Why do you have to link the math library in C?
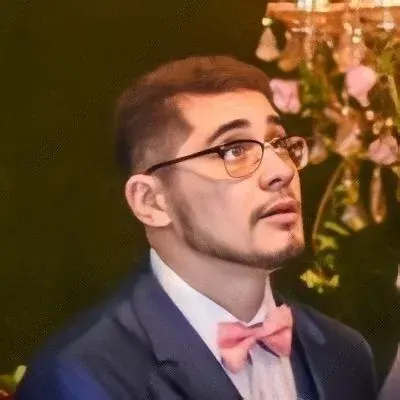
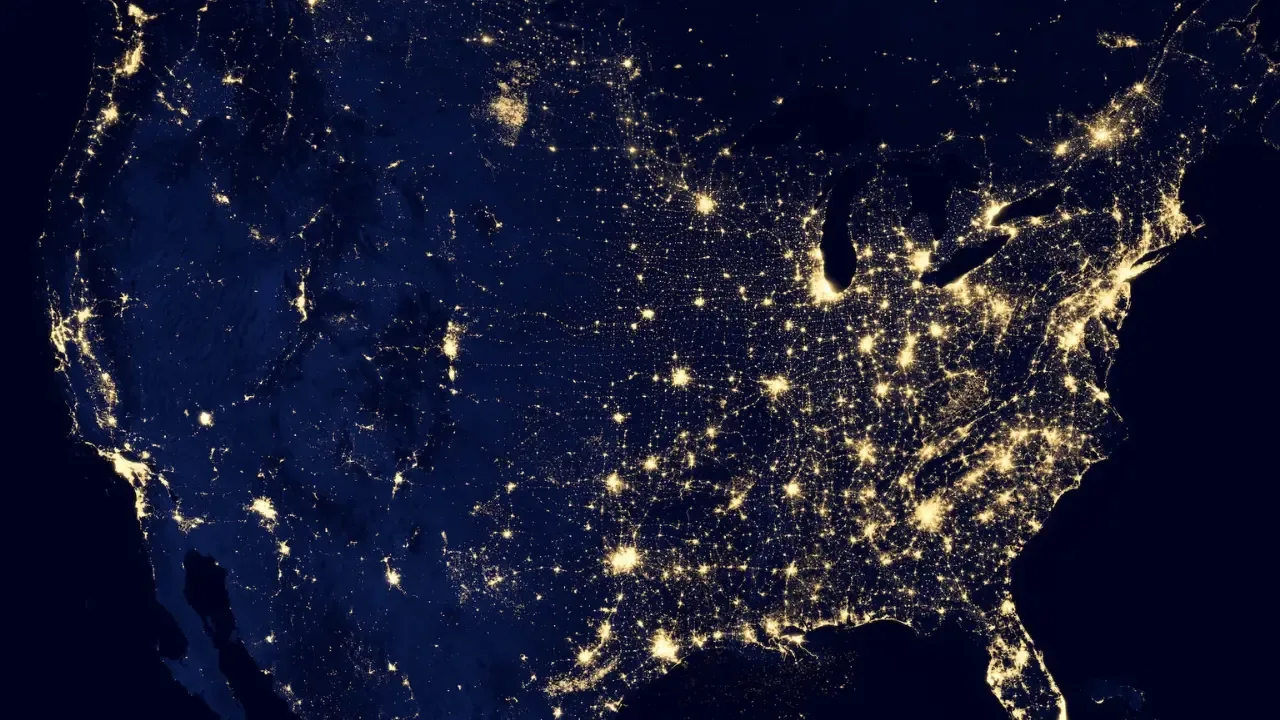
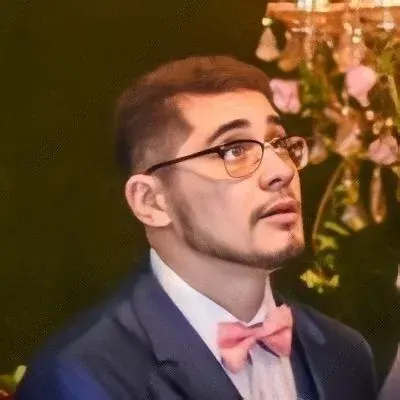
Why do you have to link the math library in C? 🤔
So, you're working on a C program and you encounter this peculiar situation. When you include <stdlib.h>
or <stdio.h>
in your code, you don't have to worry about linking them during compilation. But, when it comes to <math.h>
, you suddenly need to include a link to the math library using -lm
with GCC, just like this:
gcc test.c -o test -lm
Now, you're wondering: what's the reason behind this? Why do you have to explicitly link the math library while the other libraries are automatically linked?
The Math Library Mystery Unveiled 🎩
The thing is, the math library in C contains a variety of mathematical functions like sqrt()
, sin()
, cos()
, and many more. These functions are often used in programs that require complex mathematical computations.
Unlike the standard libraries like <stdlib.h>
and <stdio.h>
, which are often needed by almost all C programs, the math library is only needed for programs that utilize these specialized mathematical functions. Therefore, it is not included by default during the linking process.
By linking the math library explicitly using the -lm
option, you are telling the compiler to search for the necessary code for these mathematical functions in the math library and include them in your program.
Simple Solutions to Linking the Math Library ✨
Now that we understand why we need to link the math library, let's explore a couple of straightforward solutions to successfully link it in your program:
Option 1: Modify Your Compilation Command
When compiling your C program, include the -lm
option along with the other relevant libraries and source files.
For example:
gcc test.c -o test -lm
This ensures that the math library gets linked during the compilation process, enabling your program to access all the mathematical functions it needs.
Option 2: Include the Linking Directive in Your Code
Alternatively, you can also include the linking directive #pragma comment(lib, "m")
in your code, right after the #include <math.h>
line.
Here's an example:
#include <math.h>
#pragma comment(lib, "m")
// Rest of your code
This directive tells the compiler to automatically link the math library without needing the -lm
option during compilation.
Engage and Share Your Insights ✍️
Now that you understand the reasoning behind linking the math library in C, why not share your newly acquired knowledge with others? 👥
Feel free to leave a comment below and let us know if you found this blog post helpful. You can also share this post with your friends and fellow developers who may have been puzzled by this issue.
Keep coding and stay curious! 🚀