strdup() - what does it do in C?
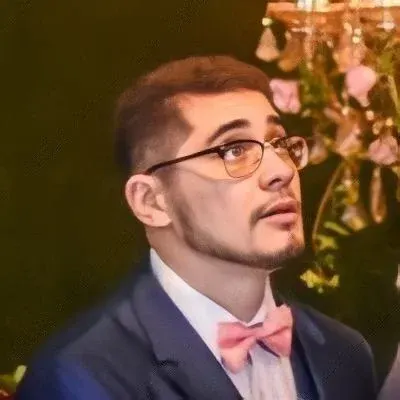
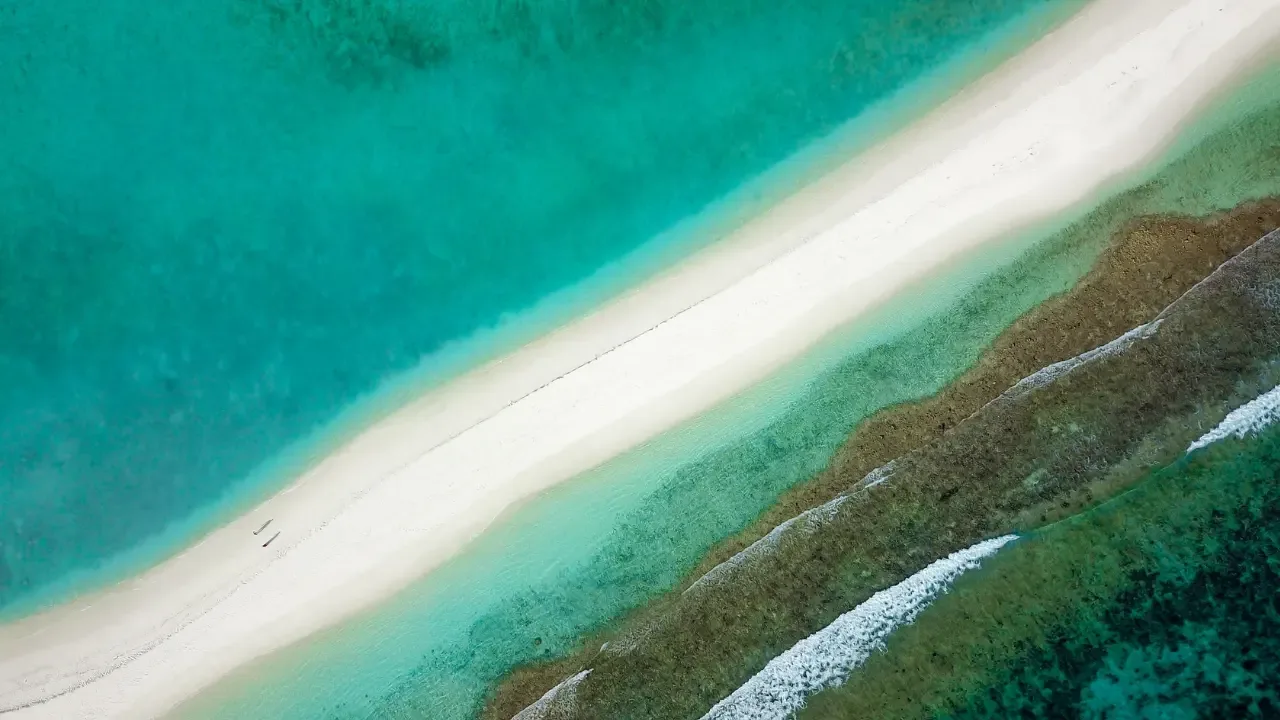
πTitle: Decoding the Magic of strdup() in C - Unraveling its Purpose and Potential Pitfalls! π§ββοΈβ¨
π Hey there, tech enthusiasts! Today, we'll dive into the fascinating world of the strdup() function in C. Ever wondered what this mysterious function does? We got you covered! πͺπ
π€ What is the purpose of the strdup() function?
