The most efficient way to implement an integer based power function pow(int, int)
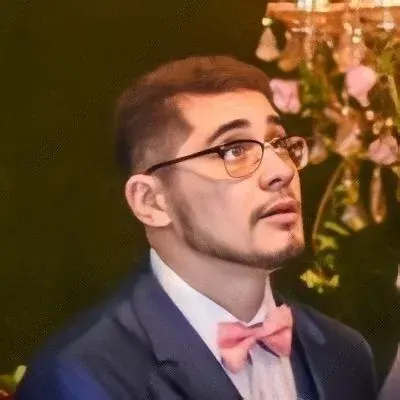
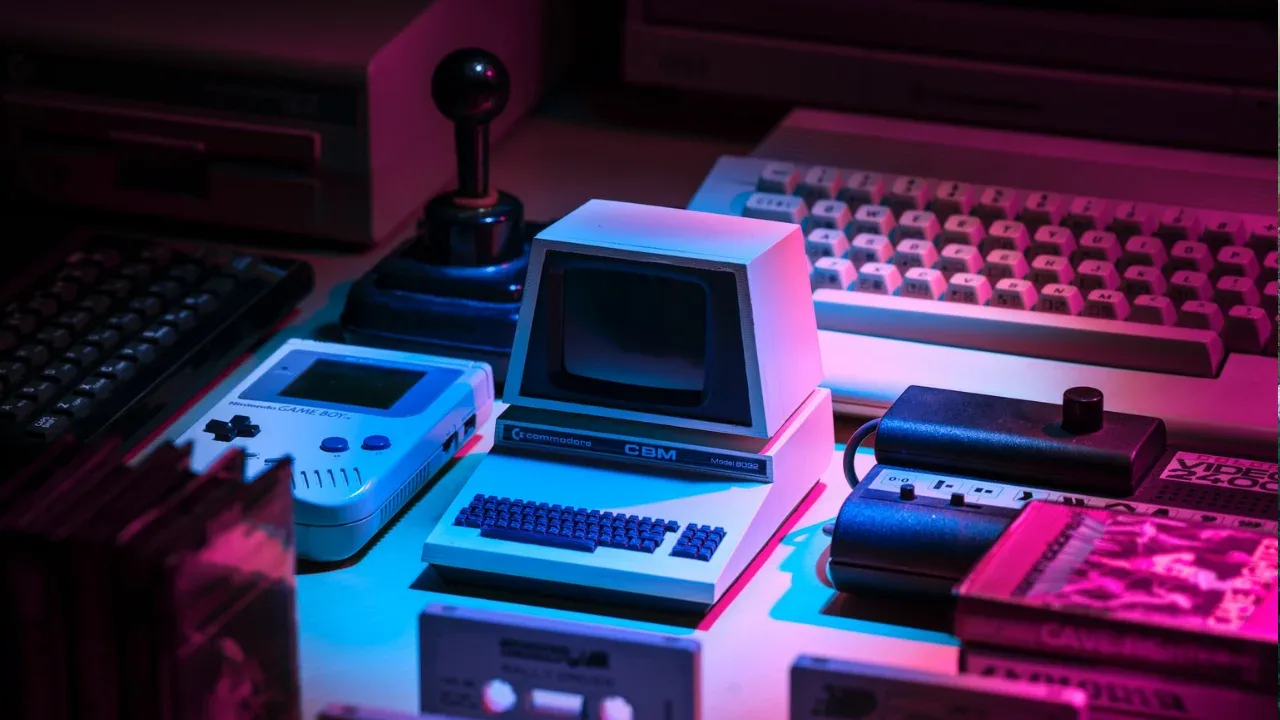
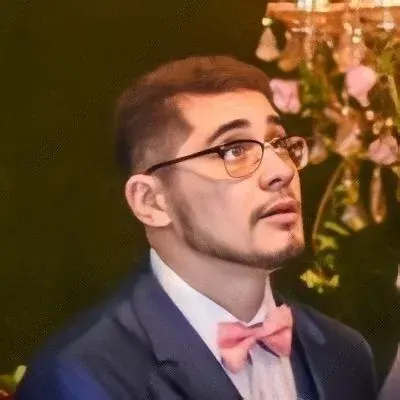
š„šŖ Power Up Your Code: Efficient Integer Power Function in C šŖš„
Are you ready to take your coding skills to the next level? Today, we're diving deep into the world of implementing an efficient integer-based power function in C. šāØ
š” The Challenge: Imagine you need to calculate the result of raising an integer to the power of another integer, such as 2 raised to the power of 3. We're looking for an optimized solution that will handle these calculations swiftly and effectively. Let's explore some common issues and their easy yet powerful solutions! šŖ
š„ Brute Force Approach - The Slow Lane š„ One tempting solution is to use a loop and multiply the base number by itself the required number of times. Let's see this in action:
int pow(int base, int exponent) {
int result = 1;
for (int i = 0; i < exponent; i++) {
result *= base;
}
return result;
}
While this method works, it isn't the most efficient approach, especially for large exponents. It requires exponential time to execute, as it performs repetitive multiplications.
š” The Optimal Solution: We're here to save you from that sluggish brute force approach! The optimal solution, known as the exponentiation by squaring algorithm, utilizes recursive calls and clever mathematical properties. Let's take a look:
int pow(int base, int exponent) {
if (exponent == 0) {
return 1;
} else if (exponent % 2 == 0) {
int result = pow(base, exponent / 2);
return result * result;
} else {
int result = pow(base, (exponent - 1) / 2);
return result * result * base;
}
}
⨠This algorithm has a time complexity of O(log n), allowing us to perform the calculations much faster, even for larger exponents. š
š Hooray! You've optimized your code with the most efficient integer power function in C! š
š£ Let's Go the Extra Mile: Now that you're armed with this powerful piece of knowledge, why not implement this function in your code and see the magic happen? Share your results with your fellow coders, and let's celebrate the speed and elegance of your optimized solution! šš»
š share: Twitter | Facebook | LinkedIn
I hope this guide helps you power up your code and inspires you to explore more efficient solutions in your programming journey. Stay tuned for more tech tips and tricks! Happy coding! š¤š»