Pointers in C: when to use the ampersand and the asterisk?
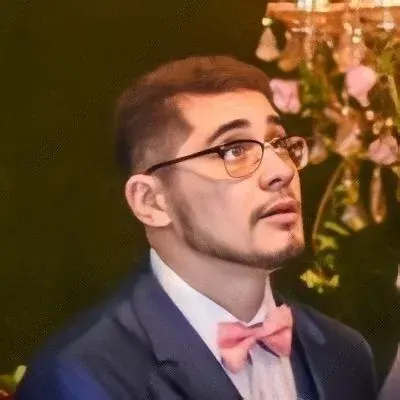
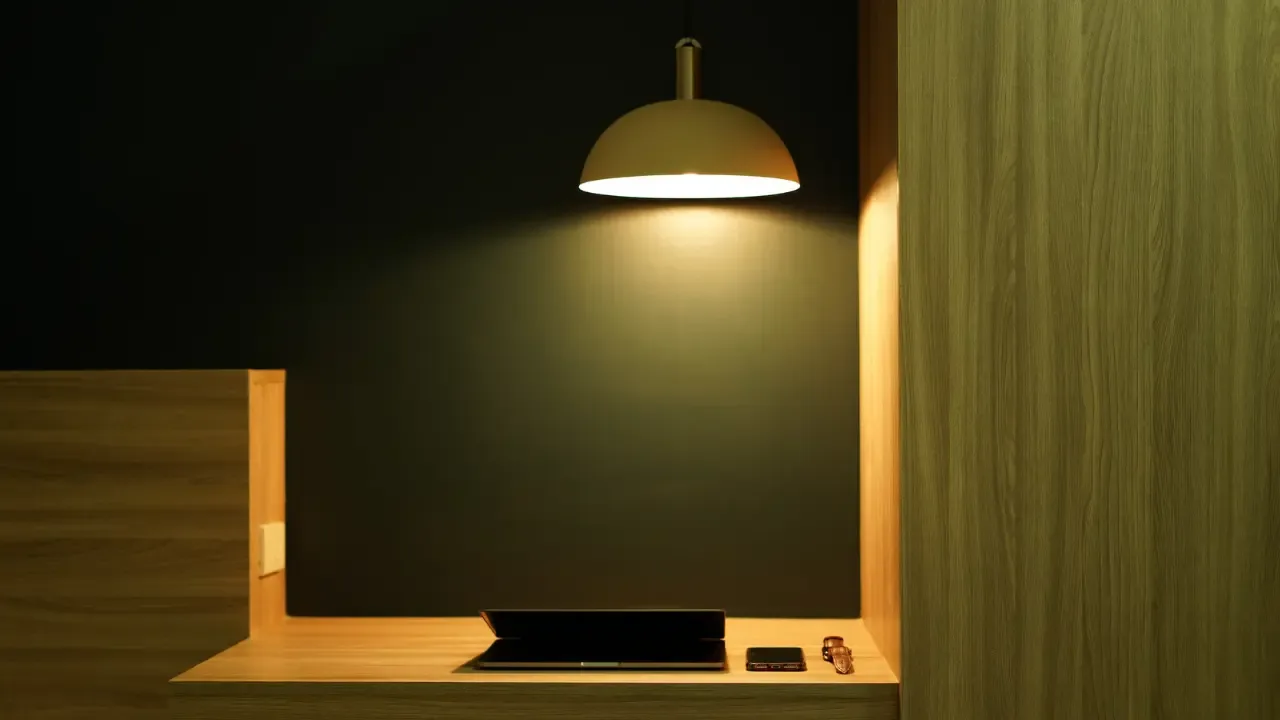
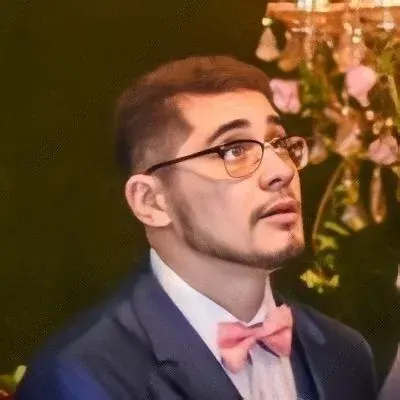
๐๐๐คDiscovering the Logic Behind Pointers in C: Breaking Down the Mystery of (&) and (*)๐๐๐ก
Are you new to the world of pointers in C and finding yourself slightly perplexed? Don't worry! We're here to help you make sense of those cryptic symbolsโthe ampersand (&) and the asterisk (*)โthat often seem to complicate things. ๐งฉ๐
Let's clear up the confusion and uncover the logic behind these pointer operators, highlighting when to use them in various scenarios. We'll dive into the common issues you may encounter while working with arrays, strings, and passing pointers to functions, providing easy-to-apply solutions along the way. ๐๐ซ๐งฉโก๏ธ๐๏ธโ
Understanding the Basics
First, let's establish the fundamentals. The ampersand (&) symbol is used to find the memory address of a variable. On the other hand, the asterisk (*) symbol can be used in front of a pointer variable to retrieve the value stored at the memory location pointed to by that pointer. ๐
Scenario 1: Pointers and Variables
Suppose we have a variable myVariable
with the value 42
, and we want to store its memory address in a pointer variable myPointer
. Here's how we can do it:
int myVariable = 42; // Our variable
int *myPointer = &myVariable; // Our pointer, stores the address of myVariable
Notice how the ampersand (&) operator helps us retrieve the address of myVariable
and store it in myPointer
. Cool, right? ๐ค
Scenario 2: Retrieving Values from Pointers
But what if we want to get the value stored at the memory location pointed to by myPointer
? Do we need yet another magic trick? Not at all! By using the asterisk (*) operator, we can obtain the desired value. Let's take a look:
int myValue = *myPointer; // Retrieves the value stored at the memory location pointed to by myPointer
As you can see, the asterisk (*) operator grants us access to the value held by the pointer. It's like having the key to unlock the treasure! ๐ฐ๐๐ก
Arrays, Strings, and Function Pointers? Oh My!
Things tend to become a bit trickier when we deal with arrays, strings, and passing pointers to functions. But persevere, young Padawan, we'll guide you to enlightenment!
Arrays and Pointers
