Removing trailing newline character from fgets() input
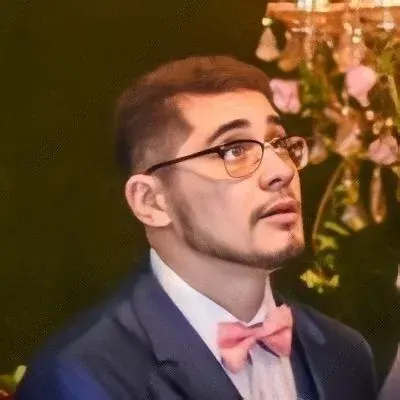
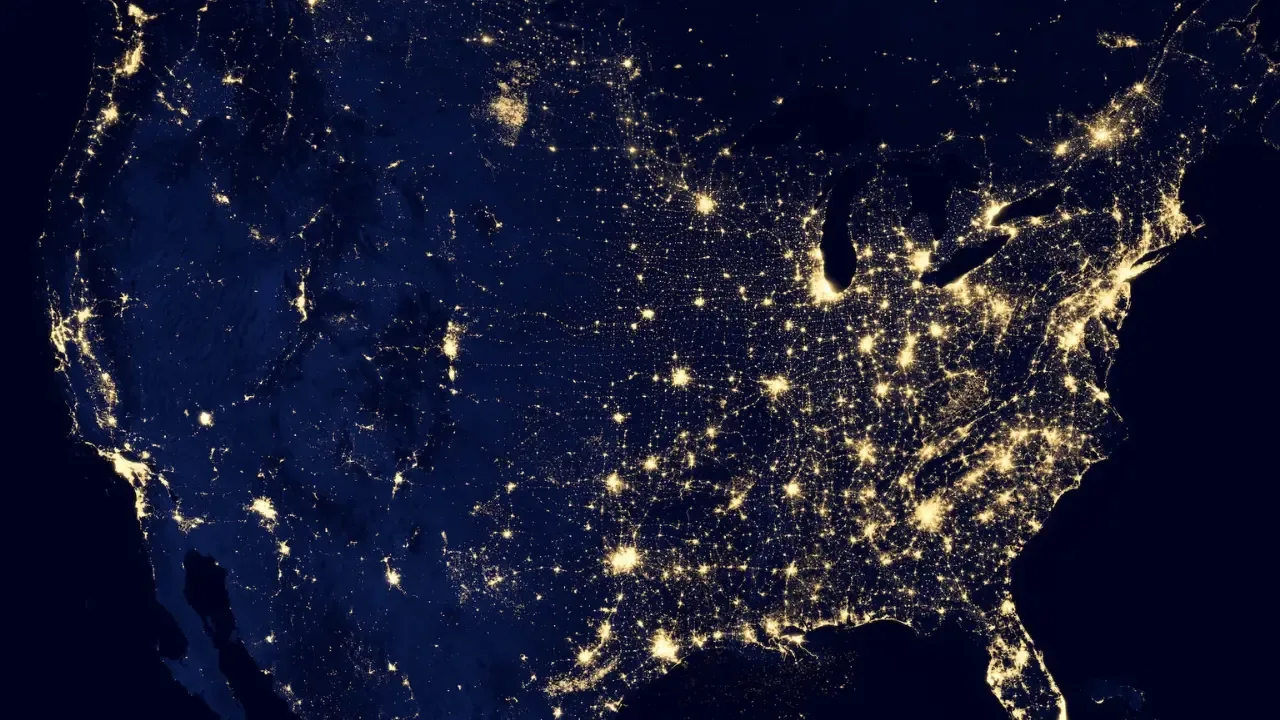
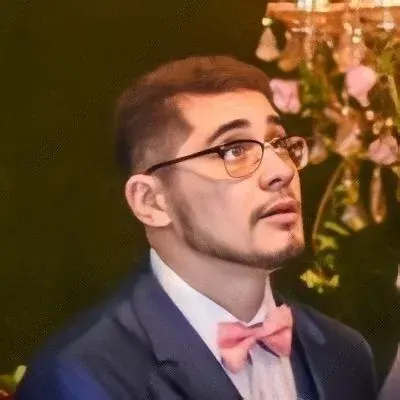
Removing trailing newline character from fgets() input
š Hey there! Are you struggling with removing that pesky trailing newline character from fgets() input? Don't worry, you're not alone. Many programmers face this issue while getting user input using fgets().
š Let's dive into the problem and explore some easy solutions to remove that unwanted newline character and send a proper string to your function.
The Problem
š¤ Here's a scenario to provide some context. You're using fgets() to get user input, like a name, and pass it to another function. However, when you print or use the input, you notice that it includes the newline character '\n' at the end.
<pre><code>printf("Enter your Name: "); if (!(fgets(Name, sizeof Name, stdin) != NULL)) { fprintf(stderr, "Error reading Name.\n"); exit(1); } </code></pre>
š In the given example, if you enter "John", it ends up sending "John\n". š¤·āāļø
The Solution
š” To remove the trailing newline character from the fgets() input, we can make use of the strcspn()
function. The strcspn()
function returns the index of the first occurrence of any character from a given set in a string.
šÆ Here's how you can use strcspn()
to remove the trailing newline character:
Name[strcspn(Name, "\n")] = '\0';
š In the above code snippet, strcspn(Name, "\n")
gives you the index of the first '\n' character. š By assigning '\0' (null character) to that index, we effectively remove the trailing newline character from the string.
š Now your string will be "John" instead of "John\n"! You can then pass this cleaned-up string to your desired function.
Example Implementation
š Let's update our original code snippet with the solution. This way, you can see how it works in action.
printf("Enter your Name: ");
if (!(fgets(Name, sizeof Name, stdin) != NULL)) {
fprintf(stderr, "Error reading Name.\n");
exit(1);
}
Name[strcspn(Name, "\n")] = '\0'; // Removing the trailing newline character
someFunction(Name); // Pass the cleaned-up string to your desired function
š By adding the line Name[strcspn(Name, "\n")] = '\0';
, you ensure that the trailing newline character is removed before passing the string to someFunction()
.
Call-to-Action
š Congratulations! You now know how to remove the trailing newline character from fgets() input. Go ahead and give it a try in your own code. You'll find that your strings are cleaner and more useful without that extra newline.
š¬ If you have any questions or other programming-related topics you'd like me to cover in future blog posts, leave a comment below. I'd love to hear your thoughts and help you out.
šÆ Keep coding and keep exploring those awesome tech solutions! š