Structure padding and packing
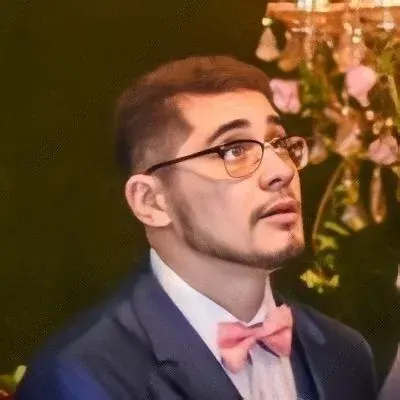
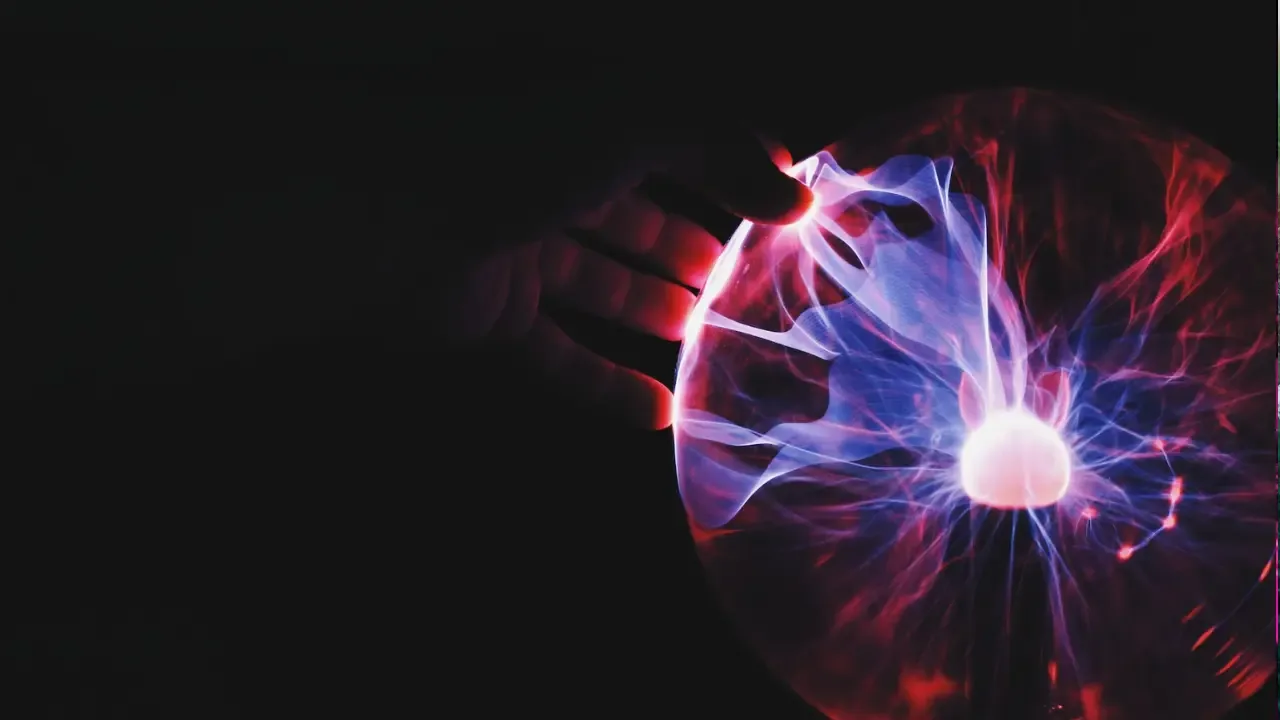
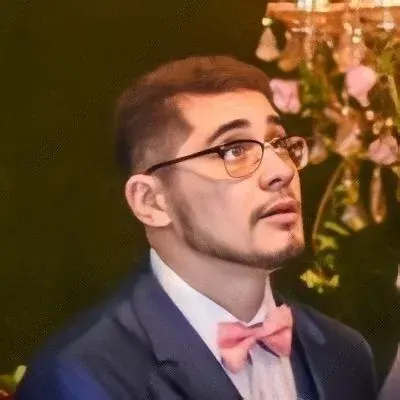
The Art of Structure Padding and Packing: Cracking the Code! 😎
Hey there tech enthusiasts! Have you ever come across those puzzling questions about structure padding and packing and wondered what they were all about? 🤔 Well, fear no more! Strap in, because we're about to dive into the fascinating world of structure alignment in programming.
Unraveling the Mystery of Padding and Packing
Let's start by unraveling the mystery of structure padding and packing using a classic example. Consider the following code snippet:
struct mystruct_A
{
char a;
int b;
char c;
} x;
struct mystruct_B
{
int b;
char a;
} y;
In this example, we have two structures: mystruct_A
and mystruct_B
. Now, let's analyze their sizes.
The size of mystruct_A
is 12 bytes, while mystruct_B
is 8 bytes. But what's the reason behind these sizes, and how does padding or packing play a role? Let's find out! 🕵️♂️
Padding or Packing: The Ultimate Question
The concept of structure padding and packing revolves around aligning the members of a structure to certain memory boundaries. This alignment is crucial for optimizing memory access and ensuring efficient performance in systems that rely heavily on memory operations.
1. Structure Padding
Structure padding occurs when the compiler introduces extra bytes within a structure to align its members according to specific memory boundaries. This alignment is often based on the size and alignment requirements of the members.
In mystruct_A
, the compiler adds 3 extra bytes after the member c
to align the subsequent int
member, b
, on a 4-byte boundary. This results in a total size of 12 bytes, as shown below:
struct mystruct_A
{
char a; // 1 byte
[padding] // 3 bytes
int b; // 4 bytes
char c; // 1 byte
} x;
2. Structure Packing
Structure packing, on the other hand, aims to minimize the memory footprint of a structure by eliminating any unnecessary padding bytes. This is achieved by reducing or disabling the automatic alignment of structure members.
In mystruct_B
, the order of members b
and a
is swapped compared to mystruct_A
. As a result, there are no additional padding bytes introduced, resulting in a size of only 8 bytes:
struct mystruct_B
{
int b; // 4 bytes
char a; // 1 byte
} y;
When Does Padding or Packing Take Place?
Now that we've demystified the concepts of padding and packing, you might be wondering when they actually take place. Let's break it down:
Padding typically occurs when a structure contains members with different sizes or alignment requirements. The aim is to ensure efficient memory access by aligning the larger members on appropriate boundaries.
Packing comes into play when you explicitly instruct the compiler to minimize or eliminate padding within a structure. This can be done using compiler-specific directives or pragmas.
Ultimately, the decision to pad or pack a structure depends on the specific requirements of your application, memory usage considerations, and the targeted platform.
Crunching the Numbers: Summing It All Up
To recap, structure padding and packing are techniques used to optimize memory access and minimize memory consumption in programming.
Padding adds extra bytes within a structure to align members according to memory boundaries, enhancing performance at the expense of larger memory usage.
Packing reduces or removes padding to minimize memory consumption but may result in slower memory access due to inefficient alignment.
Understanding when and how to use padding or packing can significantly impact the performance and memory utilization of your applications. So, don't be afraid to experiment and find the optimal balance based on your specific use case! 🚀
And that's a wrap, folks! We hope this guide has shed some light on the confusing world of structure padding and packing. Embrace the knowledge, apply it wisely, and optimize your code like a true tech warrior!
Are you facing any other mind-boggling programming problems? Share them with us in the comments below, and let's conquer them together! 💪🔥