Why does rand() + rand() produce negative numbers?
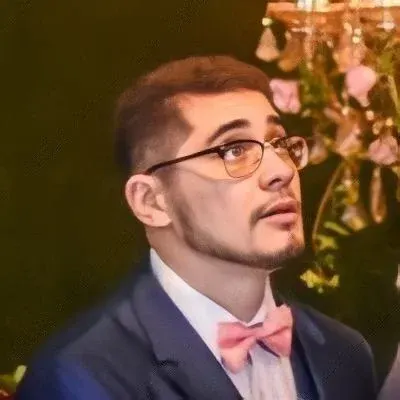
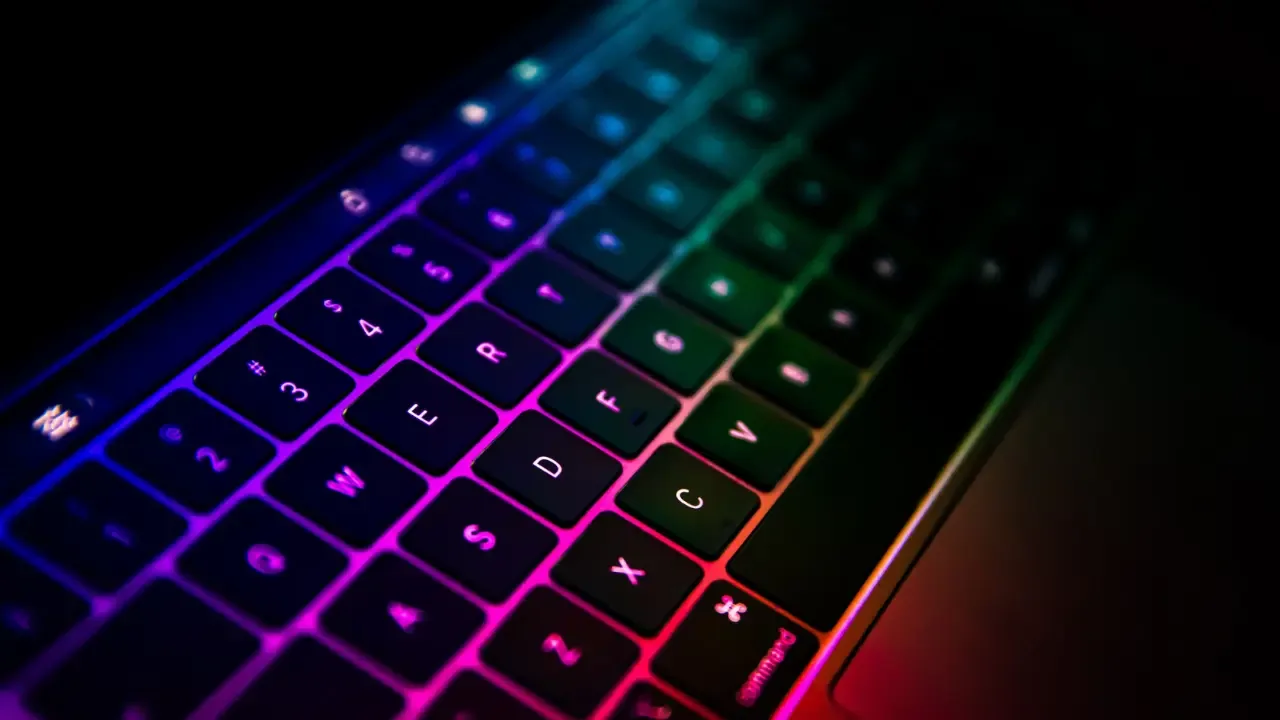
Title: ๐ฅ Why does rand() + rand() produce negative numbers? ๐ฅ
Intro:
Hey there, tech enthusiasts! Have you ever wondered why the rand() + rand()
combination sometimes produces negative numbers? ๐ค Well, in this blog post, we're going to dig deep into this common issue and provide you with easy solutions. Buckle up because it's going to be a wild ride! ๐
The Observation:
Recently, while tinkering with the rand()
library function, I stumbled upon an interesting behavior. When I called rand()
just once within a loop, it consistently spat out positive numbers. ๐ฎ Let's take a look at an example:
for (i = 0; i < 100; i++) {
printf("%d\n", rand());
}
Surprisingly, all the numbers generated were positive. Life was somewhat blissful at this moment, but then... curiosity struck! ๐ก
The Unexpected Twist:
Being a brave explorer, I decided to level up the randomness by adding two rand()
calls together like this:
for (i = 0; i < 100; i++) {
printf("%d = %d\n", rand(), (rand() + rand()));
}
And boom! ๐ฑ That's when I noticed that the generated numbers now had a sprinkle of negativity. What just happened? Why do we have negative numbers all of a sudden? Let's dive into the mysteries of randomness together to uncover the truth!
