What is the difference between char * const and const char *?
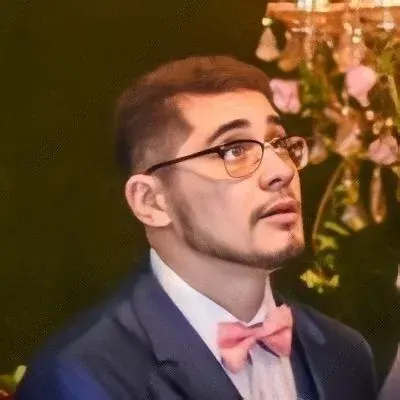
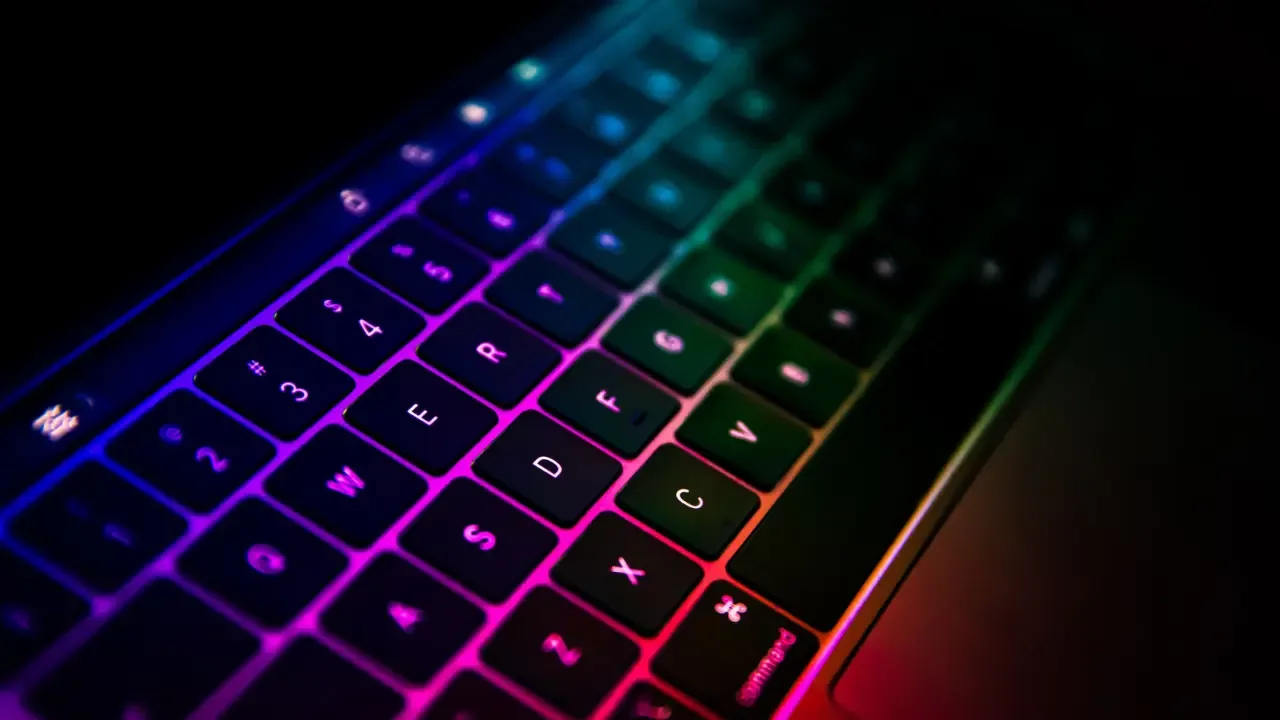
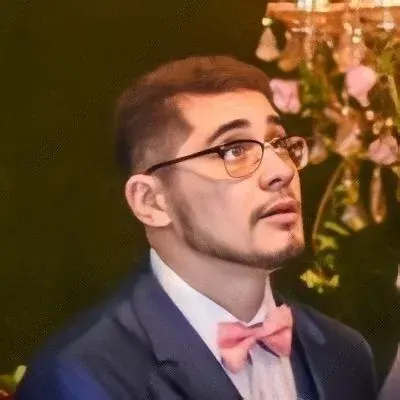
š Blog Post: Understanding the Difference Between char * const
and const char *
š Hey tech enthusiasts! Welcome back to my blog. Today, let's talk about a common source of confusion in C and C++ programming - the difference between char * const
and const char *
. š¤
Let's dive right in and break it down for you! šŖ
š¤ What do these declarations mean?
char * const
and const char *
may look similar, but they have different meanings.
š char * const
means that the pointer itself (char *
) is constant, but the data it points to can be changed. This means you cannot reassign the pointer to point to a different memory location, but you can modify the data it points to. š
š const char *
means that the data being pointed to is constant, but the pointer itself can be changed. In this case, you can reassign the pointer to point to a different memory location, but you cannot modify the data it points to. š«
ā” Common Issues and Problems
š One common confusion arises when trying to understand which one to use when declaring function parameters. Let's take a look at an example:
void functionName(const char * str) {
// Do something with str
}
In this case, const char *
is used to indicate that the function will not modify the content of str
. It's a good practice to use this declaration when you don't intend to modify the string within the function. š
āāļø
Another tricky situation is when you want to create a constant pointer to a string:
const char * const str = "Hello, World!";
Here, str
is a constant pointer to a constant string. You cannot modify the pointer or the data it points to. Use this declaration when you have a fixed string value that should not be changed throughout the program. š
š§ Easy Solutions
š If you want to declare a pointer that cannot be reassigned but can modify the data it points to, use char * const
. This is useful when you want to ensure the pointer doesn't accidentally point to something else. šÆ
š If you want to declare a pointer that can be reassigned but cannot modify the data it points to, use const char *
. This is helpful when passing string literals to functions, ensuring they won't be modified within the function. š”ļø
š” Takeaways
ā
char * const
means a constant pointer to a modifiable data.
ā
const char *
means a modifiable pointer to a constant data.
š¬ Engage With Us
Now that you understand the difference between char * const
and const char *
, try using them in your own code and see how it affects your program logic. Share your experiences and any additional tips you discover with us in the comments below. Let's learn together! š¤
If you found this blog post helpful, give it a š and don't forget to share it with your fellow programmers who might be struggling with the same question. Sharing is caring, after all! š
Thanks for reading, and until next time, happy coding! š»āØ