In C, an array is essentially a pointer to the first element of the array. Therefore, we don't need to use the ampersand (&) operator to obtain the address of the array. For instance:
char myArray[] = "Hello, World!"; // An array of characters
// No need for & operator, as myArray already represents the address of the first element
char *myPointer = myArray; // Storing the address of the first element in myPointer
By letting myArray
represent the memory address of the first element, we can assign it directly to our pointer variable. ๐ช๐
Strings and Pointers
In C, strings are arrays of characters, and we can leverage pointers to access or manipulate them. Let's see how that works:
char *myString = "Hello, World!"; // A string represented by a char pointer
Here, myString
directly points to the memory location where the first character of the string is stored. No need for any additional operatorsโjust the pointer and the string! ๐ฌ๐โ๏ธ๐
Passing Pointers to Functions
Now, let's talk about passing pointers to functions. When we pass a pointer to a function, it allows us not only to share data but also to modify it without returning a value. Observe this example:
void modifyValue(int *myPointer) {
*myPointer = 100; // Modifies the value at the memory location pointed to by myPointer
}
int main() {
int myVariable = 42;
// Passes the address of myVariable to modifyValue function
modifyValue(&myVariable);
// myVariable has been modified
// Now it stores the value 100
}
By utilizing the reference to the memory address of myVariable
(denoted by &myVariable
), we are not just passing the value but also the ability to modify it directly within the function. Magic happens when pointers come into play! ๐ช๐ฅ๐ง
Your Pointer Journey Continues!
Congratulations! You've journeyed through the realm of (&) and (*) operators in C and gained valuable insights about pointers. But your adventure doesn't end here. Keep exploring, experimenting, and becoming more comfortable with pointers. ๐๐
If you have any additional questions or seek further guidance on pointers or any other coding topics, leave a comment below. We'd love to hear about your experiences and help you level up your programming expertise! ๐ค๐ฌโ
So, gear up, fellow coders, and let's continue unraveling the mysteries of the tech universe, one concept at a time! Share this post with your friends and colleagues to spread the pointer wisdom far and wide. Until next time, happy coding! ๐งโ๐ปโจ๐ข
โจ๏ธ๐ก๐ #coding #pointers #Cprogramming