Is there a printf converter to print in binary format?
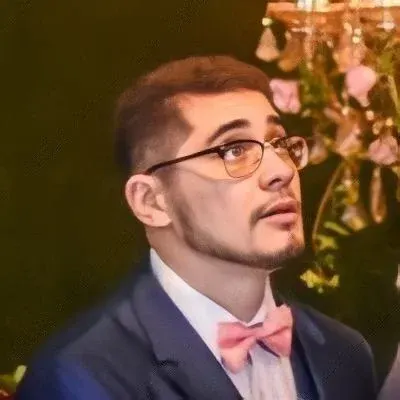
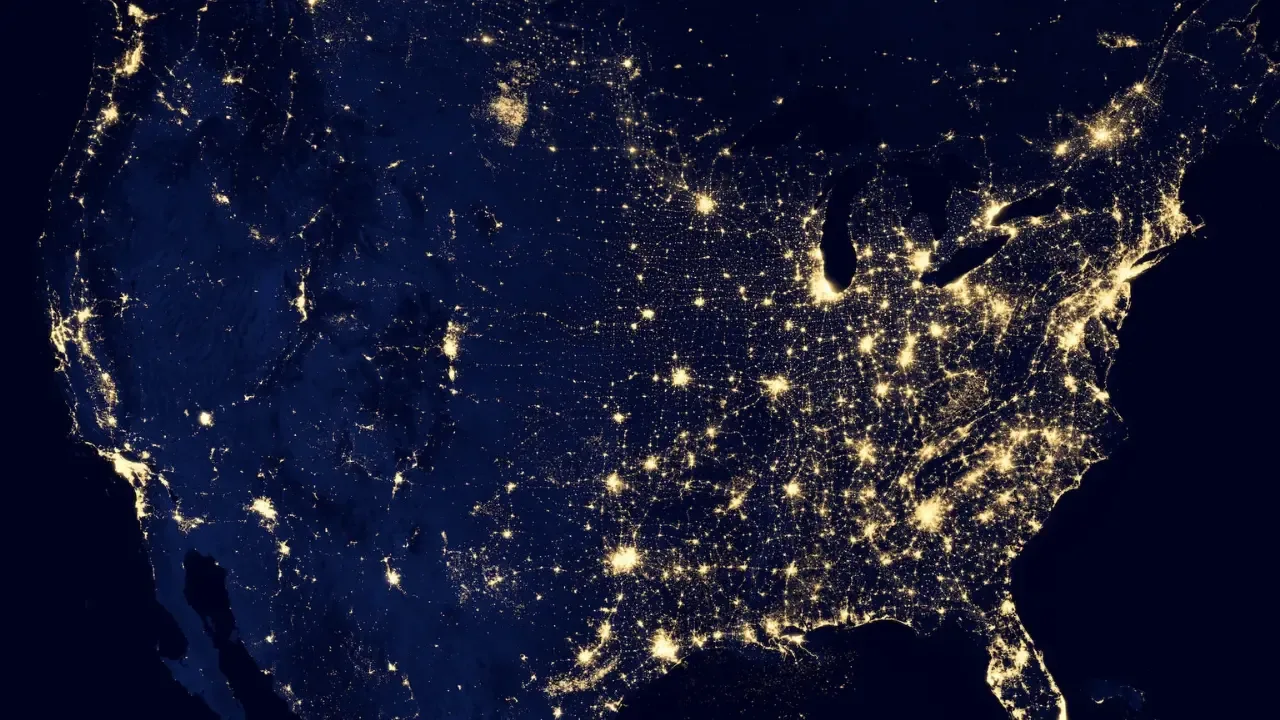
📝💥🔄Blog Post Title: "Print in Binary with printf: The Complete Guide"
Introduction:
Hey there tech enthusiasts! 👋 Have you ever wondered if there's a way to print numbers in binary format using the venerable printf
function? Well, you're in luck because we're here to demystify this coding conundrum for you. In this guide, we'll explore the common issues, provide easy solutions, and get you ready to rock and roll with binary printing. Let's dive in!
Common Issue: Printing in Binary with printf
The question that sparked this blog post was an interesting one. The developer wanted to know if there was a format tag that would allow them to print numbers in binary, or arbitrary base. By default, printf
supports printing numbers in decimal, hexadecimal, and octal formats using the %d
, %x
, and %o
format specifiers, respectively. But what about binary?
