Why does the arrow (->) operator in C exist?
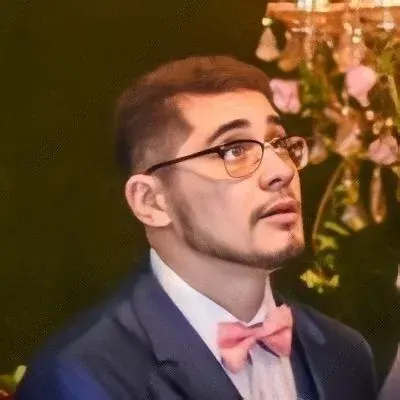
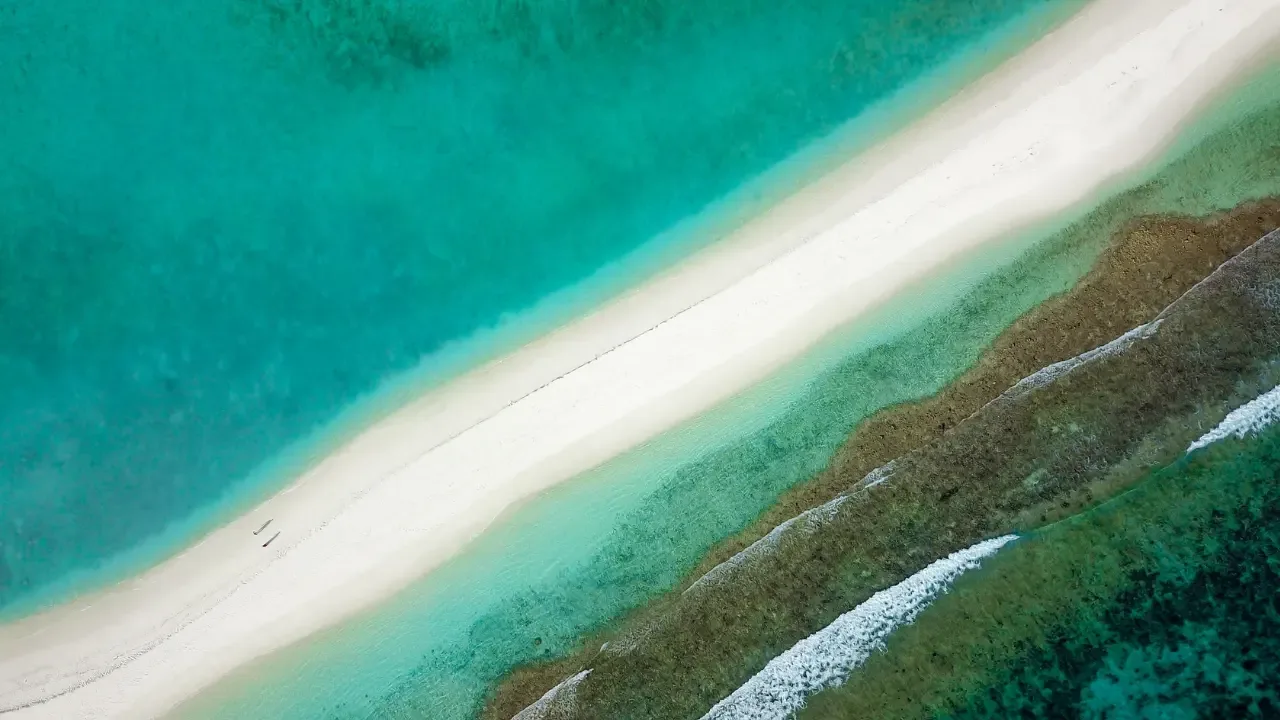
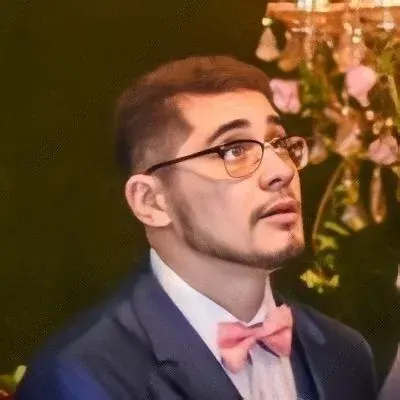
Why does the arrow (->) operator in C exist? 🤔
Have you ever wondered why C has the arrow operator (->) instead of just using the dot operator (.) for accessing struct members through a pointer? It may seem like a small syntax difference, but there is a good reason why the arrow operator exists in C.
Understanding the difference between the dot (.) and arrow (->) operators 🎯
To understand why the arrow operator is needed, we first need to understand the fundamental difference between the dot and arrow operators.
The dot operator (.) is used to access struct members directly. For example, if we have a struct called person
with a member name
, we could access it like this:
struct person {
char name[20];
};
struct person p;
strcpy(p.name, "John");
However, when we have a pointer to a struct, we cannot access its members directly using the dot operator because the pointer itself does not have any members. It only points to a memory location where the struct is stored.
Solving the problem with the arrow (->) operator 🏹
This is where the arrow operator comes into play. The arrow operator allows us to access struct members through a pointer. So, instead of writing p.name
, we use p->name
. It is a shorthand way of dereferencing the pointer and then accessing the member.
struct person *ptr = &p;
strcpy(ptr->name, "Mike");
The arrow operator provides a concise and intuitive syntax for accessing struct members through a pointer.
The design decision behind the arrow operator 🎨
Now, you might be wondering why the language creators decided to introduce the arrow operator instead of automatically dereferencing the pointer when using the dot operator.
The key reason behind this design decision is clarity and avoiding ambiguity. By explicitly using the arrow operator, the code becomes more readable and self-documenting. It clearly indicates that we are accessing a struct member through a pointer.
If the dot operator automatically dereferenced the pointer, it would introduce ambiguity in cases where the struct itself has a member that happens to be a pointer. Consider the following example:
struct person {
char *name;
};
struct person p;
p.name = "John";
If the dot operator automatically dereferenced the pointer, accessing the name member would require an extra step: (*p.name)
, which is not as intuitive as p->name
.
Conclusion and call-to-action 📝
So, the arrow operator exists in C to provide a clear and concise syntax for accessing struct members through a pointer. It helps to differentiate between accessing struct members and accessing the members of a struct pointed to by a pointer.
Next time you come across the arrow operator in C, remember that it serves a purpose and makes your code more readable.
Do you have any other questions about C operators or any other programming languages? Let me know in the comments below! Let's explore the intricacies of coding together. 💪👩💻👨💻