Why does a function with no parameters (compared to the actual function definition) compile?
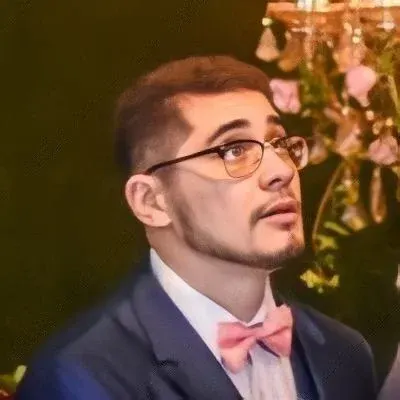
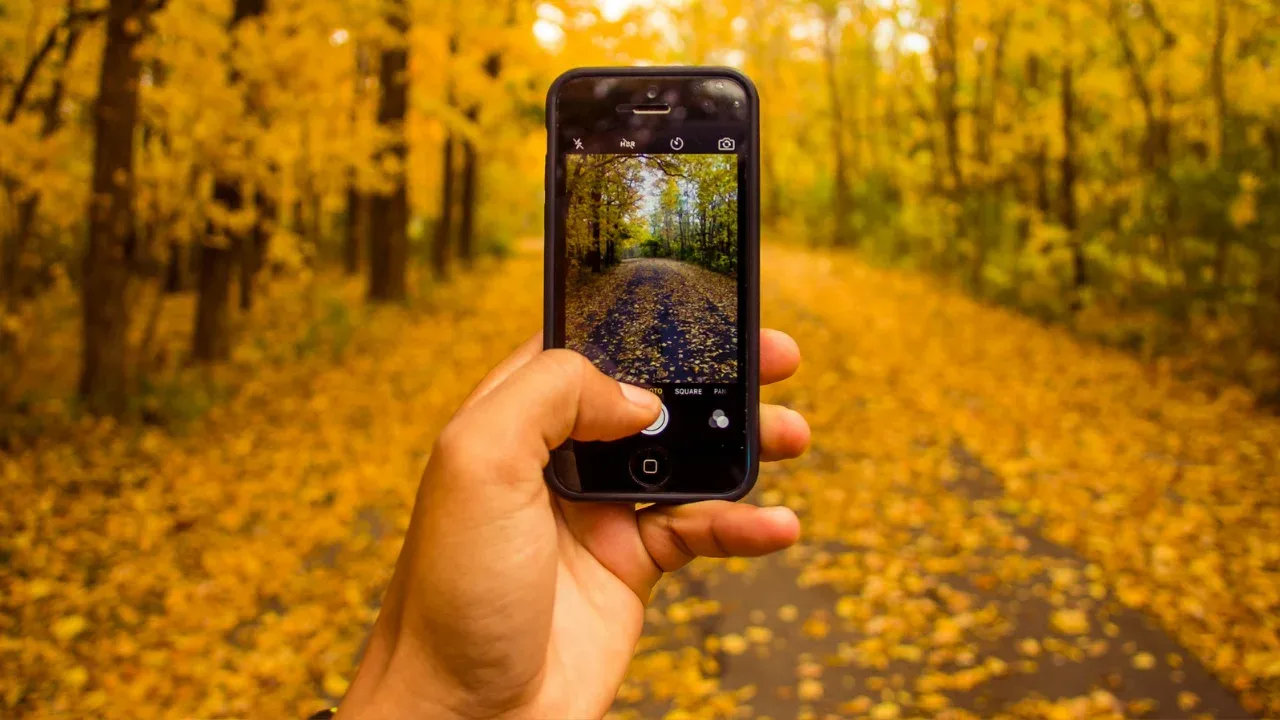
๐ค Understanding Function Compilation with No Parameters
Do you sometimes come across code that seems confusing or even incorrect, but it still compiles without any errors? ๐คทโโ๏ธ Today, we'll dive into a perplexing C code snippet and unravel the mystery behind its successful compilation. ๐
The Code Puzzle ๐งฉ
Here's the intriguing code that caught our attention:
#include <stdio.h>
int func();
int func(param)
{
return param;
}
int main()
{
int bla = func(10);
printf("%d", bla);
}
Now, let's tackle the two puzzling points mentioned in the context ๐
Point 1: No Parameters in Function Prototype ๐คจ
The function prototype of func
is defined without any parameters, as seen here:
int func();
However, in the subsequent function definition, the parameter param
is present:
int func(param)
{
return param;
}
At first glance, this seems contradictory. How can a function be declared without any parameters but then defined with one? ๐ค
The Hidden Power of Old-Style Function Definitions ๐ช
To understand why this code snippet compiles successfully, we need to take a step back and explore the history of C. In older versions of C (pre-ANSI C), parameter types were not explicitly required in function declarations. In these cases, the compiler implicitly assumed that the parameter type was int
.
