Preventing console window from closing on Visual Studio C/C++ Console application
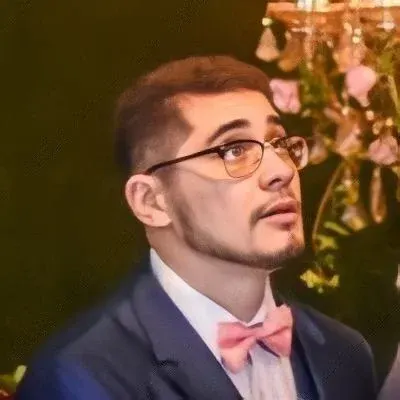
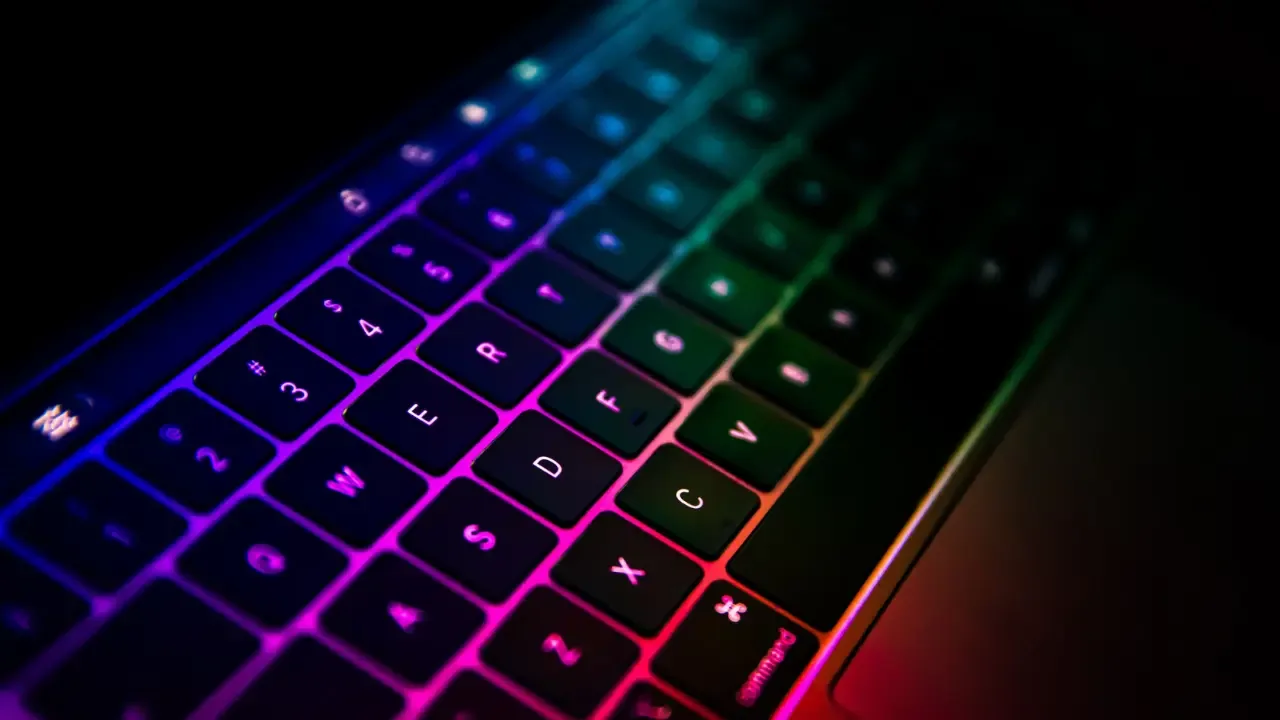
🚀 Keeping the Console Window Open in Visual Studio C/C++ Console Application
Hey there, console application developers! 👋
If you're using Visual Studio for the first time to develop a console application and the console window keeps closing as soon as your program exits, don't worry! You're not alone in asking this question. Many beginners face this same challenge, and in this blog post, we'll tackle this annoying issue head-on and provide you with easy solutions. 🛠️
Problem: The Console Window Closes Too Quickly 😫
So, you've written your code, hit the run button, and voila! The console window appears, you see your program's output, and just as you're about to examine the results, poof, the window disappears. Sound familiar? We've got you covered!
