How to split a string literal across multiple lines in C / Objective-C?
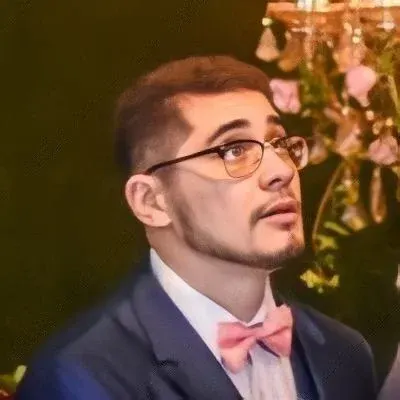
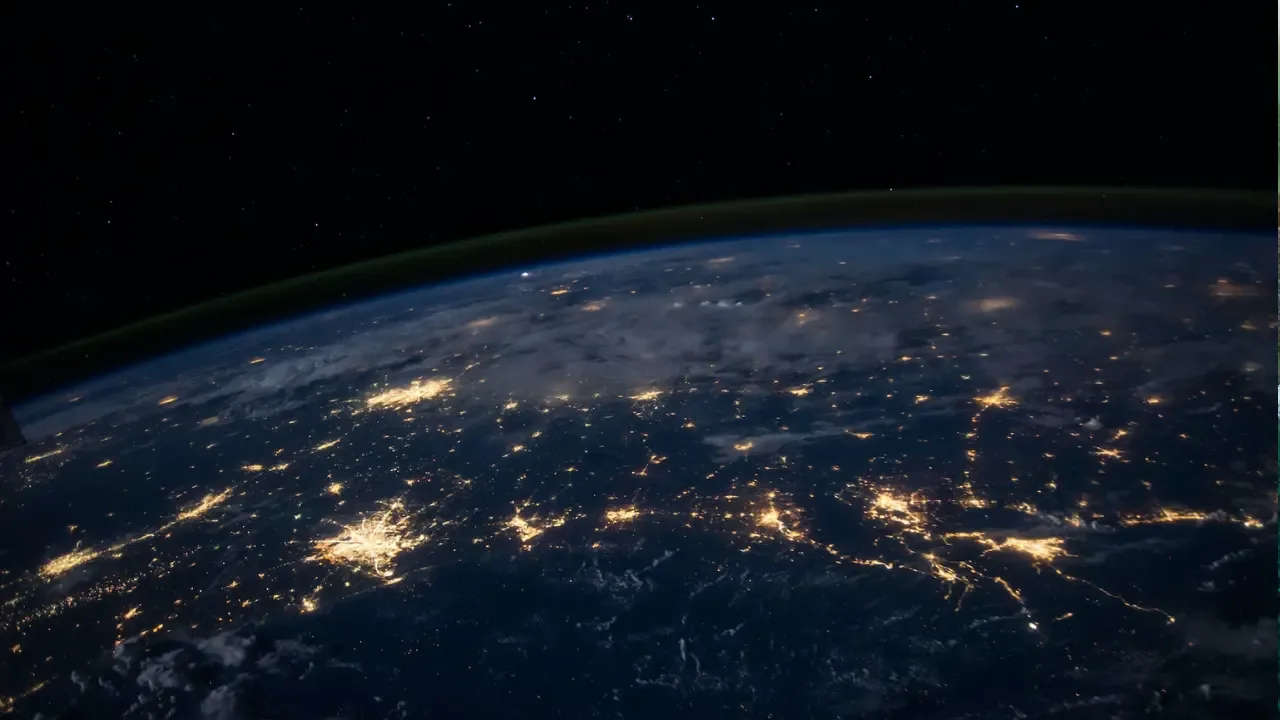
How to Split a String Literal Across Multiple Lines in C / Objective-C?
If you've ever encountered the need to write a long string literal in C or Objective-C, you might have found yourself struggling to keep it readable and maintainable. Long strings can be cumbersome to work with, especially when they need to fit within the constraints of a single line of code. Thankfully, there is a simple solution: splitting string literals across multiple lines. In this blog post, we'll explore common issues with splitting string literals and provide easy solutions to make your code more readable.
The Problem
Let's consider the following example where we have a lengthy SQL query:
const char *sql_query = "SELECT statuses.word_id FROM lang1_words, statuses WHERE statuses.word_id = lang1_words.word_id ORDER BY lang1_words.word ASC";
This one-line code snippet might be difficult to read and understand, especially for larger queries.
