempted to search for a #define linux
statement in the stdio.h
file or any other included files. But as mentioned in the context, there is no such definition present. So where is this coming from? 📜
It turns out that some compilers, like GCC, define various macros implicitly based on your environment. In this case, the GCC compiler defines a macro named "linux" by default, which maps to the value "1". This macro is meant to indicate Linux-based systems. 🐧
To overcome this problem, we have a couple of options:
1️⃣ Rename your variable: One straightforward solution is to choose a different name for your variable. Avoiding names that clash with predefined macros is always a good practice. Choose something meaningful and unique to your code. 🔄
2️⃣ Undefine the macro: If you really need to use the name "linux" for your variable, you can undefine the macro explicitly. Add the following line before your code:
#undef linux
This way, the preprocessor won't substitute your variable name with the predefined macro. ✋🔄
Now that you understand why the C preprocessor interprets "linux" as the constant "1" and learned some simple solutions, it's time for some hands-on practice! Try out these solutions and share your experience in the comments below. 👇
Remember, keeping an eye on potential clashes with predefined macros can save you from unexpected bugs and compilation errors. Stay vigilant and choose your identifiers wisely! 💡
That's it for today's blog post! If you found this information helpful, make sure to share it with your fellow developers. If you have any questions or want more content like this, reach out to me on social media or leave a comment. I'd love to hear from you! 🎉
Keep coding and stay curious! Until next time! 😄👋