Solution 1: The Trusty getchar()
Function 🙌
One simple and effective way to keep the console window open is by using the getchar()
function from the stdio.h
or cstdio
library. This function prompts the user to enter a character and waits until one is pressed. By adding getchar()
at the end of your code, the program will halt its execution and wait for your input, keeping the window open until you're ready to close it manually.
#include <stdio.h>
int main() {
// Your code goes here
getchar(); // Keeps the console window open until a character is entered
return 0;
}
Now you can leisurely examine your program's output before closing the console window. 🕵️♂️
Solution 2: Debugging Techniques 🐞
Another quick way to prevent the console window from closing is to run your console application in debug mode. Here's how:
Add a breakpoint at the end of your code by clicking on the left margin of the line or pressing
F9
.Hit the "Start Debugging" button in the toolbar or press
F5
to run your program in debug mode.The console window will now stay open until you manually stop debugging by pressing
Shift + F5
or the stop button.
Debug mode allows you to step through your code, set additional breakpoints, and closely examine the behavior of your program. 🕵️♀️
Solution 3: Lasting Impressions with system("pause")
🌟
If your code doesn't need to abide by strict cross-platform conventions, you can use the system("pause")
command. This approach forces the console window to wait for your action by executing the "pause" command in the operating system shell. Just add it at the end of your code, and the console window will remain open until you hit any key to continue.
#include <stdio.h>
#include <stdlib.h>
int main() {
// Your code goes here
system("pause"); // Keeps the console window open until any key is pressed
return 0;
}
Please note that this solution may not work in some non-Windows systems or on systems without a command line shell.
Call-to-Action: Share Your Experience! 📢
Congratulations! You can now prevent the console window from closing on your Visual Studio C/C++ Console Application. We hope these solutions made your development journey smoother. But we want to hear from you!
Have you ever faced this issue before? Which solution worked best for you? We'd love to know! Share your experience in the comments section below and help others facing the same challenge. Let's learn from each other and build a strong developer community! 🌟
Happy coding! 💻
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
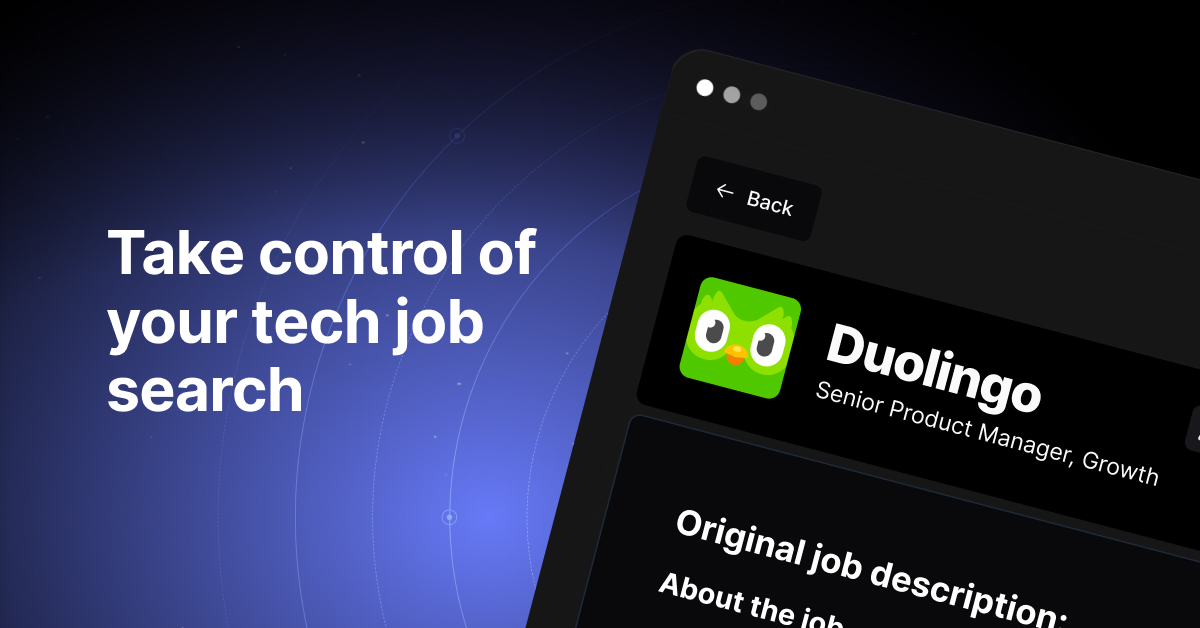