Why do I get a segmentation fault when writing to a "char *s" initialized with a string literal, but not "char s[]"?
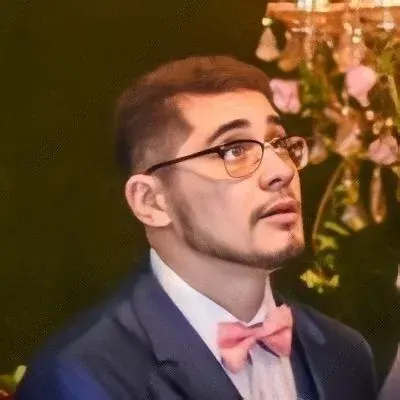
![Cover Image for Why do I get a segmentation fault when writing to a "char *s" initialized with a string literal, but not "char s[]"?](https://images.ctfassets.net/4jrcdh2kutbq/1WcuxFyh8PuMmD6vqX07qH/d36c7ea7d37475dfaccf64149a352875/Untitled_design__16_.webp?w=3840&q=75)
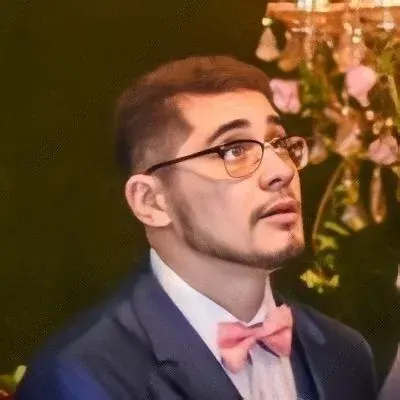
What's With the Segmentation Fault: "char *s" vs "char s[]"?
āš± Oh no! You just ran into a dreaded segmentation fault error when trying to modify a string. Don't worry, you're not alone. This error can be quite sneaky, but fear not! šŖ In this blog post, we'll unravel the mystery behind why a segmentation fault occurs when trying to modify a char *s
initialized with a string literal, while char s[]
works like a charm. Along the way, we'll dive into some common issues, provide easy solutions, and ensure you leave with a clearer understanding. Let's get started! š
Understanding the Problem š¤
The code snippet provided sets the stage for our exploration. It consists of two examples: one using char *str
and the other using char str[]
. The first example triggers a segmentation fault, while the second one runs without a hitch. Let's dissect why this happens.
The Pointer Predicament š
In the first example, char *str
is declared as a pointer and initialized with a string literal, "string"
. A string literal is a sequence of characters enclosed in double quotes. Essentially, char *str
points to the memory location where the string literal resides.
But here's where things get tricky. š¬ In most programming languages, string literals are considered read-only and stored in a special area of memory known as the read-only data segment or text segment. When we try to modify a string literal using str[0]
or *str
, we're attempting to change memory that is off-limits, which is why a segmentation fault occurs. Think of it as an invisible barrier preventing you from altering the string.
Array Advantage š¹
In contrast, the second example char str[]
declares an actual character array. When the string literal, "string"
, is assigned to char str[]
, a new block of memory is created, and the literal is copied into it. Since arrays in C and C++ are mutable, you can freely modify the elements of str[]
without causing any harm.
Easy Solutions š ļø
Now that we understand the root cause of the segmentation fault, let's explore a couple of solutions to help you out of this conundrum.
Option 1: Pre-Allocate Known Maximum Length š
If you know the maximum length of the string literal in advance, you can allocate the appropriate memory using char str[MAX_LENGTH]
instead of char *str
. Remember, in this case, str
is an array, not a pointer. By explicitly allocating sufficient memory, you avoid modifying read-only memory and eliminate the segmentation fault risk.
Option 2: Dynamically Allocate Memory š¦
Alternatively, if you don't know the maximum length ahead of time or need a flexible solution, you can dynamically allocate memory for char *str
using the malloc
or calloc
functions. This allows you to create mutable memory space for your string and modify it without triggering a segmentation fault. Just remember to free the memory when you're done to prevent memory leaks.
char *str = malloc(MAX_LENGTH * sizeof(char)); // Allocate memory
strcpy(str, "string"); // Copy the string literal
str[0] = 'z'; // Modify the string
printf("%s\n", str); // Print modified string
free(str); // Release the allocated memory
Engage with Us! āļø
Congratulations! You've successfully navigated the treacherous world of segmentation faults when dealing with char *s
and char s[]
. But don't stop now! We'd love to hear your thoughts and experiences. Have you encountered other common pitfalls related to string manipulation? Did you find this blog post helpful? Leave a comment below and let's start an exciting conversation! š
Remember to like, share, and subscribe to our newsletter to stay up-to-date with the latest tech tips and tricks. Help us spread the knowledge and empower fellow developers in their coding adventures. Together, we can conquer any bug that stands in our way! šš»
Happy coding! š©āš»šØāš»