The Challenge: printf Doesn't Have a Native %b Format Specifier
Unfortunately, the printf
function doesn't have a built-in format specifier for binary numbers like it does for other bases. This poses a challenge when we want to print numbers in binary format using printf
.
Easy Solution: Writing a Binary Conversion Function To overcome this challenge, we can write a simple binary conversion function that takes an integer input and converts it into its binary representation as a string. Here's an example of how we can achieve this in C:
void printBinary(int num) {
if (num > 1)
printBinary(num / 2);
printf("%d", num % 2);
}
Explanation: The function printBinary
recursively calculates the binary representation of the number num
by dividing it by 2 and printing the remainder until the number becomes less than or equal to 1. This process essentially reverses the bits of the binary representation and outputs it in the correct order using printf("%d", num % 2)
.
Usage Example: Printing Numbers in Binary with printf
Now that we have our handy printBinary
function ready, let's see how we can use it to print numbers in binary format with printf
. Here's an example:
int main() {
int number = 10;
printf("Binary: ");
printBinary(number);
printf("\n");
return 0;
}
Output:
Binary: 1010
Voila! 🎉 We've successfully printed the number 10 in binary format using printf
and our custom printBinary
function.
Call-To-Action: Share Your Binary Printing Adventures!
Now it's your turn to unleash your coding prowess and experiment with printing numbers in binary format using printf
. Share your experiences, code snippets, and any other interesting findings in the comments below. Let's learn together and level up our programming superpowers! 💪
Conclusion:
Printing numbers in binary format using printf
may not be as straightforward as with other bases, but with a little creativity and code magic, we can achieve our goal. By writing a simple binary conversion function, we can print numbers in binary format and expand the capabilities of printf
. So go ahead, give it a shot, and impress your fellow programmers with your binary printing skills!
Remember, the only limit is your imagination! 🚀
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
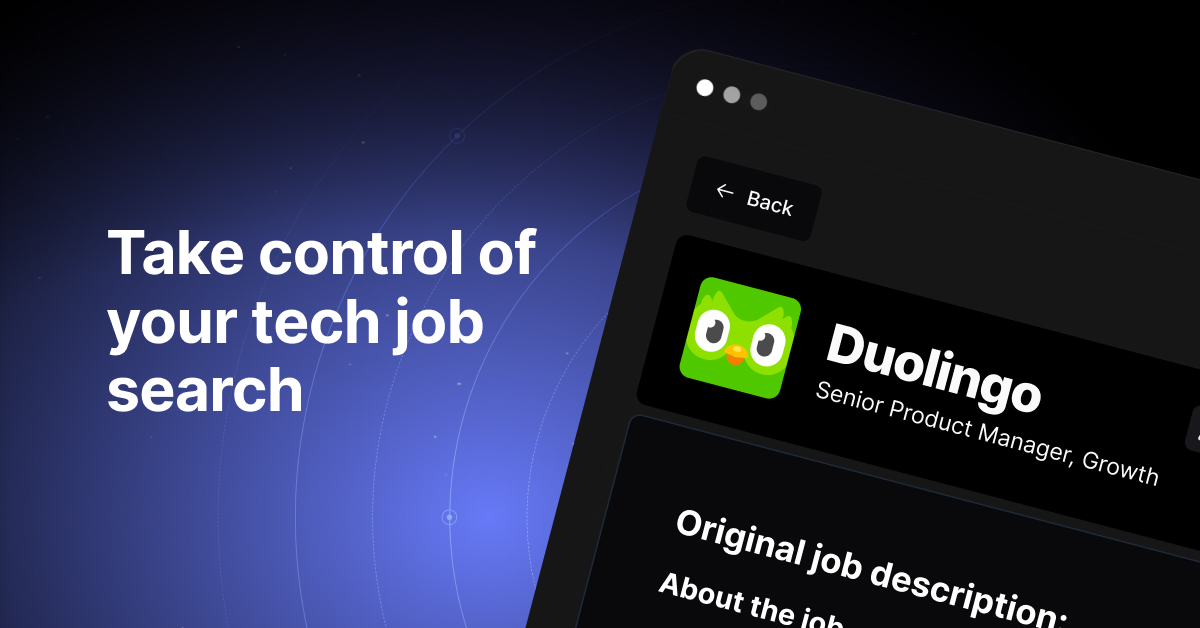