How to prevent SIGPIPEs (or handle them properly)
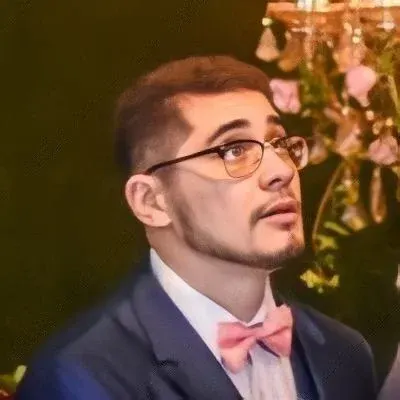
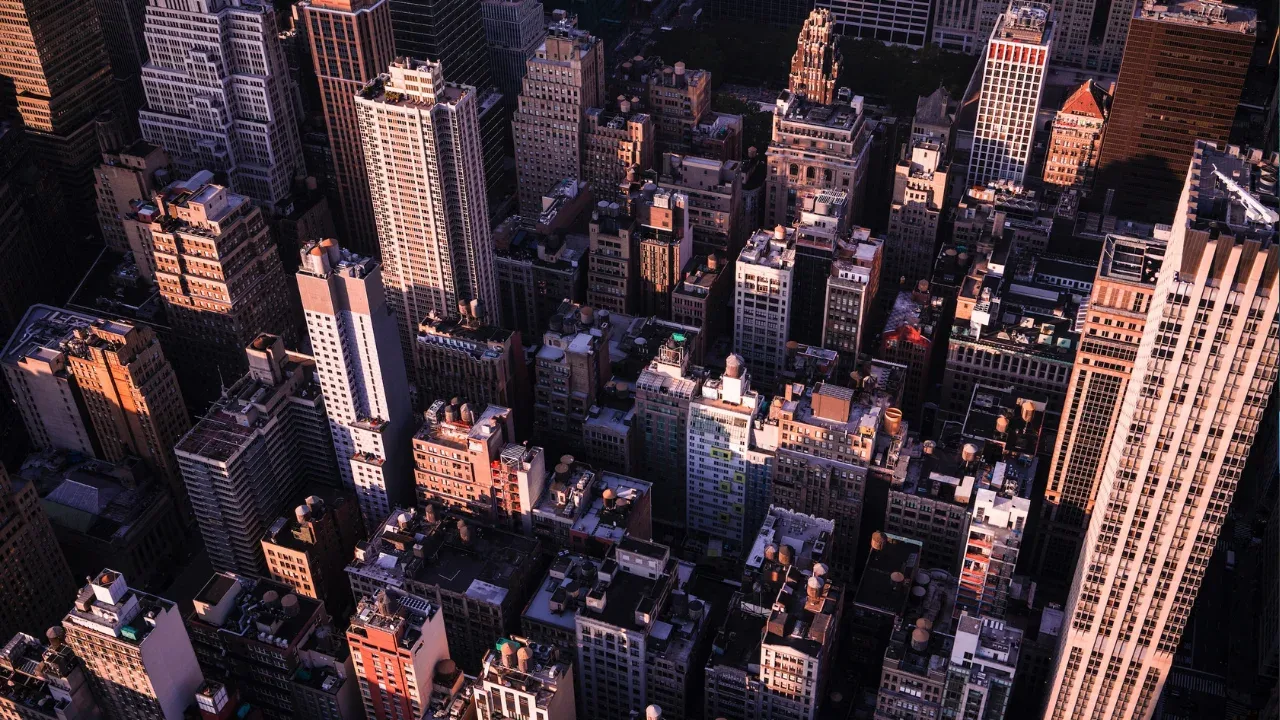
š Title: Preventing SIGPIPEs: Keeping Your Server Crash-Free
š„ Have you ever encountered the dreaded SIGPIPE error that crashes your server program? We know the struggle! In this blog post, we'll dive into the common issues surrounding SIGPIPEs and provide easy solutions to prevent them from derailing your server. But that's not all! We'll also answer the burning question: should you catch the SIGPIPE or prevent it altogether? Let's get started! šŖ
š¤ Understanding the SIGPIPE Problem
Imagine this scenario: you have a small server program that accepts connections on either a TCP or local UNIX socket. It reads a simple command, processes it, and sends a reply accordingly. However, the trouble arises when a client, for whatever reason, exits early before receiving the reply. Writing to that socket in such cases triggers a SIGPIPE, causing your server to come crashing down.
šØ We can't let that happen! But fear not, there's a solution - or rather, multiple possibilities.
š” Preventing SIGPIPE: Best Practices
1. Checking If the Other Side Is Still Reading
One common approach is to check if the other side of the socket is still actively reading before writing to it. Many developers instinctively turn to the select()
function for this purpose. However, be warned that the select()
function may not be suitable in all cases, as it always reports the socket as writable. So, what can we do instead?