In C, the strdup() function is used to duplicate a given string. It takes a string as input and creates a new copy of that string in memory, allowing you to safely modify or manipulate it without affecting the original. ππ¨οΈ
This handy function saves you the trouble of manually allocating memory, initializing it, and copying the string character by character. It does all that heavy lifting for you, making your code cleaner and more concise. π»π₯
π‘Pro Tip: strdup() stands for "string duplicate," so think of it as a copy machine for strings! π¨οΈποΈ
π₯ Potential Pitfalls you need to be aware of
While strdup() seems like a magical solution, it's crucial to be aware of potential pitfalls to avoid unexpected errors or memory leaks. Here are some important points to keep in mind:
Null Check: strdup() uses dynamic memory allocation, so you should always validate if the input string is not null before calling strdup(). Otherwise, it may lead to undefined behavior. π¨π§
Memory Leaks: Remember to free the memory allocated by strdup() once you are done using the duplicated string. Failing to do so can result in memory leaks, which might hamper your code's efficiency and performance. π‘π
π§ Easy Solutions to common strdup() issues
To help you navigate through common issues that might arise while using strdup(), here are a few easy solutions:
1. Handling null input strings
char* duplicateString(const char* originalString) {
if (originalString == NULL) {
// Handle error or return appropriate value
}
char* duplicate = strdup(originalString);
// Perform operations on duplicate
return duplicate;
}
2. Freeing the memory allocated by strdup()
char* duplicateString(const char* originalString) {
// Check for null inputs as mentioned earlier
char* duplicate = strdup(originalString);
// Perform operations on duplicate
free(duplicate); // Don't forget to free the memory!
return duplicate;
}
π’ Let's Engage!
Now that you've learned about strdup(), we want to hear from you! Have you ever used this function in your C projects? Share your experiences and thoughts in the comments below! π¬π€©
Remember, strdup() can be your superhero when it comes to duplicating strings in C, but be cautious about potential pitfalls and always appreciate the power of freeing allocated memory. Stay safe, code responsibly! π¦ΈββοΈπ»π
Happy coding! ππ‘
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
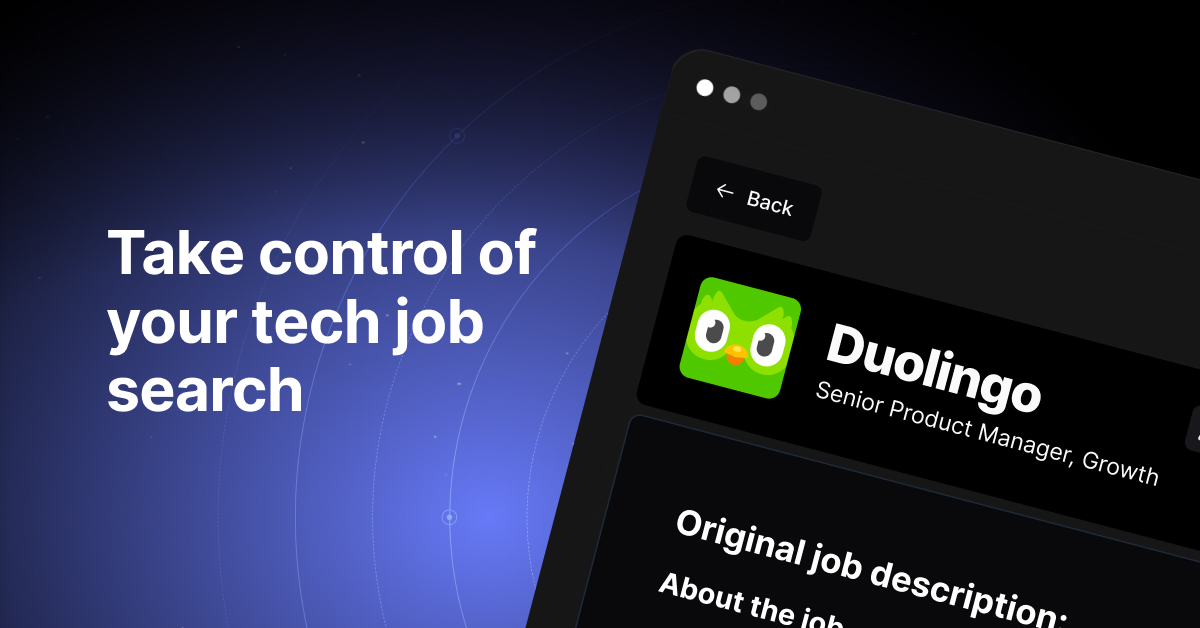