This C function should always return false, but it doesnβt
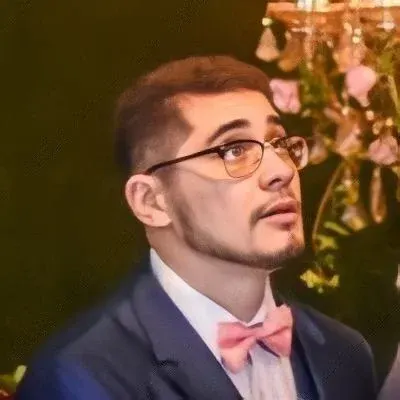

π Blog Post: Why does this C function return true when it should be false? π€
Hey there tech enthusiasts! π Are you ready for another mind-boggling coding mystery? π΅οΈββοΈ Today, we're going to dig into a puzzling question that seems to defy logic. Strap on your thinking caps, because we're about to unravel the mystery behind a C function that stubbornly returns true when it should be false. Let's dive in! π»π
The Function and Its Unexpected Behavior
Our story begins with a curious C function named f1()
. π§ The function appears to be simple enough: it calculates the sum of two variables, var1
and var2
, and assigns the result to var3
. The function then checks if var3
is equal to zero, and if so, returns true; otherwise, it returns false.
Here's the code for your reference:
#include <stdbool.h>
bool f1()
{
int var1 = 1000;
int var2 = 2000;
int var3 = var1 + var2;
return (var3 == 0) ? true : false;
}
Now, let's move on to the main()
function, where the plot thickens. π΅οΈββοΈ In the main()
function, we call f1()
twice: once to print the result directly using printf()
, and another time inside an if
statement. We expect to see only one "false" printed to the screen, but to our surprise, "executed" also appears!
Here's the main.c
code for reference:
#include <stdio.h>
#include <stdbool.h>
int main()
{
printf(f1() == true ? "true\n" : "false\n");
if (f1())
{
printf("executed\n");
}
return 0;
}
Running the program using gcc
and executing it yields the following output:
$ gcc main.c f1.c -o test
$ ./test
false
executed
Unveiling the Mystery: Undefined Behavior or Something Else?
Now, let's get to the heart of the mystery: why does the f1()
function return true when it should be false? π€·ββοΈ Does this code hide some undefined behavior, which can make our brains ache with confusion?
The answer is simpler than expected: there's no undefined behavior here! π Phew!
The key lies in the way the program calls f1()
multiple times. Each time f1()
is called, the variables var1
, var2
, and var3
are re-initialized. This means that the second call to f1()
inside the if
statement has different variable values compared to the first call, resulting in a different outcome. π
Easy Solutions to Prevent Confusion
To avoid such confusion and ensure consistent results, follow these easy solutions:
Store the result of
f1()
in a variable: Instead of callingf1()
multiple times, store its result in a variable, and use that variable in your program logic. This ensures that the function is only called once, preventing any unexpected behavior.Use a conditional block: If multiple calls to the function are necessary, use a conditional block to encapsulate the logic that should only execute when the function returns true. This way, you have more control over the flow of your program and can prevent unexpected behavior.
π‘ Pro Tip: Avoid relying on the return value of a function that mutates internal state or relies on external factors, as it may lead to unexpected outcomes. Always consider the context and purpose of the function before relying on its return value.
Join the Discussion and Share Your Insights! π£οΈ
So there you have it, fellow tech detectives! We've cracked the case of the misbehaving C function and uncovered a solution to ensure consistent behavior. But what are your thoughts and insights? Share your experiences with similar coding mysteries or any additional tips in the comments below. Let's keep the conversation going! π¬π‘
Happy coding! ππ©βπ»π¨βπ»
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
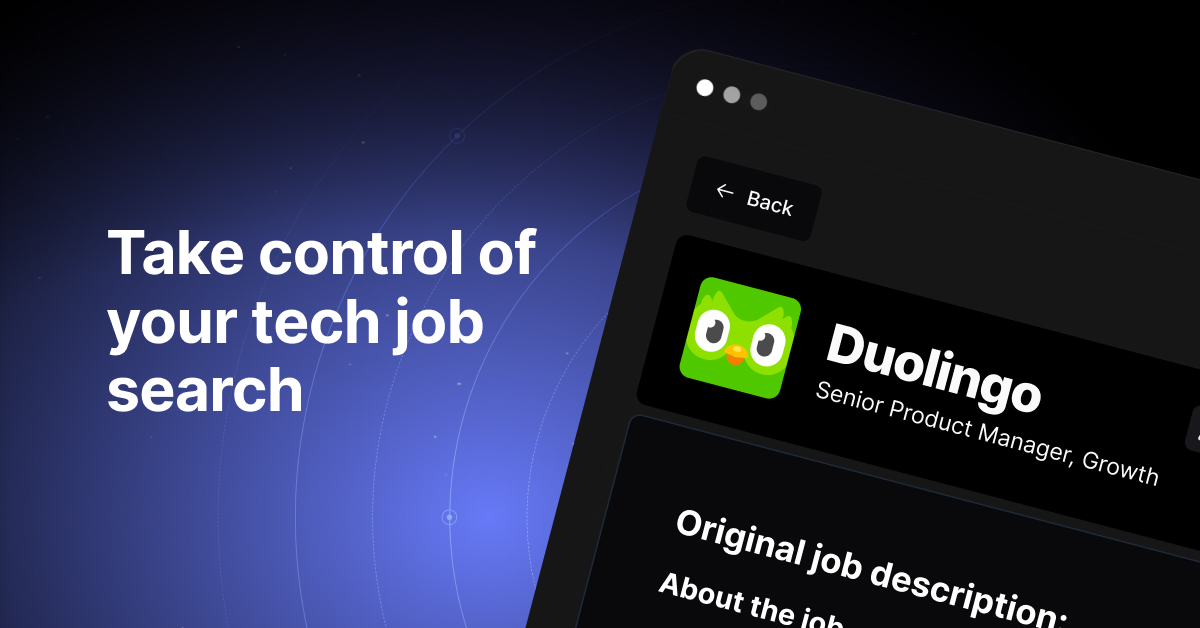