š Solution: Use the SO_ERROR
Socket Option
By using the SO_ERROR
socket option, you can check the error status of the socket and determine if the other end is still actively reading. Here's an example:
int error;
socklen_t len = sizeof(error);
getsockopt(socket_fd, SOL_SOCKET, SO_ERROR, &error, &len);
if (error == 0) {
// The other side is still reading, safe to write
write(socket_fd, data, data_length);
} else {
// Handle the error gracefully
// Maybe close the socket or take appropriate action
}
š” Pro Tip: Wrap the socket error-checking code in a function for easy reuse across your server program! š
2. Catching and Ignoring the SIGPIPE Signal
If the previous solution seems a bit complex or doesn't fit your specific use case, you can handle SIGPIPE directly by catching and ignoring the signal.
š”ļø Solution: Catch and Ignore SIGPIPE
When a SIGPIPE signal occurs, you can gracefully handle it within your server program. By ignoring the signal, you prevent it from crashing your server. Here's how you can achieve this in C using sigaction()
:
#include <signal.h>
void ignore_sigpipe() {
struct sigaction sa;
sa.sa_handler = SIG_IGN;
sigaction(SIGPIPE, &sa, NULL);
}
š The choice is yours! Each solution was handcrafted with care, aiming to keep your server crash-free. Experiment and find the approach that best fits your requirements.
š£ The Power Moves to Take Action!
Now that you're armed with the knowledge to prevent SIGPIPEs and keep your server running smoothly, we want to hear from you! Share your thoughts, experiences, and any other novel solutions you've discovered. Let's unite as a community and keep our servers crash-free like true tech warriors! š„š
š Conclusion
ā Don't let SIGPIPEs ruin your day! By implementing the best practices discussed in this blog post, you can prevent crashes and ensure the stability of your server program. Whether you choose to check if the other side is still reading or catch and ignore the SIGPIPE signal, the goal is the same: server resilience. So go forth, implement these solutions, and join the battle against SIGPIPEs! šŖš
Remember, we're all in this together, and sharing is caring. Spread the word to fellow developers facing SIGPIPE issues, and let's empower each other to build robust server programs. Happy coding! š
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
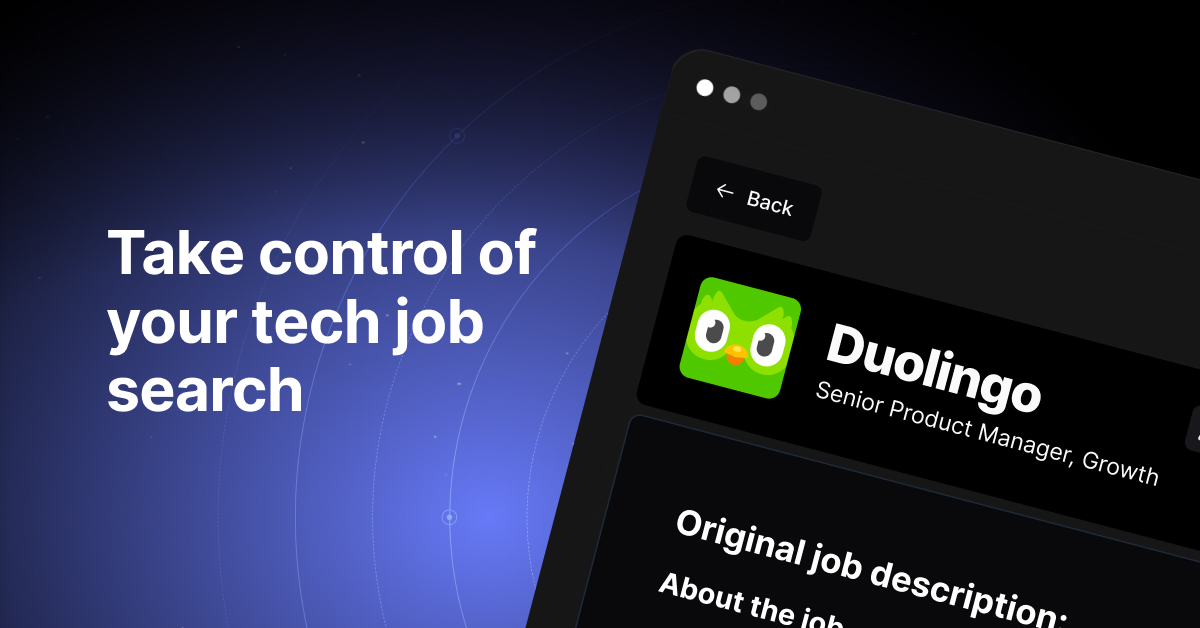