Is bool a native C type?
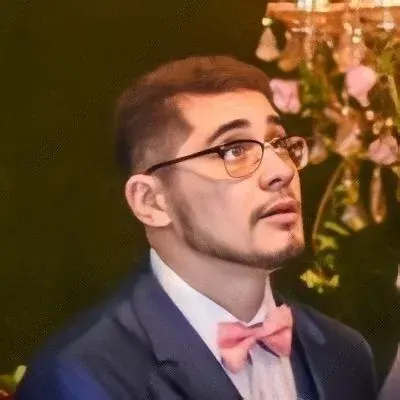
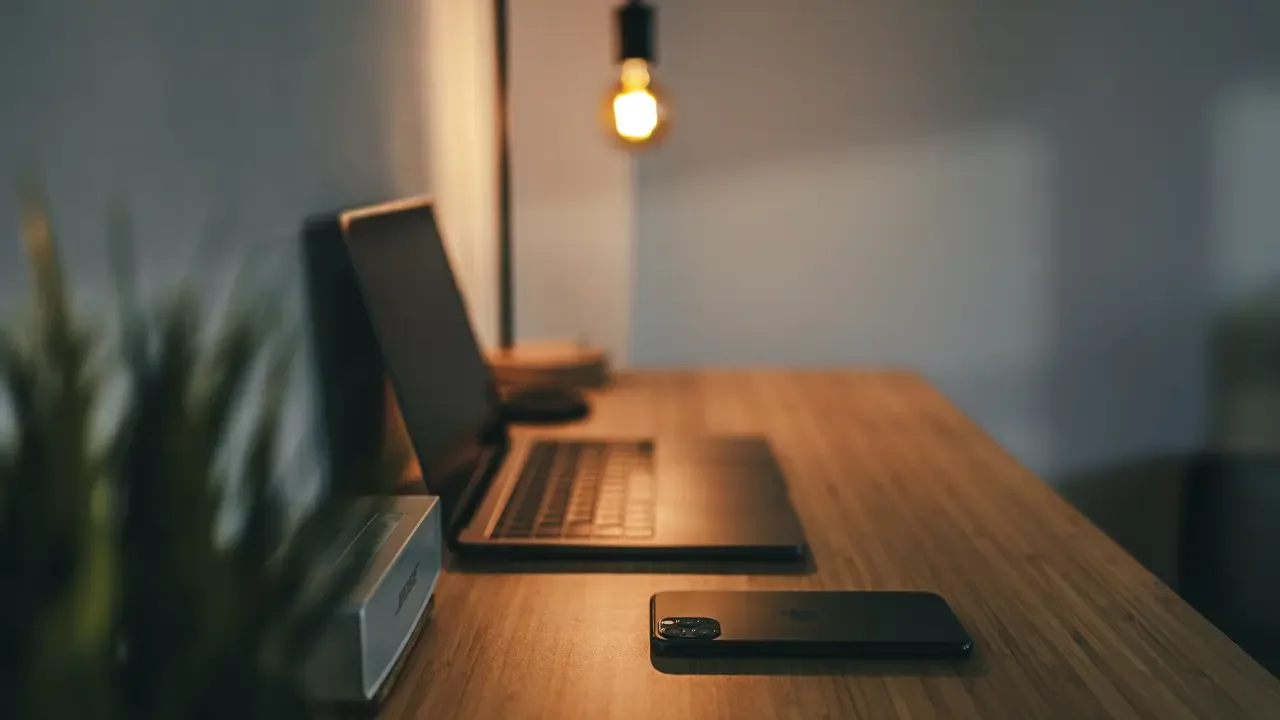
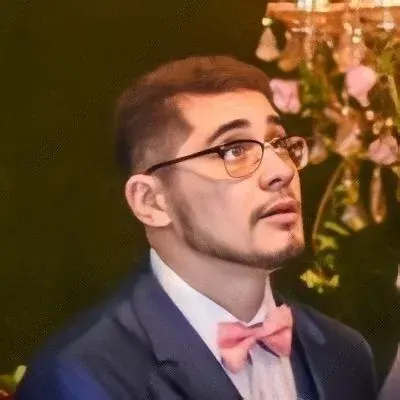
š Title: Bool in C: Unraveling the Mystery
š· Image: Illustration of a C code snippet with a question mark
Are you puzzled by the presence of bool
in the Linux kernel code? You might be wondering, isn't bool
a C++ type? š¤ Don't worry; you're not alone in this confusion. In this article, we'll dive into the world of bool
and demystify whether it's a native C type, a standard C extension, or a GCC extension. Let's go! šØāš»š¬
Understanding the Bool Conundrum
Yes, it's true that bool
is not a built-in type in standard C, like int
or float
. However, many modern C compilers, including GCC, provide support for bool
as an extension. This means you can use bool
in your C code without any issues as long as you're using a compatible compiler.
The Standard Bool Alternative
If you're working on a codebase that doesn't have support for bool
as an extension or you want your code to be more portable across different compilers, there's a standard alternative available. The <stdbool.h>
header, introduced in the ISO C99 standard, defines three new types: bool
, true
, and false
. By including this header, you can use these types just like you would in C++.
Here's an example of how you can use the standard bool values:
#include <stdbool.h>
#include <stdio.h>
int main() {
bool myBool = true;
if (myBool == true)
printf("It's true! š\n");
else
printf("False alarm! š±\n");
return 0;
}
By leveraging <stdbool.h>
, you ensure your code remains valid across various C compilers and versions.
Working with GCC and Bool
GCC, being one of the most widely used C compilers, provides an extension that allows you to use bool
directly without including any headers or external libraries. It treats bool
as an alias for int
, where false
is represented by 0, and true
is represented by any non-zero value. This means you can write code like this:
#include <stdio.h>
int main() {
bool myBool = 42;
if (myBool)
printf("It's true! š\n");
else
printf("False alarm! š±\n");
return 0;
}
In this code, myBool
is assigned the value 42, which is a non-zero value. Therefore, the condition if (myBool)
evaluates to true, and the corresponding message is printed.
Keep in mind that this GCC extension may not be available in all C compilers, so relying on it can limit the portability of your code. If you're working on a project where cross-platform compatibility is crucial, it's best to utilize the <stdbool.h>
approach we discussed earlier.
š¢ Share Your Thoughts!
Now that you have a clearer understanding of the bool
conundrum in C, we want to hear from you! Have you encountered any challenges or surprises while working with bool
in your projects? Share your experiences and insights in the comments below. Let's keep the conversation going! š¬āØ
In conclusion, while bool
is not a standard built-in type in C, it can be used with the help of extensions like GCC or the standard <stdbool.h>
header. Choose the approach that suits your project's requirements and ensure better code portability. Happy coding! šš»