Printing leading 0"s in C
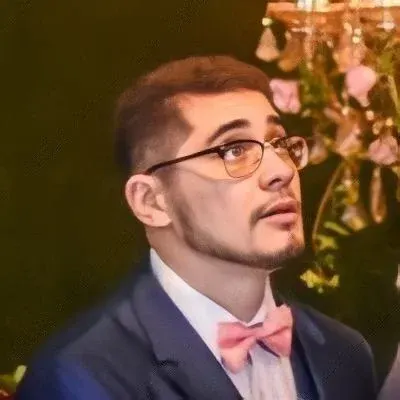

Printing Leading 0's in C: A Handy Guide 👨💻🔢
Do you need to print leading 0's in C, such as "01001" for a ZIP Code? 📬 While the number is stored as "1001", finding a good way to format it with leading 0's can prove to be a bit tricky. But fret not! In this blog post, we'll explore common issues and provide you with easy solutions using the printf format syntax. Let's dive right in! 🏊♂️
The Struggle is Real 😩
You might be wondering, "Should I use case statements or if statements to handle this?" While those can be viable options, let us introduce you to a more elegant solution using the power of printf format specifiers. 💪🔥
Solution 1: Using Precision Width ⚙️
By specifying the precision width in the format specifier, we can achieve the desired result. Let's take a look at an example:
#include <stdio.h>
int main() {
int zipCode = 1001;
printf("%05d\n", zipCode);
return 0;
}
In this example, we have used the format specifier "%05d". Here's how it works:
The "0" indicates that we want to pad the number with leading 0's.
The "5" specifies the total width of the number, including leading 0's.
The "d" signifies that we are dealing with a decimal integer.
As a result, the output will be "01001". Easy, right? 🎉
Solution 2: Using Format String Calculations 🧮
Sometimes, you might need a more dynamic solution that adapts to varying lengths of numbers. In such cases, format string calculations can come to the rescue. Take a look at this example:
#include <stdio.h>
int main() {
int zipCode = 1001;
int numDigits = snprintf(NULL, 0, "%d", zipCode);
printf("%0*d\n", numDigits + 3, zipCode);
return 0;
}
Here's how it works:
We calculate the number of digits in the zipCode using the snprintf function with a NULL destination.
The result is stored in numDigits.
We then use the format specifier "%0*d" to specify a dynamic precision width using the numDigits variable.
Adding 3 to numDigits accounts for the extra leading 0's we desire.
Voila! The output will be "01001" once again. 🎊
Spread the Word 📣
Now that you've learned two easy ways to print leading 0's in C, why not share your newfound knowledge with others? 🌟 Help your fellow developers facing the same struggle by sharing this blog post with them. Let's empower the coding community together! 💪💙
If you have any further questions or alternative approaches to this problem, feel free to leave a comment below. Happy coding! 🚀💻
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
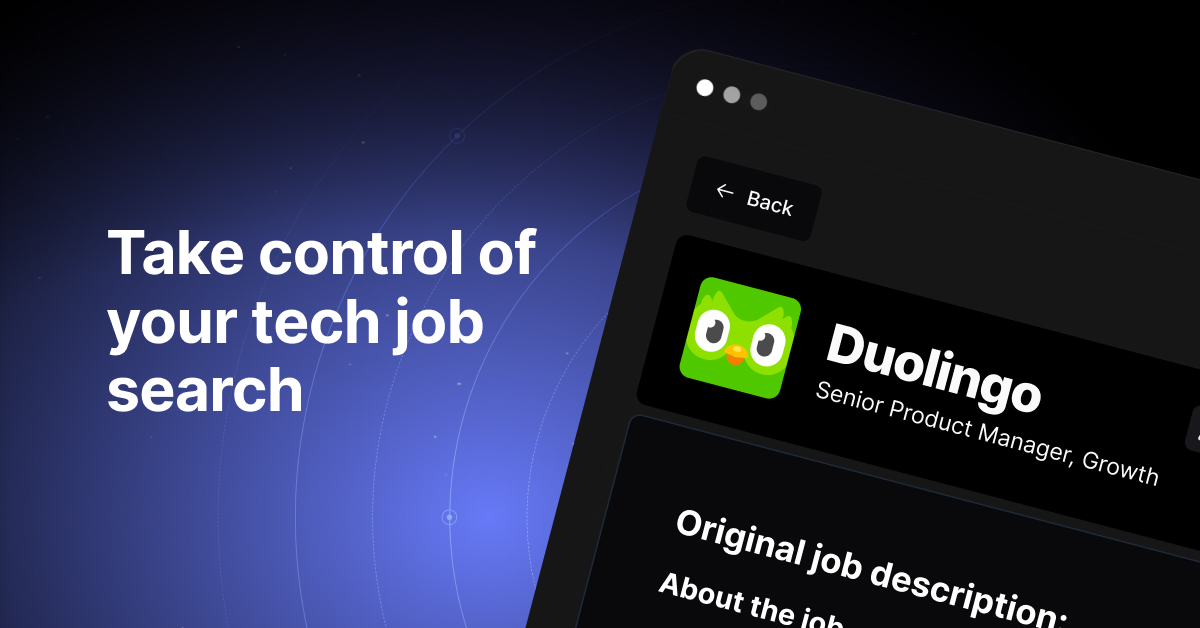