So, in our code example, when func()
is declared without parameters, it's actually a shorthand way of declaring func(int)
. When the corresponding function definition is encountered, the compiler is already aware that the parameter type is int
. Hence, no type mismatch or compilation error occurs. ๐
Point 2: Lack of Type in Parameter Definition ๐ถ
Another peculiar aspect of the code snippet is the absence of a type for the param
parameter in the function definition:
int func(param) // Missing type here
{
return param;
}
When we define a parameter without specifying its type, the compiler again assumes the default type of int
. This assumption aligns with the old-style C conventions that allow functions to be defined without explicit type declarations for parameters.
Why Does This Code Compile? ๐ฏ
Now it's time to answer the burning question: why does this code actually compile and work as expected? ๐
The code compiles successfully because the old-style function declaration and definition are consistent with each other. The implicit assumption of the int
type for both the parameter in the definition and the empty parameter declaration in the prototype leads to a harmonious compilation process.
To further reassure ourselves, we ran this code snippet through multiple compilers, and they all accepted it without any errors. ๐
Best Practices: Clarity and Compatibility โ
Although the code we explored compiles successfully due to historical C compatibility, it's highly recommended to follow current best practices to write clear, modern, and maintainable code.
Here are a few tips to ensure your code is compatible and less likely to confuse developers:
Use explicit function prototypes: Instead of relying on default assumptions, clearly declare function prototypes with their corresponding parameter types.
Specify parameter types in function definitions: Clearly define the types of function parameters to prevent any ambiguity or confusion.
Leverage modern compilers: Taking advantage of robust modern compilers helps detect potential issues and ensures better compatibility with the latest programming language standards.
By adopting these practices, you'll enhance code readability, simplify maintenance, and minimize the risk of compatibility issues across different compilers and programming environments. ๐
Join the Conversation! ๐ฌ
Have you ever stumbled upon code that defies expectations by compiling against all odds? Share your experiences in the comments below! Let's unravel more mysteries together and celebrate the wonders of coding. ๐
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
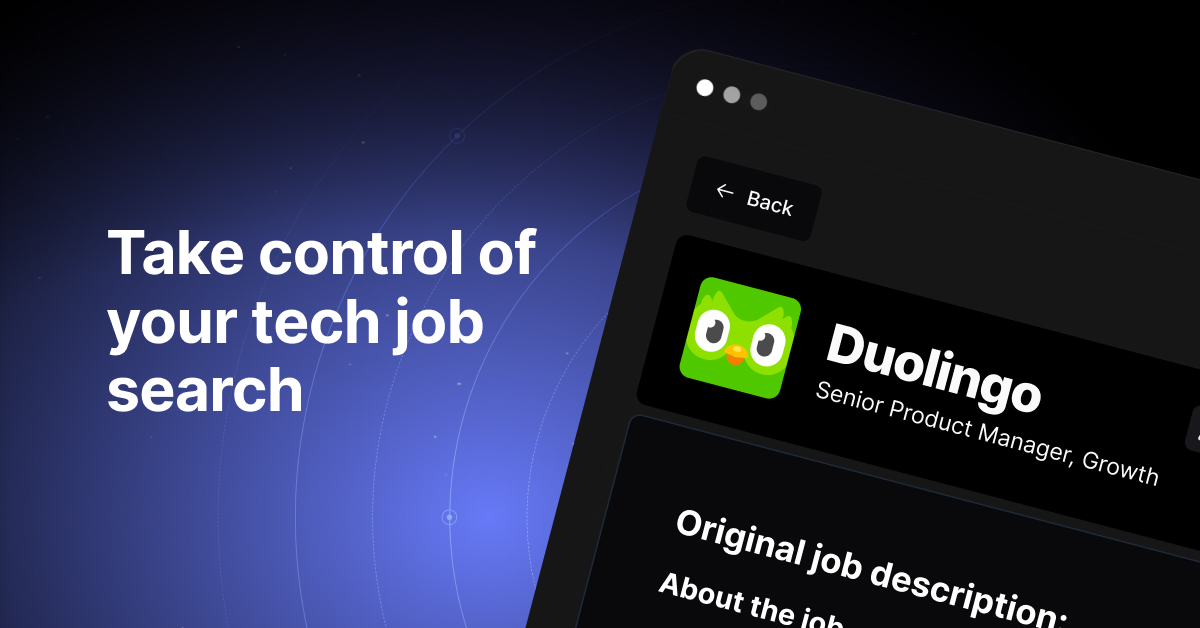