Passing variable number of arguments around
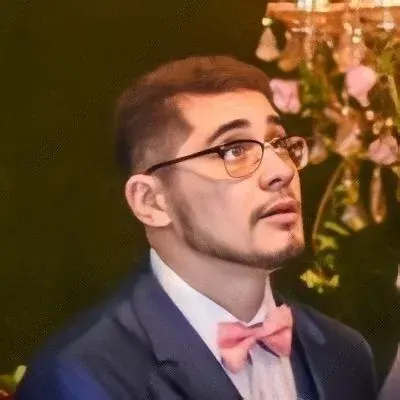
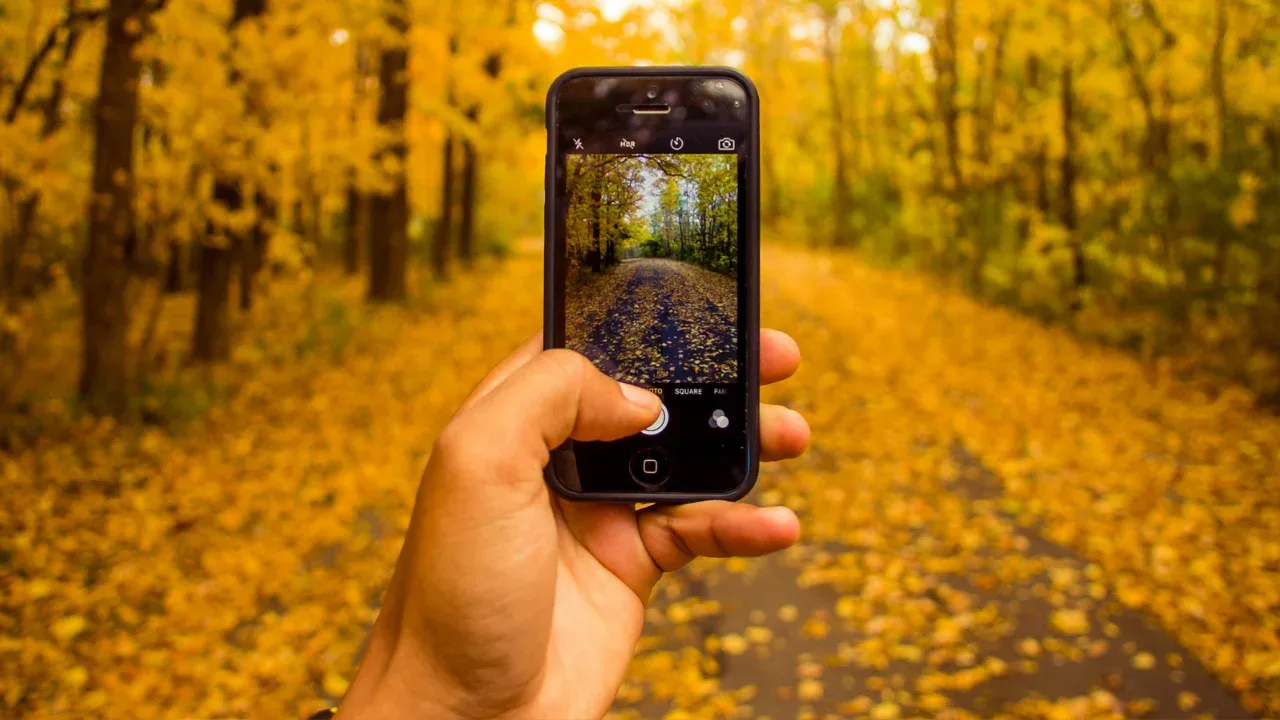
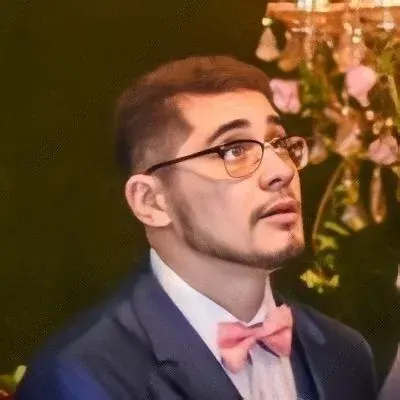
Passing Variable Number of Arguments: A Guide to Solving Common Issues
Have you ever encountered a situation where you need to pass a variable number of arguments around in your code? 🤔 It can be a bit tricky, especially when it comes to calling another function that expects this kind of flexibility. But fear not! In this blog post, we'll dive deep into this topic, provide you with easy solutions, and empower you to conquer this coding challenge! 💪
The Scenario: Passing Variable Number of Arguments
Let's consider a practical scenario to better understand the problem at hand. Imagine you have a C function called format_string
that takes a variable number of arguments. Now, you need to call another function, debug_print
, from within this first function, and pass all the arguments that have been received by debug_print
to format_string
. 🔄
void format_string(char *fmt, ...);
void debug_print(int dbg_lvl, char *fmt, ...) {
format_string(fmt, /* how do I pass all the arguments from '...' ? */);
fprintf(stdout, fmt);
}
As you can see in the example above, the debug_print
function has its own set of arguments, including dbg_lvl
and fmt
. The challenge lies in passing the remaining arguments, received through the ...
notation, to the format_string
function. But don't worry, we've got you covered! 😉
Solution 1: Using a Variadic Macro
One straightforward solution to this problem involves using a variadic macro. A macro is a piece of code that gets expanded by the preprocessor before the actual compilation takes place. In this case, we can define a macro that takes in all the arguments and expands them as needed. Here's how you can implement it: 🛠️
#define DEBUG_PRINT(fmt, ...) do { \
format_string(fmt, ##__VA_ARGS__); \
fprintf(stdout, fmt, ##__VA_ARGS__); \
} while (0)
With this macro, you can now replace the debug_print
function with a simple macro invocation:
DEBUG_PRINT("%d %s", 42, "Hello World");
Awesome, right? This solution allows you to pass any number of arguments to the format_string
function without worrying about explicit argument handling.
Solution 2: Using stdarg.h
Another viable solution revolves around utilizing the stdarg.h
header file. This header provides facilities for accessing variable arguments in functions. By using the va_list
, va_start
, and va_end
macros, you can achieve the desired outcome. Let's see how it works in practice: ⌨️
#include <stdarg.h>
void debug_print(int dbg_lvl, char *fmt, ...) {
va_list args;
va_start(args, fmt);
format_string(fmt, args);
va_end(args);
fprintf(stdout, fmt);
}
In this approach, we declare a va_list
variable, args
, which will store the variable arguments received by the debug_print
function. va_start
initializes the args
list with the first argument after fmt
, and va_end
cleans up the resources allocated for the variable argument list. Using this method ensures that all the arguments are correctly passed to the format_string
function.
Conclusion
Passing a variable number of arguments within complex code scenarios can be challenging. However, armed with the knowledge provided in this guide, you now have two powerful tools at your disposal: the variadic macro solution and the stdarg.h
approach. 🎉
Remember, the key takeaway is to choose the solution that best fits your specific use case. Whether you prefer the simplicity of macros or the flexibility of the stdarg.h
library, the choice is yours!
Take a moment to reflect on your own experiences with variable arguments and share your thoughts in the comments below. We'd love to hear about your unique challenges and the creative solutions you've implemented. Let's learn and grow together! 🚀