What is the >>>= operator in C?
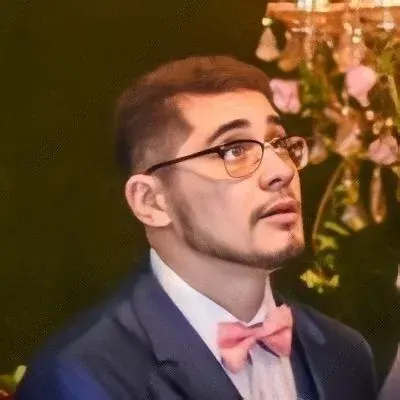
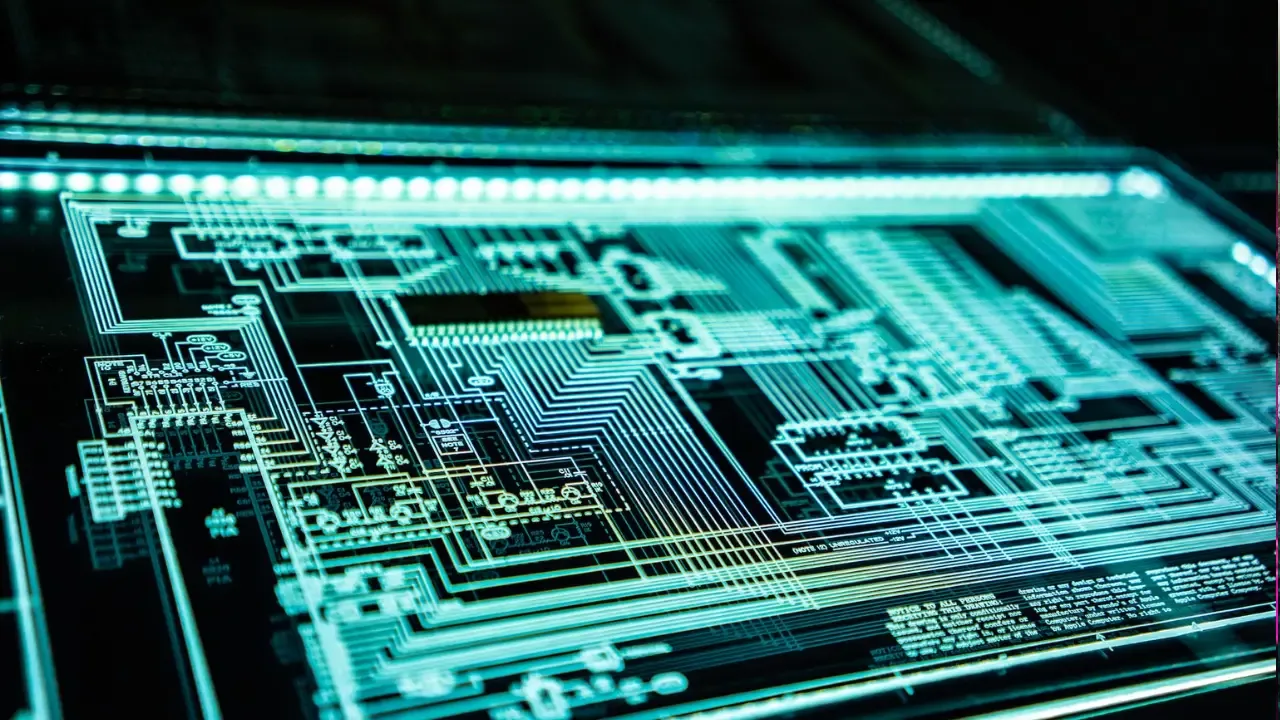
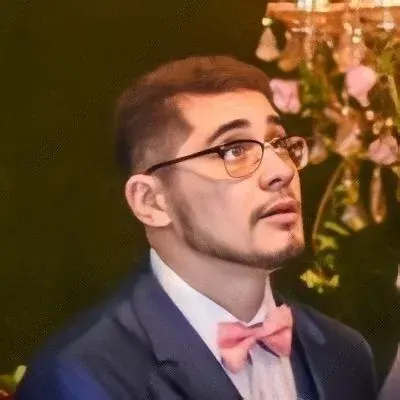
š Blog Post: Unlocking the Mystery of the >>>=
Operator in C
Introduction
š¤ Have you ever come across strange symbols or operators in C code that made you scratch your head? One such example is the >>>=
operator, which might puzzle even seasoned programmers. In this blog post, we'll unravel the mystery behind this operator and shed some light on the peculiar 1P1
literal. So, buckle up and prepare to dive into the world of unusual C syntax!
