How to initialize a struct in accordance with C programming language standards
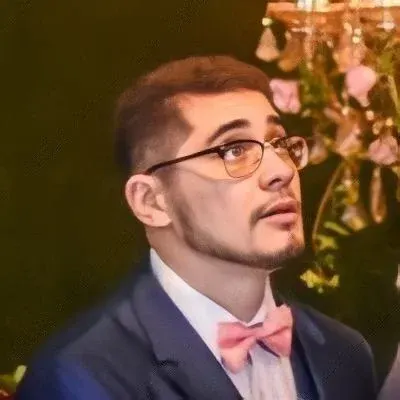
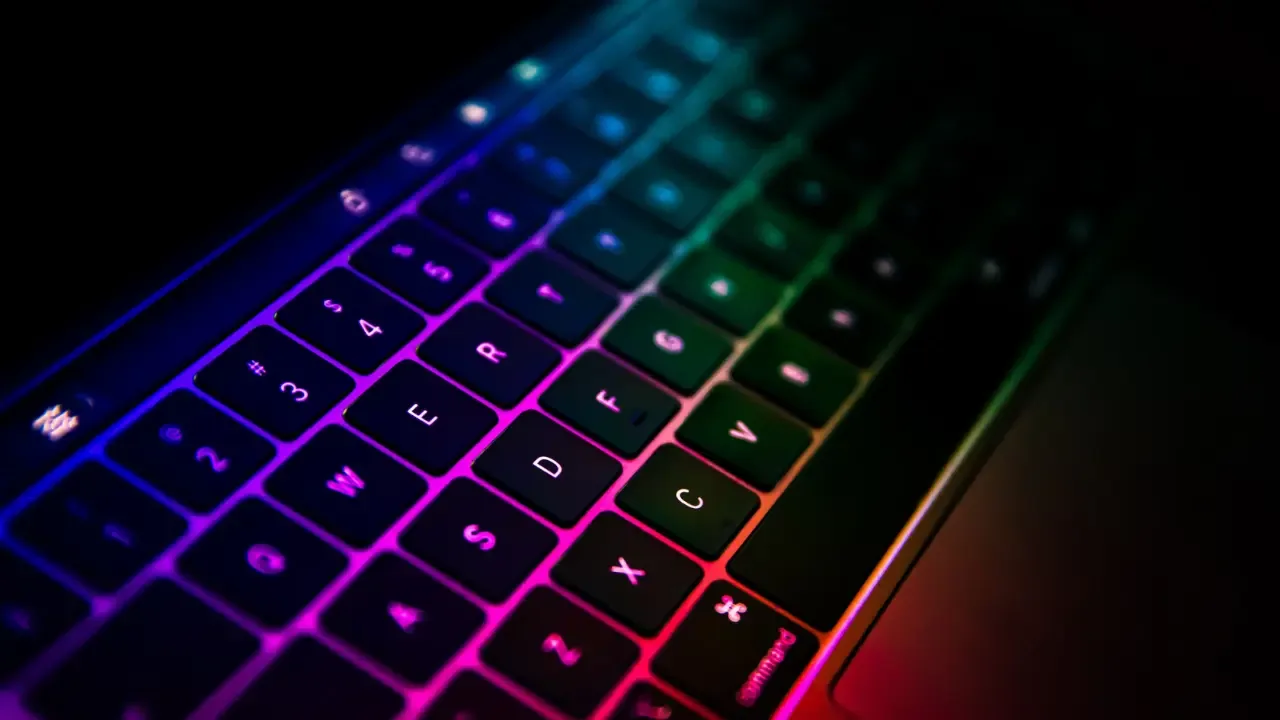
How to Initialize a Struct in C Programming 🏗️
Are you a C programmer who's struggling with initializing a struct according to C programming language standards? You've come to the right place! In this guide, we'll address common issues and provide easy solutions to help you properly initialize a struct. Let's dive in! 💪
The Problem 😕
Let's take a look at the code snippet you provided:
typedef struct MY_TYPE {
bool flag;
short int value;
double stuff;
} MY_TYPE;
void function(void) {
MY_TYPE a;
...
a = { true, 15, 0.123 };
}
You're trying to declare and initialize a local variable of MY_TYPE
within the function function()
. However, you're unsure if this is the correct way to do it according to C programming language standards. Let's find out together! 🕵️♂️
The Solution 💡
To initialize a struct in C, you have a few options depending on the programming language standard you're using. Let's explore the possibilities:
C89/C90 Standard 🆕
If you're programming in C89/C90 standard, the initialization syntax you used (a = { true, 15, 0.123 }
) is invalid. The C89/C90 standard only allows initializers in a declaration. So, you need to modify your code like this:
void function(void) {
MY_TYPE a = { true, 15, 0.123 };
// Rest of your code...