Understanding the Issue:
To understand this behavior, we need to take a step back and explore the inner workings of the rand()
function. ๐ง
The rand()
function generates random numbers based on a seed value. Each time we call it, it returns a pseudorandom number derived from the seed. By default, if we don't provide a seed explicitly, the srand(time(NULL))
function is often used to initialize the seed based on the current time.
However, there's a catch with the seed initialization. When we call srand(time(NULL))
once, it sets the seed for the subsequent rand()
calls. But guess what? The rand()
function doesn't rely on just one seedโit uses two! ๐ฒ
Yes, you heard it right! In some implementations of the C standard library, rand()
internally uses two seeds and combines them to generate numbers. When we call rand()
only once, it's using one seed, but when we call it twice (like in rand() + rand()
), it's using both seeds together.
The Seed Combination Magic:
Up until now, we've learned that rand()
uses two seeds. But what happens when we add two rand()
calls together? ๐ค It turns out that the combination of these two seeds has an interesting side effectโit introduces a bias towards negative numbers.
Here's a simplified explanation: The sum of two positive random numbers can naturally be positive. But when one number is negative, the sum has a higher chance of being negative. Think of it as a seesaw where positive numbers try to balance the negative ones, but ultimately fail. ๐ข
Solutions and Workarounds: Now that we understand the issue, we can explore possible solutions and workarounds:
Use unsigned integer arithmetic: By working with unsigned integers (
unsigned int
), we eliminate the possibility of dealing with negative numbers altogether. That's a simple and effective workaround! ๐
for (i = 0; i < 100; i++) {
printf("%u = %u\n", rand(), (rand() + rand()));
}
Subtract half the range: Another way to mitigate negative numbers is by subtracting half the range of the random values. This approach shifts the generated numbers towards the positive side. ๐
for (i = 0; i < 100; i++) {
printf("%d = %d\n", rand() - RAND_MAX / 2, (rand() + rand()) - 2 * (RAND_MAX / 2));
}
Remember to choose a solution based on your specific problem and requirements.
The Call-to-Action:
Congratulations, explorer! You've successfully unraveled the mystery behind why rand() + rand()
sometimes produces negative numbers. Now it's time to apply this newfound knowledge in your coding adventures. Experiment, tinker, and share your experiences and questions in the comments section below! Let's keep the discussion going and learn from each other. ๐
Until next time, happy coding! ๐๐ฉโ๐ป๐จโ๐ป
Note: The behavior of the rand()
function can vary across different implementations and programming languages. This blog post focuses primarily on the behavior observed in C's rand()
function.
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
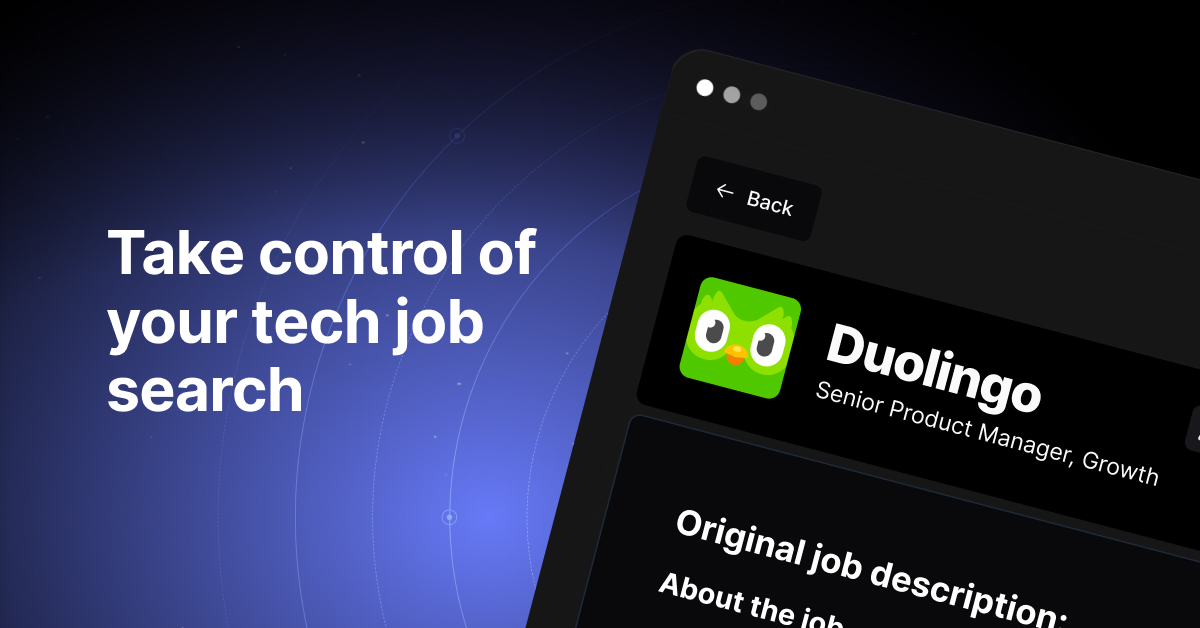