How to portably print a int64_t type in C
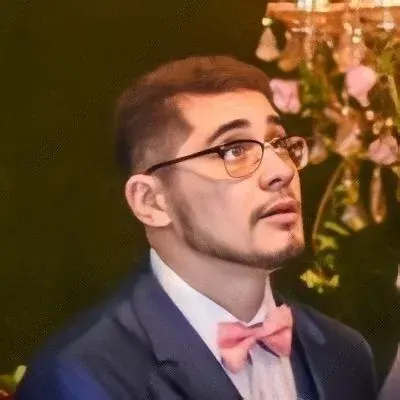
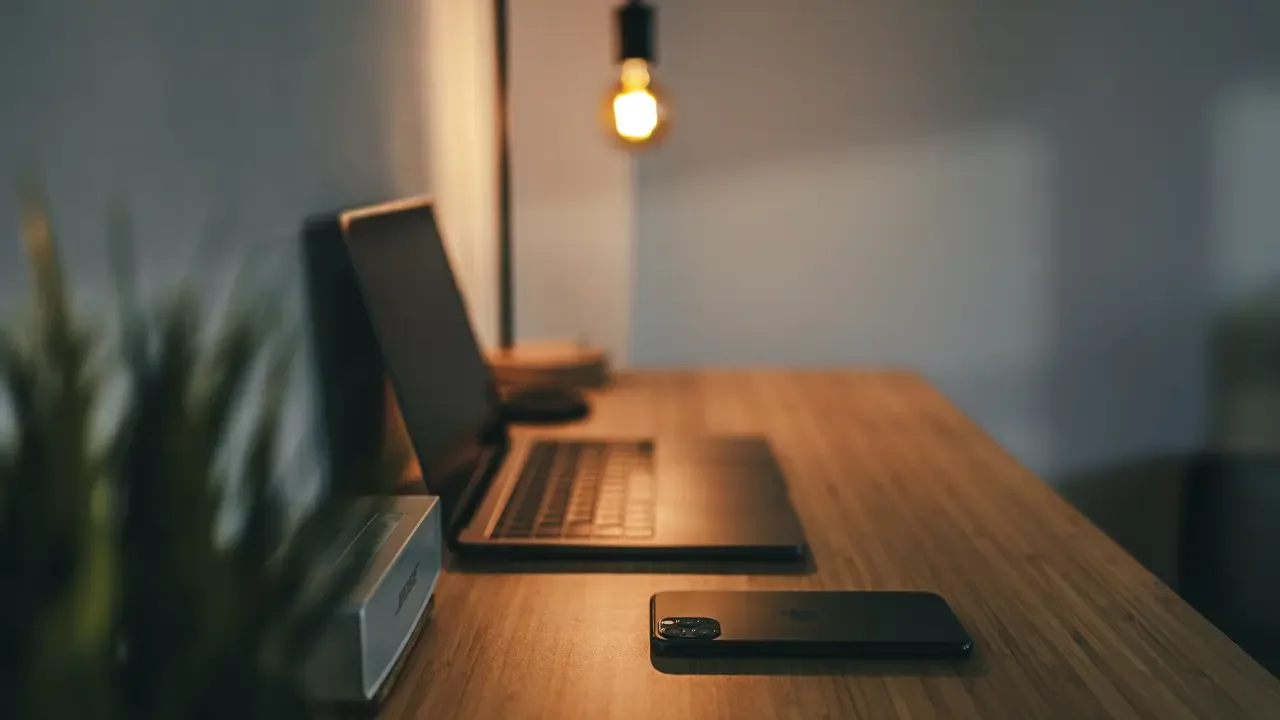
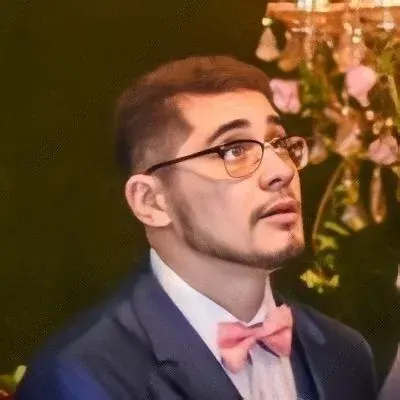
How to Portably Print an int64_t Type in C π
Are you working with the C99 standard and dealing with the int64_t type? Fantastic! But wait, are you struggling with printing these types portably without compiler warnings? Don't worry, we've got you covered! π
The Problem π€
Let's take a look at the code snippet you posted:
#include <stdio.h>
#include <stdint.h>
int64_t my_int = 999999999999999999;
printf("This is my_int: %I64d\n", my_int);
You mentioned that you receive a compilation warning:
warning: format β%I64dβ expects type βintβ, but argument 2 has type βint64_tβ
Sounds like a typical problem! The %I64d
format specifier is specific to certain compilers and platforms (like Windows), but it's not portable across different systems. π
Easy Solutions π‘
Here are a few simple solutions to help you print your int64_t
variable without any hassle:
Solution 1: "PRI" Macros from <inttypes.h>
πͺ
C99 introduced the <inttypes.h>
header, which defines format macros for different integer types. These macros make it easier to print integer types in a portable way. Let's modify your code:
#include <stdio.h>
#include <stdint.h>
#include <inttypes.h>
int64_t my_int = 999999999999999999;
printf("This is my_int: %" PRId64 "\n", my_int);
No more warning! π Using the PRId64
macro ensures that the correct format specifier is used for your int64_t
variable, regardless of the platform and compiler you're using.
Solution 2: Casting to long long
π
If you don't have access to the <inttypes.h>
header or prefer a simpler solution, you can cast your int64_t
variable to long long
and use the %lld
format specifier. Here's what it looks like:
#include <stdio.h>
#include <stdint.h>
int64_t my_int = 999999999999999999;
printf("This is my_int: %lld\n", (long long) my_int);
This workaround helps you avoid the compiler warning and ensures consistency across different systems.
π You Did It! π
Now you know how to print an int64_t
type in C without encountering those pesky compiler warnings! Give yourself a pat on the back! π
Your Turn! π
Did these solutions work for you? Have any other ideas or questions? Let us know in the comments section below! We're here to help you out. π