Understanding the Code
š Let's start by analyzing the code snippet provided by our curious colleague:
#include <stdio.h>
int main()
{
int a[2] = { 10, 1 };
while( a[ 0xFULL ? '\0' : -1 : >>>= a < : !!0X.1P1 ] )
printf("?");
return 0;
}
Decoding the Code
š¢ Before we tackle the >>>=
operator, let's decipher the 1P1
literal. In C, literals are representations of fixed values, such as numbers or characters. The peculiar 1P1
literal represents the floating-point number 1.0
, using hexadecimal notation. The P
in this context denotes the exponent, indicating 1
multiplied by 16
raised to the power of 1
, resulting in 16.0
.
š Now, let's move on to the >>>=
operator. In C, the >>>=
operator is not a standard operator; rather, it is a mixture of the >>
(right shift) and =
(assignment) operators.
š The >>
operator performs a right shift operation on its left operand by the number of bits specified on the right. For example, a >> 1
shifts all bits of a
to the right by one position. In our case, the >>>
operator seems to be an extension, but it does not provide any extra functionality; rather, it adds complexity by confusing the reader.
āļø Simplifying the Code
ā
To make the code more readable and avoid confusion, we can replace the >>>=
operator with the equivalent >>=
(right shift and assignment) operator. The modified code would look like this:
#include <stdio.h>
int main()
{
int a[2] = { 10, 1 };
while( a[0xFULL ? '\0' : -1] >>= a < : !!0X.1P1 ]
printf("?");
return 0;
}
š By replacing the >>>=
operator with >>=
, we ensure that the code is easier to understand for other developers who might read it.
Solving the Mystery
š” Now that we have simplified the code, it's time to understand its functionality. In the original code snippet, the while
loop's condition is evaluating an expression involving array indexing and bitwise operations. Let's break it down step by step:
a[0xFULL ? '\0' : -1]
performs an array indexing operation ona
with either a non-existent index (in case0xFULL
evaluates to a non-zero value) or-1
(if0xFULL
evaluates to zero). As a result, this expression always accesses an element either before the start of the array or one that is out of bounds.>>=
performs a right shift operation on the value retrieved froma
. The right shift value is obtained by comparinga
with0
. Ifa
is zero, the result is1
, and ifa
is non-zero, the result is0
. This effectively acts as a toggle, shiftinga
by1
bit to the right if it was previously zero, and by0
bits if it was previously non-zero.!!0X.1P1
is equivalent to1
, since it performs a double-negation operation on0X.1P1
(which we previously decoded as16.0
).
š” The output of the code is "?"
because the printf
statement inside the loop is executed every time a
is non-zero (i.e., an element of a
is non-zero after shifting).
Conclusion
š Congratulations! You have successfully decoded the meaning behind the mysterious >>>=
operator and the peculiar 1P1
literal in the given C code. By simplifying the code and breaking down its functionality, we made it easier to understand. Remember, in C, it's always beneficial to write code that is clear and straightforward to prevent confusion among fellow developers.
š£ We hope you found this blog post helpful for unraveling perplexing C code snippets. If you have any more questions or similar coding puzzles, let us know in the comments below. Let's keep the discussion going!