The Solution
To make the query more readable, we can split it across multiple lines. However, doing so without the appropriate approach can lead to errors. Let's see how to tackle this issue:
const char *sql_query = "SELECT word_id \
FROM table1, table2 \
WHERE table2.word_id = table1.word_id \
ORDER BY table1.word ASC";
By placing a backslash \
at the end of each line, we indicate that the string continues on the next line. This tells the compiler to treat the entire block of code as a single string, even though it is physically split across multiple lines.
Common Issues
When splitting a string literal across multiple lines, there are a few common issues to be aware of:
1. Whitespace Matters
Be mindful of the leading whitespace before the backslash \
at the end of each line. It is crucial to ensure that there is no additional space after the backslash, as it can lead to compilation errors.
2. String Concatenation
In C and Objective-C, adjacent string literals are automatically concatenated. However, when splitting a string across multiple lines, you need to account for this to avoid syntax errors. For example:
const char *str = "Hello" // Error: missing terminating " character
"World";
To resolve this issue, you can add a space or a newline character at the end of the line:
const char *str = "Hello "
"World";
3. Escape Sequences
If your string contains escape sequences like \n
(new line) or \t
(tab), ensure they are handled correctly across the split lines. For example:
const char *str = "Hello \
\nWorld";
Conclusion
Splitting a string literal across multiple lines can greatly improve the readability and maintainability of your code. By using backslashes \
to indicate string continuation, you can break long, unwieldy lines into smaller, more manageable chunks. Just remember to be mindful of whitespace, string concatenation, and escape sequences to avoid any issues.
Now that you have learned how to split string literals in C and Objective-C, give it a try in your own code and see how it improves readability. 🚀
Feel free to share your experience in the comments below or reach out on our social media channels. Happy coding! 😄💻
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
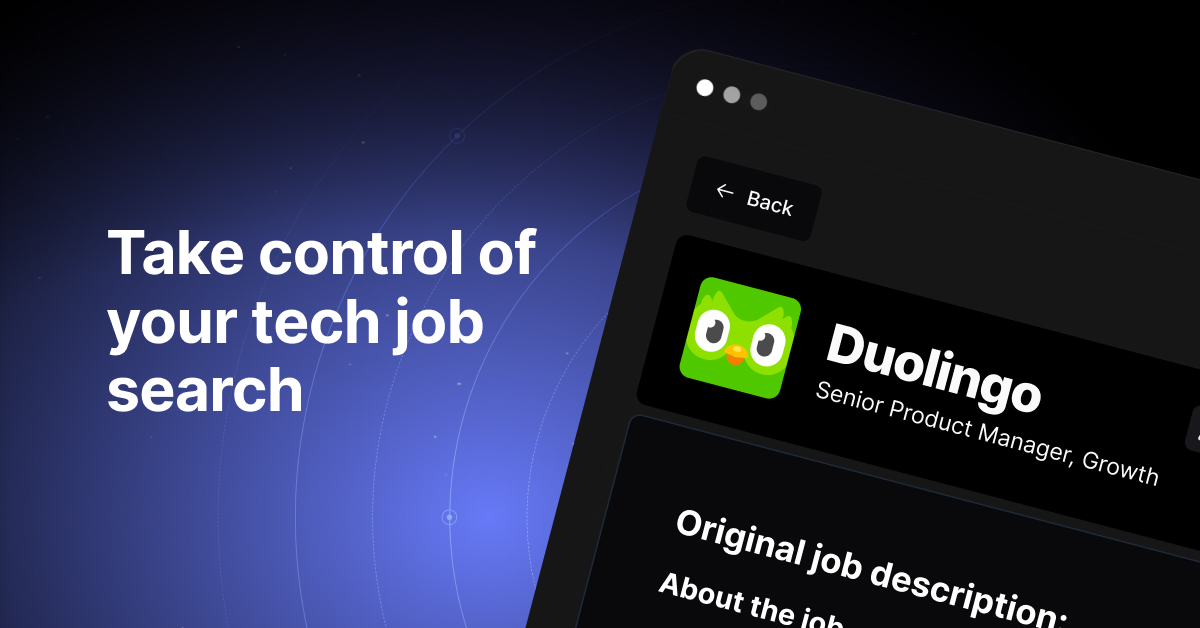