How to printf "unsigned long" in C?
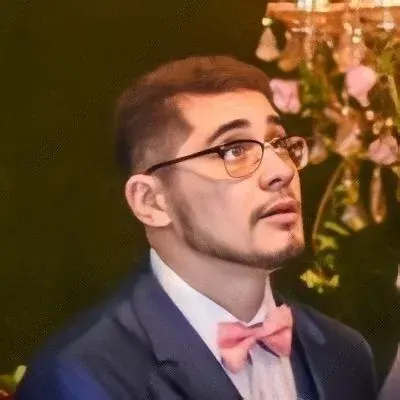
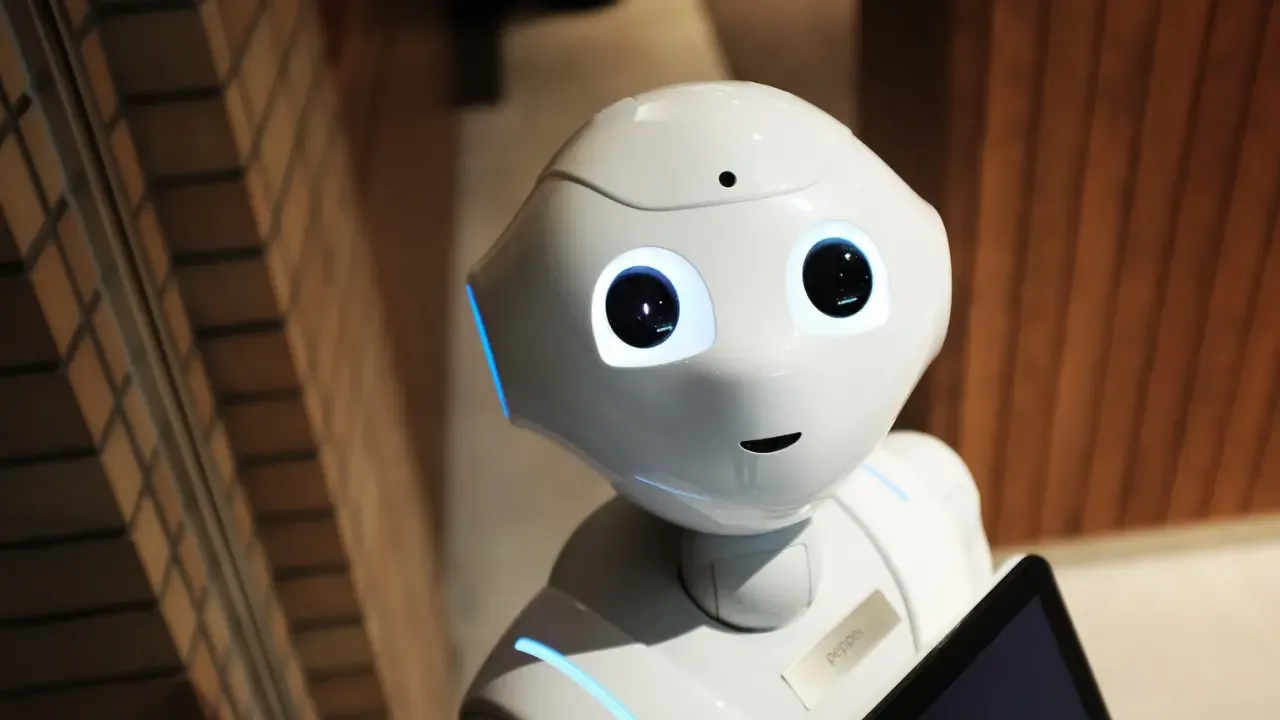
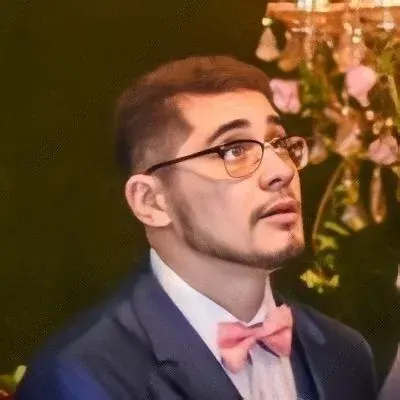
How to printf "unsigned long" in C? 🖨️
Have you ever found yourself scratching your head trying to figure out how to properly print an "unsigned long" datatype in C? Don't worry, you're not alone! Many developers face this common issue, but fear not, we're here to help you out. 💪
The Problem 😕
One of our readers recently asked us how to correctly print an "unsigned long" value using the printf
function in C. They mentioned trying various format specifiers like %lu
, %du
, %ud
, %ll
, %ld
, and %dl
, but none of them yielded the expected output. Instead, all they got was some strange negative number like -123123123
, instead of their actual "unsigned long" value.
The Solution 🎉
The issue lies in using incorrect format specifiers when using printf
to print an "unsigned long" value. To correctly print an "unsigned long" in C, you need to use the %lu
format specifier. Let's break it down:
unsigned long unsigned_foo = 123456789;
printf("%lu\n", unsigned_foo);
By using %lu
, you are telling printf
that you want to print an "unsigned long" value. This tells printf
to interpret the value as an unsigned integer and display the correct output.
Example Usage 🚀
Let's take a look at a complete example to illustrate the correct usage of the printf
function for an "unsigned long" value:
#include <stdio.h>
int main() {
unsigned long unsigned_foo = 123456789;
printf("The value of unsigned_foo is: %lu\n", unsigned_foo);
return 0;
}
In this example, we declare an "unsigned long" variable unsigned_foo
and assign it the value 123456789
. We then use printf
to display the value of unsigned_foo
by using the %lu
format specifier.
Conclusion 🎯
Printing an "unsigned long" value in C might seem tricky at first, but with the correct format specifier, it becomes a breeze. Remember to use %lu
when using printf
to print an "unsigned long" value.
If you have any more questions or face any other coding challenges, feel free to reach out to us. We're always here to help you become a better developer! 💻🌟
Now that you know the secret to printing "unsigned long" values, go ahead and give it a try in your own code. Happy coding! 💡🚀