}
This way, you declare a
and initialize it in a single line, adhering to the C89/C90 standard. Easy, right? 😊
C99 Standard 🆗
If you're working with the C99 standard or a newer version, you can use the compound literal initialization syntax you've mentioned (a = { true, 15, 0.123 }
). It is a valid syntax in these newer versions, and you're good to go with your code as-is. Yay! 🎉
Mixing Standards ⚖️
If you're working on a project that mixes different C standards, it's important to consider the lowest common denominator. In your case, the solution for initializing a struct that works across all C standards would be:
void function(void) {
MY_TYPE a = { true, 15, 0.123 };
// Rest of your code...
}
By following this approach, your code will be compatible with both C89/C90 and C99 standards.
The Call-to-Action 📢
Congratulations! You've successfully learned how to initialize a struct in accordance with C programming language standards. Now, it's time to put your newly acquired knowledge into practice and level up your C programming skills. 🚀
If you found this guide helpful, share it with your fellow C programmers and spread the knowledge! Don't forget to leave a comment below if you have any further questions or want to share your own experiences. Happy coding! 👩💻👨💻
References 📚
Disclaimer: The information provided in this guide is based on commonly accepted practices and standards at the time of writing. Always refer to the official documentation or consult experienced developers for the most up-to-date information.
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
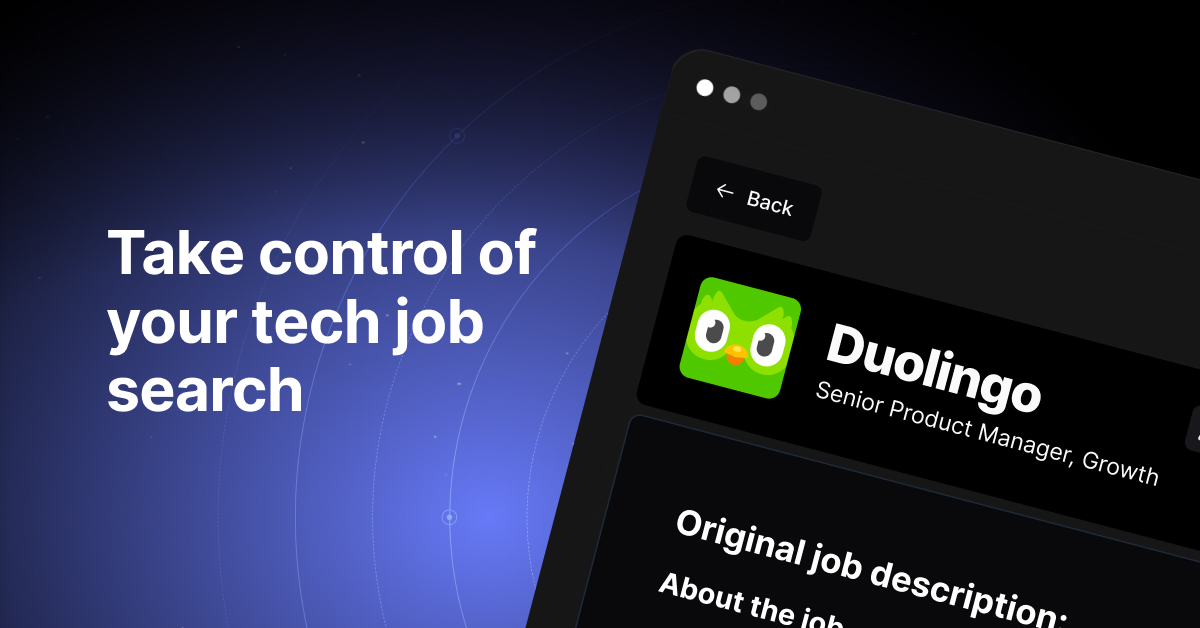